Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial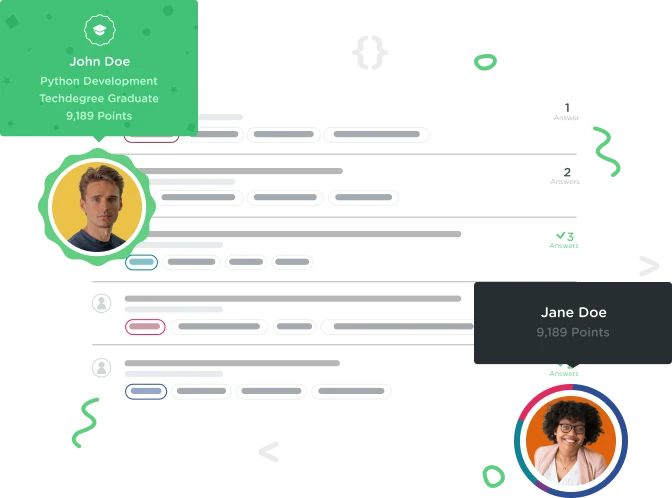
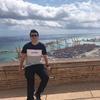
Tadjiev Codes
9,626 PointsHi, Dear Folks, Can you please help me to understand how to populate the form with the object or array that I have?
Hi, Dear Folks, Can you please help me to understand how to populate the form with the object or array that I have? For example, if the user enters 1 on the prompt. I want the first User that I have in my array to be filled in the form. I actually create the users in an object constructor way but then push inside an empty array of users. The link for the workspace snapshot: https://w.trhou.se/svt3or52gq When this button is pressed: • Prompt the user for the user index • Using that index, display the corresponding user details by writing them to the forms input fields ▪ If the index is out of range of the bounds of the array, alert the user that they entered an invalid index I would really appreciate your help)))
5 Answers
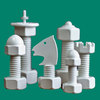
Steven Parker
231,271 PointsDisplaying the info is essentially just reversing what you do when you collect it:
const notify = prompt('Enter the user index number please!', 1);
const user = users[parseInt(notify) - 1];
document.getElementById('userFirst').value = user.firstName;
document.getElementById('userLast').value = user.lastName;
document.getElementById('userAge').value = user.age;
document.getElementById('userPhone').value = user.phone;
document.getElementById('userEmail').value = user.email;
Also, you don't need a template literal when only literal values are being interpolated:
So instead of: `${555}-${123}-${4567}
`
This would do: "555-123-4567"
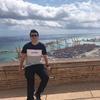
Tadjiev Codes
9,626 PointsDear Mr. Steven Steven Parker
Hope you're doing great)
Can you please help me to figure out this issue? At least a few hints like how to do When this button is pressed: • Prompt the user for the user index • Using that index, display the corresponding user details by writing them to the forms input fields ▪
I just need to understand how to relate to my objects in the last function showUserDetails() and populate the form input with that object user that I have lest's say if entered 1 in the prompt then user 1 fills the form or 2 3 4 5 and etcetera. And non existing index then alert that its wrong entry that I should be able to do by myself. Many thanks)))
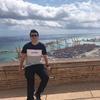
Tadjiev Codes
9,626 PointsThank you so much Mr.Steven) Hope you're doing great amidst this difficult time everywhere) So the code you wrote
const user = users[parseInt(notify) - 1];
basically, makes the users array equal to the variable user and converts the entered value of notify prompt into integer numbers and - 1 so that it will start from 1 but not 0? Earlier I did +1 in string interpolation so that users are displayed from 1 but not 0. Am I getting it right? Plus,
if (notify.value > users.length) {
alert('You entered an invalid index, please try again!');
}
I'm throwing this if statement inside the same function. Or would it be better for your code to add it inside an if statement and the rest in else condition? I can't understand how to make my alert work if its a non-existing array index? Thanks in advance
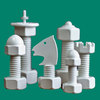
Steven Parker
231,271 PointsYou're right, the -1 here does the opposite of the +1 when displaying the index values.
And "notify" is not an element, but the string return from "prompt". So it doesn't have a "value" property.
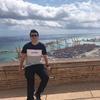
Tadjiev Codes
9,626 Pointsfunction showUserDetails() {
const notify = parseInt(prompt('Enter the user index number please!', 1));
const user = notify - 1;
if (notify > users.length) {
alert('You entered an invalid index, please try again!');
// First we're evaluating the false condition and then else for the true part kind of
} else {
document.getElementById('userFirst').value = users[user].firstName;
document.getElementById('userLast').value = users[user].lastName;
document.getElementById('userAge').value = users[user].age;
document.getElementById('userPhone').value = users[user].phone;
document.getElementById('userEmail').value = users[user].email;
}
}
I made it this way Mr.Steven. It does work but do you think there's any redundant code or that's okay and I added parseInt near the prompt otherwise it was not working like in ur code it was with const user. Thanks)
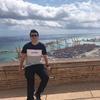
Tadjiev Codes
9,626 PointsAlso, I tried to add some form validation,
// Targeting the form elements input areas in order to perform some validation on it
const userFirst = document.getElementById('userFirst');
const userLast = document.getElementById('userLast');
const userAge = document.getElementById('userAge');
const userPhone = document.getElementById('userPhone');
const email = document.getElementById('userEmail');
// FUNCTIONS to validate the Form on change event
function validateEmail(emails) {
const re = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
return re.test(emails);
}
function numberValidation(numbers) {
const hasNumber = /\d/;
return hasNumber.test(numbers);
}
function validateForm() {
if (userFirst.value.length < 6) {
alert('Name is too too short. The Name must be 6+ characters.');
userFirst.focus();
} else if (userLast.value.length < 7) {
alert('Lastname is too too short. The Lastname must be 7+ characters at least.');
userLast.focus();
} else if (userAge.value.length < 2) {
alert('Age should contain two digits or numbers');
userAge.focus();
} else if (userPhone.value.length < 6) {
alert('Number is too short. Number must be 6+ characters.');
userPhone.focus();
} else if (!numberValidation(userPhone.value)) {
alert('Phone number does not include a number. It must include at least 1 number.');
} else if (!email.value) {
alert('Enter email.');
email.focus();
} else if (validateEmail !== email.value) {
// This is easier way to understand as !== strictly not equals although just throwing !before the function name is a bit lesser code to write
alert('Invalid email entered.');
email.focus();
}
}
And in HTML I added as an onchange="validateForm()" event. Is there any better practice? I heard usually its done with JS frameworks but I'm still learning vanilla JAvaScript.But generally adding as addEventListner on load would be better? Thanks
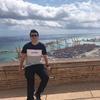
Tadjiev Codes
9,626 Pointsfunction showUserDetails() {
const notify = parseInt(prompt('Enter the user index number please!', 1));
const user = notify - 1;
if (notify > users.length + 1) {
alert('You entered an invalid index, please try again!');
// First we're evaluating the false condition and then else for the true part kind of
} else {
document.getElementById('userFirst').value = users[user].firstName;
document.getElementById('userLast').value = users[user].lastName;
document.getElementById('userAge').value = users[user].age;
document.getElementById('userPhone').value = users[user].phone;
document.getElementById('userEmail').value = users[user].email;
}
}
Anyways, just in cases I added +1 to my condition
if (notify > users.length + 1)
To make sure it adds + 1 to the length of the array
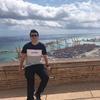
Tadjiev Codes
9,626 PointsMy Bad with + 1 it doesn't work for the fifth user. SO no + 1 is better