Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial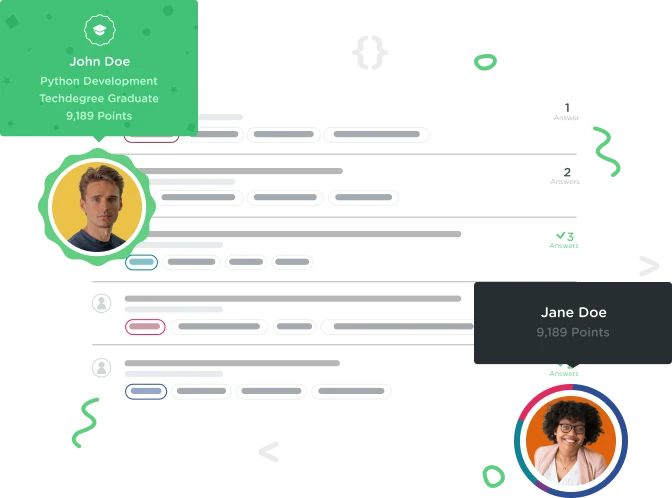

Michael Zaro
13,196 PointsHi, do I need to round the length?
Hi, do I need to round the length? The number should be either an int or some .5 value, so round is not needed?
Here is my code. What went wrong?
# The first half of the string, rounded with round(), should be lowercased.
# The second half should be uppercased.
# E.g. "Treehouse" should come back as "treeHOUSE"
def sillycase(string):
return string(:len(string)/2).lower() + string(len(string)/2:).upper()
3 Answers

boog690
8,987 PointsI see you're using parentheses, (), when you try to slice the strings. Slicing strings requires brackets, [], not parentheses.
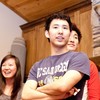
Sang Han
1,257 PointsYou might not even need round
, maybe you can always use the...
FlOOR DOUBLE DIVISION SYMBOL!!!!!!!! //
//
floor division operator returns the value rounded down to the nearest integer.
Examples
>>> # WOW
>>> 1 // 10
0
>>> # Doesn't meet you half way
>>> 5 // 10
0
>>> # Almost there!
>>> 9 // 10
0
>>> # Finally oh yes yes yes!
>>> 10 // 10
1
>>> 18 // 10
1
Right, so you get the picture
def sillycase(text):
half = len(text) // 2
return text[:half].lower() + text[half:].upper()
Your curly braces aren't balanced correctly, which could be an issue.
Also, string indexing/slicing should use []
brackets not ()
curly braces
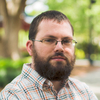
Kenneth Love
Treehouse Guest TeacherFloor division is not the same as rounding and won't produce the right answer.
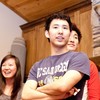
Sang Han
1,257 PointsI brought up floor division because it's assured to return a ints
that can be used directly for slicing the index. It also has a consistent api for Python 2 & 3 unlike True Division.
In the case of using round()
, it sometimes will casts floats to ints, but otherwise returns floats that are sometimes rounded incorrectly, which is less preferrable if a more pythonic solution exists. Ultimately type inspection albeit sometimes necessary, is always less preferable to invocation for writing code that is generic and maintainable.
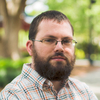
Kenneth Love
Treehouse Guest TeacherI appreciate the thought, but floor division, again, won't produce the right answer. round()
will, by default, always produce an int so that's not a problem, and it'll produce the right int in this situation, too, since we're not dealing with iffy fractions.
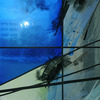
Philip Finch
5,262 PointsI would like to present my not-so-silly case for floor division rather than rounding for this function.
I made one function using round()
and one using floor division (//
), and a printout function that incrementally runs a string through it, adding one letter per iteration so you can see the progression of where the "halves" are split for many consecutive lengths of string. I added a separator to make it more obvious why floor division behaves more consistently here.
def sillycase_round(arg):
first_end = round(len(arg) / 2)
return arg[:first_end].lower() + " | " + arg[first_end:].upper()
def sillycase_floor(arg):
first_end = int(len(arg) // 2)
return arg[:first_end].lower() + " | " + arg[first_end:].upper()
def printout(func):
print("\nwith {}...".format(func.__name__))
count = 1
for x in range(0, len(my_string)):
incr_string = my_string[:count]
print(func(incr_string))
count += 1
my_string = 'teamtreehouse'
printout(sillycase_round)
printout(sillycase_floor)
The results:
treehouse:~/workspace$ python silly.py
with sillycase_round...
| T
t | E
te | A
te | AM
te | AMT
tea | MTR
team | TRE
team | TREE
team | TREEH
teamt | REEHO
teamtr | EEHOU
teamtr | EEHOUS
teamtr | EEHOUSE
with sillycase_floor...
| T
t | E
t | EA
te | AM
te | AMT
tea | MTR
tea | MTRE
team | TREE
team | TREEH
teamt | REEHO
teamt | REEHOU
teamtr | EEHOUS
teamtr | EEHOUSE
As you can see, as string length increases, with rounding, each half is increased in a staggered 1-3-1-3 pattern. With floor division, each half is increased in a very consistent 2-2-2-2 pattern.
In other words, with rounding, one half stays exactly the same length while the other half "grows" by three characters, then adds one, then another, then stays the same for three more iterations while the other half gains three characters in a row. (Characters are added in a left-left-right-right pattern.)
With floor div, one half gains a character, then the other, very consistently. (Characters are added in a left-right-left-right pattern.)
And there you have it! Sillycase 2.0. Yes, using floor div doesn't produce the "right answer" for this code challenge without a minor adjustment, but it seems "better".

Scott Dunstan
12,517 Pointsyou could put int(round(len(the_variable)/2))