Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial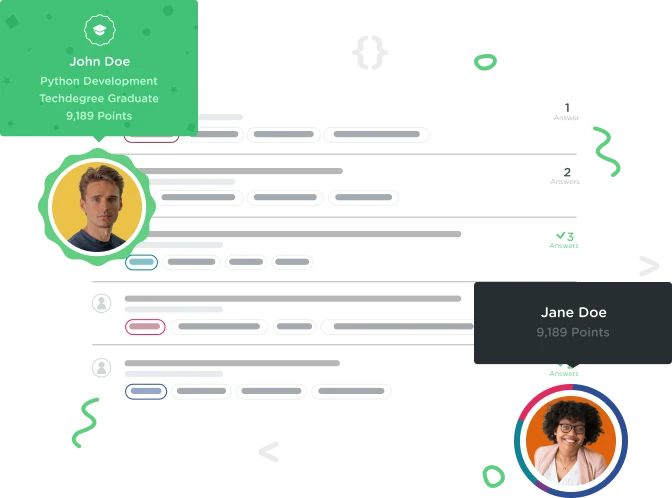

Kashish Singal
3,368 PointsHi guys, I am having trouble understanding what "this.name = name;" means. What does each word do?
What does "this" do and why are there two "name"s? Thanks.
6 Answers
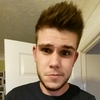
Adam Bowers
11,812 PointsHello Kashish!
I'll try to answer best I can for you.
The 'this' keyword is a bit of a JavaScript gotcha. It is covered in a course here in the JavaScript library, but to get you started the 'this' keyword is commonly used in Object in Javascript that is the prototype for a new Object.
You may be familiar with the format of object literals in JavaScript. Taking a dog for example we could make a dog object like below literally.
var dog = {
name: 'sally'
coat: 'smooth',
breed: 'dashhound',
bark: function(sound)
{
console.log(sound);
}
}
The above creates a dog object, it has three object properties. name, coat and breed. You can access these using the dot notation. For instance javascript console.log(dog.name);
The dog object also has one method (The name for a function inside an object) called bark: accessible like so: javascript console.log(dog.bark('Woof'));
Notice that when we created the dog object and gave it a method (function) called bark we provided it with a 'sound' parameter in the parenthesis, then inside the method (function) we supplied console.log() with the sound argument again, this means that when you declare dog.bark(); you'd include a string for the dog to make a noise, you could for instance use console.log(dog.bark('oink')); to the same affect.
But what if you wanted multiple dogs, all with similar properties and methods, but each needed to be different. That's where 'this' will come into play.
Rather than declaring an Object Literal inside a variable as above, what you do instead is create a constructor function (Factory, Prototype - they have many names).
function Dog(name, coat, breed, sound){
this.name = name;
this.coat = coat;
this.breed = breed;
this.bark = function(){
console.log(sound);
}
}
Notice above the first difference is that we declare the object with a function, as this is now our factory for creating other objects, based off this prototype.
To make it clear that your function is for the creation of objects and not part of your programs logic it's good practise to name your object with a Capital letter.
Then like before parameters are passed into this function, then notice that instead of providing key value pairs literally like before, we say 'THIS' which refers to the object and then we use the dot syntax to provide a parameter or method.
This is where your this.name = name; comes into play.
All that is being said is that THIS objects 'name' property will equal the value of whatever is passed into the function when the object is created.
I hope this helps.
Oh, and one more thing, just to illustrate a complete object like this and how we'd create it.
function Dog(name, coat, breed, sound){
this.name = name;
this.coat = coat;
this.breed = breed;
this.bark = function(){
console.log(sound);
}
}
var myDogOne = new Dog('Mr Wiggles','smooth', 'Dalmatian','YAP' );
console.log(myDogOne.name);
//The console logs 'Mr Wiggles'
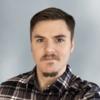
John Tasto
21,587 Pointsthis
is one of the weirdest parts of JavaScript. It gets set to whatever is before the dot when a function is called. How it works is probably best illustrated by example:
var obj = {
prop: "value",
reportProp: function () {
console.log(this.prop); // 'this' refers to obj
},
};
obj.reportProp(); // where 'this' gets set to obj
However, in a constructor function, this
gets set a little differently - it is set by the new
keyword, which creates a new empty object and sets this
to refer to that new object before running the constructor function:
function Contact(name, email) {
this.name = name; // 'this' refers to the object that is
this.email = email; // eventually assigned to 'contact'
}
var contact = new Contact('Andrew', 'andrew@teamtreehouse.com');
It gets weirder though if a function is passed to another object because its context is lost. this
is set based only on how the function gets called. Another example (using obj
from the first example):
var callFunc = function(func) {
func();
}
callFunc(obj.reportProp);
In this case, inside the callfunc
object, it only has a reference to some random unknown function (from its perspective). When it calls func()
, by default this
gets set to the global window
object (in browsers). Then when reportProp()
runs, this
no longer refers to obj
, but instead the global object. If there is a window.prop
value, it will log it, but if there isn't, this.prop
will be undefined.
There are several built in JavaScript functions that allow you to set this
explicitly, and they can be used when the context for this
has been lost:
function.apply(inst, [arg1, ... argN]);
function.call(inst, arg1, ... argN );
So in this case, we could rewrite callFunc
as follows:
var callFunc = function(func, context) {
func.call(context); // set 'this' to 'context', which in turn refers to 'obj'
}
callFunc(obj.reportProp, obj);
It can be really confusing, especially if you're coming from C++ or Java like me. If my explanation doesn't make sense, please let me know and I'll try to clarify.

Kashish Singal
3,368 PointsNo need your explanation works great! Thank you so much!

Rachelle Wood
15,362 Pointsthis is a pain-in-the-rear sometimes to understand. I found the Treehouse workshop on this to be helpful, but I admit I still go "huh?" from time-to-time.
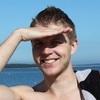
Kristjan Reinsberg
50,960 PointsConstructor function is just blueprint WITH NO DATA. You CANT GET any info from it... like usually..
You have to make new variable with new keyword: var detailsOfPerson = new Contact ("Martin", "koiv@gmail.com");
and now this variable is your classical object.. and you can use this as usually... as example we created that:
var detailsOfPerson = { this.name = "Martin", this.email = "koiv@gmail.com" }
Juan García
10,723 PointsExcellent thread, thank you!

nathan goel
7,410 Pointsthank you for excellent explanation on this subject, I was myself very confused after this lesson.
Kashish Singal
3,368 PointsKashish Singal
3,368 PointsThis makes so much sense now. It did not occur to me that when "this" was used, then it would be needed for the new instances of the object. Thank you so much!
Adam Bowers
11,812 PointsAdam Bowers
11,812 PointsNo problem Kashish :)
Kashish Singal
3,368 PointsKashish Singal
3,368 PointsHey Adam, you are very knowledgeable. Do you think you can help me with this question of mine.
https://teamtreehouse.com/forum/when-to-use-this-in-the-function-and-when-to-use-prototypes
Alina Antemir
10,185 PointsAlina Antemir
10,185 PointsHi Adam,
Your explantation is excellent! I was really confused at this point and now it all makes sense! Brilliant, not only you have knowledge but also talent to share it! Thank you so much for helping us!!!!