Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial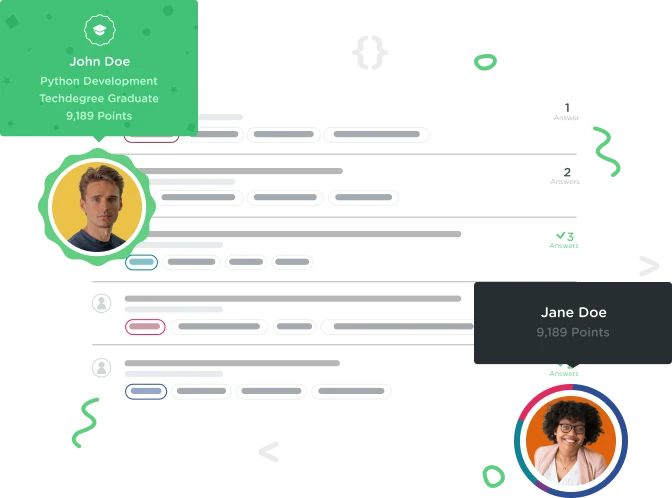

damien marez
6,085 PointsHi everyone, can you give me your opinion about my code ?
// Quizz array Part One
// Variables
var userAnswer;
var goodAnswer = 0;
var badAnswer = 0;
var goodAnswerArray = [];
var badAnswerArray = [];
// Questions and Answers for the Quizz
var questionsArray = [
['Who is the CEO of Apple ? ', 'tim cook'],
['Who is the first CEO of Microsoft ? ', 'bill gates'],
['Who is the CEO of Facebook ? ', 'mark zuckerberg'],
['Who is the second CEO of Microsoft ? ', 'steve ballmer'],
['Who is the first CEO of Apple ? ', 'steve jobs']
];
// Function which print bad and good answers
function displayMessage(good, bad){
document.write('<h1> Result of the Quizz </h1>');
document.write("<div id='good'><h3> Correct answer for these questions : " + goodAnswer + "</h3></div>");
document.write( '<ol>' + goodAnswerArray + '</ol>');
document.write("<div id='bad'><h3> Bad answer for these questions : " + badAnswer + "</h3></div>");
document.write( '<ol>' + badAnswerArray + '</ol>');
}
// Function that compare the answer of the user and the answer in the array
function compareArray() {
for (var i = 0; i < questionsArray.length; i += 1) {
userAnswer = prompt(questionsArray[i][0]);
if(userAnswer.toLowerCase() === questionsArray[i][1]){
goodAnswerArray += '<li>' + questionsArray[i][0] + '</li>';
goodAnswer += 1;
}else{
badAnswerArray += '<li>' + questionsArray[i][0] + '</li>';
badAnswer += 1;
}
}
displayMessage(goodAnswerArray, badAnswerArray);
}
// Call compareArray() function
compareArray();
I hope you will understand my english ^_^ Thanks in advance
2 Answers

Thomas Nilsen
14,957 PointsReally good code :)
I have a few suggestions though;
- Consider changing your questionsArray to an array of objects instead of an array of arrays. Like this:
var questionsArray = [
{question: 'Who is the CEO of Apple ? ', answer: 'tim cook'}
//rest of the questions here...
];
This is much more readable instead of having to remember which index is the question and which is the answer.
- Your compare array function has an if/else with two nearly identical code lines. Make a function instead.
- You count the goodAnswer and store in an array. When you have the array you can just call the length property which means you can get rid of both 'goodAnswer' and 'badAnswer'.
With that in mind, here is my take on your code.
// Quizz array Part One
// Variables
var userAnswer;
var goodAnswerArray = [];
var badAnswerArray = [];
// Questions and Answers for the Quizz
var questionsArray = [
{question: 'Who is the CEO of Apple ? ', answer: 'tim cook'},
{question: 'Who is the first CEO of Microsoft ? ', answer: 'bill gates'},
{question: 'Who is the CEO of Facebook ? ', answer: 'mark zuckerberg'},
{question: 'Who is the second CEO of Microsoft ? ', answer: 'steve ballmer'},
{question: 'Who is the first CEO of Apple ? ', answer: 'steve jobs'}
];
// Function which print bad and good answers
function displayMessage(good, bad){
document.write('<h1> Result of the Quizz </h1>');
document.write("<div id='good'><h3> Correct answer for these questions : " + goodAnswerArray.length + "</h3></div>");
document.write( '<ol>' + goodAnswerArray.join('') + '</ol>');
document.write("<div id='bad'><h3> Bad answer for these questions : " + badAnswerArray.length + "</h3></div>");
document.write( '<ol>' + badAnswerArray.join('') + '</ol>');
}
function buildArray(key, question) {
(key === 'correct') ? goodAnswerArray.push('<li>' + question + '</li>') :
badAnswerArray.push('<li>' + question + '</li>');
}
// Function that compare the answer of the user and the answer in the array
function compareArray() {
questionsArray.forEach(function(obj) {
userAnswer = prompt(obj.question);
(userAnswer.toLowerCase() === obj.answer) ? buildArray('correct', obj.question) : buildArray('wrong', obj.question);
});
displayMessage(goodAnswerArray, badAnswerArray);
}
// Call compareArray() function
compareArray();

damien marez
6,085 PointsThank you very much Thomas !
Your code is more readable and really well structured. Actually, I haven't seen the object arrays and I didn't know the forEach Method. But I can understand your code. I will do some research to better understand and improve my skill in JS.
Have a nice day ^_^