Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial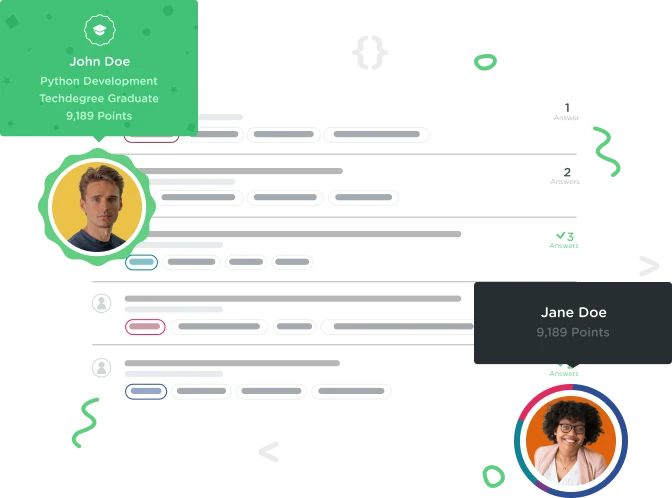

Richard Burdon
2,040 PointsHi Guys, How do I use conditional statements to return the highest value of two arguments within a function?
Some help would be much appreciated, I've tried a few ways but unable to work it out.
function max(lower, upper); {
var largest = upper > lower;
if (largest > 5)
return largest;
}
1 Answer
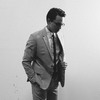
Matt Brock
28,330 PointsThe immediate issue breaking your code is the semicolon between the function's parameter list and opening bracket. If you remove that, the code runs without errors, but still doesn't achieve what you want. The function should take 2 integers and return whichever is bigger, so you could check if lower > upper
and return the appropriate input argument based on that conditional. Here's what I'd recommend:
function max (a, b) {
if (a > b) {
return a;
} else {
return b;
}
}
Or, using a tertiary operator (shorthand for a single conditional if
statement):
function max (a, b) {
return a > b ? a : b;
}
Or, use a super fancy ES6 arrow function:
var max = (a, b) => a > b ? a : b;