Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial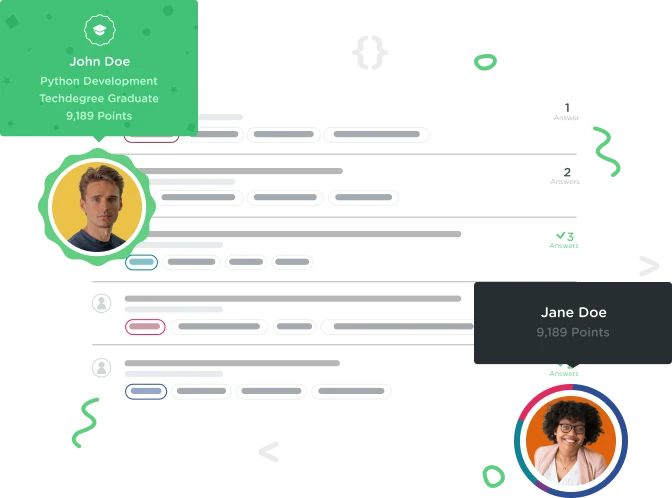

Travis Yeargans
2,673 PointsHi, i am stuck on 2/3 Refactoring into a Model
im not certain what i am doing wrong, but i can not compile. Thank You
@interface Quote : NSObject
@property (nonatomic, strong) NSArray *quotes;
- (NSString *) randomQuote;
- (NSArray *) quotes;
@end
#import "Quote.h"
@implementation Quote
- (NSArray *) quotes {
if(_quotes == nil){
NSArray * _quotes = [[NSArray alloc]initWithObjects:@"Helloo",@"Jella",@"Pudding",nil];
return _quotes;
}
}
- (NSString *) randomQuote {
return @"Some Quote";
}
@end
3 Answers

Nick Janes
5,487 PointsOkay, a few things to note.
1 On this line,
NSArray * _quotes = [[NSArray alloc]initWithObjects:@"Helloo",@"Jella",@"Pudding",nil];
You can remove the NSArray *_quotes, because you already defined it in your header file. _quotes is simply a reference to that variable you defined in your header. It should be:
_quotes = [[NSArray alloc]initWithObjects:@"Helloo",@"Jella",@"Pudding",nil];
2 When you made the:
- (NSArray *) quotes;
line in your header file, or .h, it was unnecessary. You could simply delete that out. Your header file, or .h, is pretty much saying, these methods and variables and properties can be accessed from other classes, while your implementation, or .m, is like your local classes, meaning only code within that file has access to those methods and variables and properties.
The point of this quotes method is just to simply give the _quotes array some initial values before its used, and the _quotes is locally initialized by that class.

Nick Janes
5,487 PointsHere's the final code that got me past that challenge, in case you wanted to reference it all.
Quote.h
@interface Quote : NSObject
// Add property here
@property (nonatomic, strong) NSArray *quotes;
- (NSString *) randomQuote;
@end
Quote.m
#import "Quote.h"
@implementation Quote
- (NSArray *) quotes {
if ( _quotes == nil ) {
_quotes = [[NSArray alloc] initWithObjects:@"It is certain",@"It is decidedly so",@"All signs say YES",
@"The stars are not aligned",
@"My reply is no",
@"It is doubtful",
@"Better not tell you now",
@"Concentrate and ask again",
@"Unable to answer now", nil];
}
return _quotes;
}
- (NSString *) randomQuote {
return @"Some Quote";
}
@end

Travis Yeargans
2,673 PointsI appreciate that information, im still having trouble wrapping my mind around the purpose of ivars vs @property declarations. More specifically, when i say self.property is self accessing a specific instance of the ivar or is it referring to the instance of the object it is being passed. I may be over thinking this but could you please explain.