Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial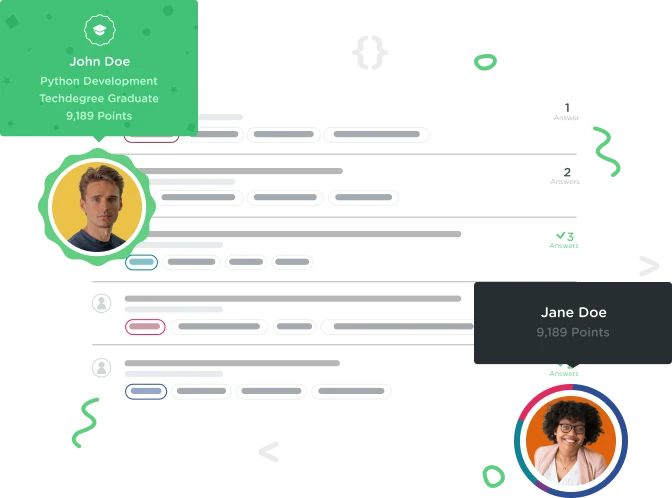

Thandolwethu Bhebhe
1,417 Pointshi guys, I have went through this test however I have questions on a few things that dont really make sense to me.
1) why is "author" being accepted in the Main class when i dont even have an instance of it. I just called the forum post constructor in the Main and passed it ("author", topic, description) how does Main.java know what author is, theres nothing that instantiates forumPost post class eg forumPost forum = new ForumPost()
2) how exactly is the args array getting filled, does it ONLY take info you pass into you default constructor, or will it carry on and save almost your entire program in the args array eg variables, printouts, ints that have been turned to strings etc
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum(String topic){
this.topic = topic;
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName = firstName;
this.lastName = lastName;
}
// TODO: add getters for firstName and lastName
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
public ForumPost(User author, String title, String description){
this.author = author;
this.title = title;
this.description = descriptioin;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(args[0],args[1]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "args array", "dont grasp this a 100%");
forum.addPost(post);
}
}
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Thandolwethu,
It might be easier to answer question 2 first since question 1 depends on it.
Question 2 - The args array is a way for you to pass arguments into your program from the command line.
An example usage:
java Main Thandolwethu Bhebhe
This will run the Main class and it will pass those 2 arguments into the Main method as the args array. This means that args[0]
is storing your first name and args[1]
is storing your last name.
Question 1 -
A User object is created with the following code:
User author = new User(args[0],args[1]);
This creates a User object with whatever first and last name was passed into the program at the command line and stores it in the variable author
. This user object can then be passed into the ForumPost constructor to create a ForumPost with that user as the author.
Louis Sankey
22,595 PointsLouis Sankey
22,595 Pointshmm.. I think the answer to your first question is that 'author' is not the author from the ForumPost class but its the name of the new User you created right above. User author = new User(args[0],args[1]); You could have named this anything. I'm not sure exactly about the main args array.