Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial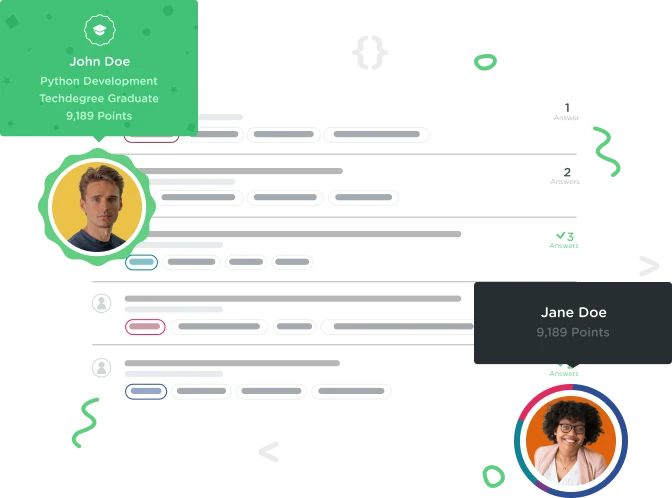

Régis Martin
2,352 PointsHi, could you please tell me what's wrong with this answer
function arrayCounter (a) {
if (typeof a === 'array') {
return a.length;
}
else {
return 0;
};
2 Answers
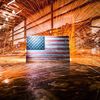
Andrew Shook
31,709 PointsI have answered this one a few times. Take a look here for an explanation of the problem.
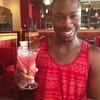
Matthew Reed
Courses Plus Student 17,986 Pointstypeof a
returns 'object'
so you have to use Array.isArray(a)
. You can check out the article for more info https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/isArray
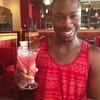
Matthew Reed
Courses Plus Student 17,986 Pointsreplace the whole statement typeof a === 'array'
with Array.isArray(a)
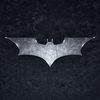
Logan R
22,989 Pointsfunction arrayCounter (a) {
if (Array.isArray(a)) {
return a.length;
} else {
return 0;
};
Tried it and that doesn't work.
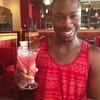
Matthew Reed
Courses Plus Student 17,986 PointsActually I guess you have to check if it's an object like Andrew Shook said in the link he posted

Jason Anello
Courses Plus Student 94,610 PointsYou're missing a closing curly brace which causes a syntax error. If you add it in then that code will pass the challenge.
The isArray
method would be the most foolproof way to check for an array that I know of. However, you don't learn it in the course and you can't really be expected to know it for this challenge.
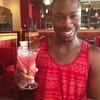
Matthew Reed
Courses Plus Student 17,986 PointsJason Anello I thought that was true about isArray()
. Nice to see I haven't gone crazy :/
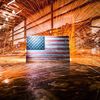
Andrew Shook
31,709 Pointsthe isArray method is the best choice in the real world. However, the JS course doesn't go over isArray(), but instead explains typeof. That is why I have, on several occasions, to answer the question using typeof. It isn't the best way to check for an array, but it does at least stay in sync with that is being taught.

Jason Anello
Courses Plus Student 94,610 PointsAndrew,
I agree with you that you should give solutions based on what is taught so far but I think it's ok to post better solutions so we can all learn more provided you've also shown what the course is based on.
I've looked over your solution but was it taught up to this point that the type of an array is an "object"? You would need to know that in order to come up with your solution and your solution doesn't match up with the challenge instructions.
I think it would be better to explicitly check the type for "string", "number", or "undefined" and return 0 in any of those cases. Otherwise, assume it's an array and return it's length. This is what the challenge instructions are leading you to do.
Something like this:
function arrayCounter(array) {
if (typeof array == 'undefined') {
return 0;
}
if (typeof array == 'string') {
return 0;
}
if (typeof array == 'number') {
return 0;
}
//Assume it's an array
return array.length;
}
It can be improved a little with the logical OR operator, ||
, but I don't think that was taught either up to this point.
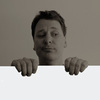
Sean T. Unwin
28,690 PointsI wanted to mention that Array.isArray()
is not compatible with <=IE8. A more legacy proof solution would be:
function arrayCounter(arr) {
if(arr instanceof Array) {
return arr.length;
} else { return 0;}
}

Aaron Graham
18,033 PointsSean T. Unwin - You can also just polyfill the Array.isArray() function:
if (!Array.isArray) {
Array.isArray = function(arg) {
return Object.prototype.toString.call(arg) === '[object Array]';
};
}
Straight from MDN
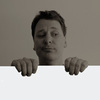
Sean T. Unwin
28,690 PointsTrue, Aaron, but then that`s more code. Why do I want to type more code? ;-D

Aaron Graham
18,033 PointsSean T. Unwin - It is my understanding that Array.isArray() is the suggested method for checking if a variable is an array in ECMA 5. There are some edge cases where other methods fail (i.e. moving arrays across multiple frames). Using polyfills allows you to code to modern browser standards and not lose backward compatibility. It's really a personal choice, I just wanted to mention that this method was available.
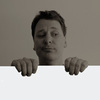
Sean T. Unwin
28,690 PointsThat's my understanding as well and I agree with you on the use of and reason for polyfills. I suppose I was a tad flippant previously. However, within a single DOM evnvironment I probably stand by my suggestion if legacy support is to be a consideration.
When there are multiple considerations it does appear the best answer, in terms of cross-browser/legacy support, support across multiple frames and the least amount of code (all 3 points), would be to simply use the polyfill:
if (Object.prototype.toString.call(obj) === '[object Array]') { }
Although, in terms of going forward and not looking back to older browsers the best answer is Array.isArray()
.
Thanks for the explanation, Aaron Graham. Good points all around and I had a good time researching it a little more deeply.
Btw, this is a detailed article on the subject - http://perfectionkills.com/instanceof-considered-harmful-or-how-to-write-a-robust-isarray/