Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial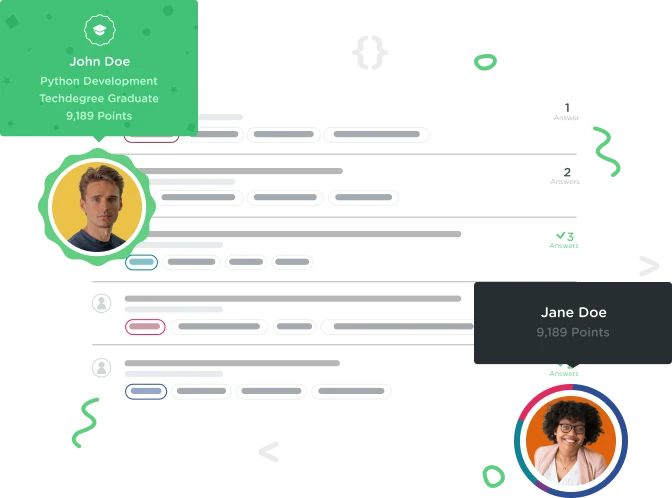
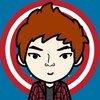
Craig Weston
Front End Web Development Techdegree Graduate 32,840 PointsHi, I'm stuck on this Challenge. To the button enum, add a method named toUIBarButtonItem that returns an instance of
This is the Challenge:
Challenge Task 2 of 2
To the button enum, add a method named toUIBarButtonItem that returns an instance of UIBarButton item configured properly.
In the buttons.swift file there is a basic implementation of UIBarButtonItem. You can create buttons with three different styles and titles.
Using the associated values as titles for the button, return a button with style UIBarButtonStyle.Done for the Done member of the Button enum. Similarly for the Edit member, return a UIBarButtonItem instance with the style set to UIBarButtonStyle.Plain.
In both cases you can pass nil for target and action. Once you have a method, call it on the value we created in the previous task and assign it to a constant named doneButton.
Here is what I have put:
enum Button { case Done(String) case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case .Done("Done"):
return UIBarButtonItem(title: "Done", style: UIBarButtonStyle.Done, target: nil, action: nil)
case .Edit("Edit"):
return UIBarButtonItem(title: "Edit", style: UIBarButtonStyle.Plain, target: nil, action: nil)
}
}
}
let doneButton = Button.Done
This is the error:
swift_lint.swift:35:9: error: switch must be exhaustive, consider adding a default clause } ^ swift_lint.swift:35:9: error: switch must be exhaustive, consider adding a default clause } ^
import Foundation
enum UIBarButtonStyle {
case Done
case Plain
case Bordered
}
class UIBarButtonItem {
var title: String?
let style: UIBarButtonStyle
var target: AnyObject?
var action: Selector
init(title: String?, style: UIBarButtonStyle, target: AnyObject?, action: Selector) {
self.title = title
self.style = style
self.target = target
self.action = action
}
}
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case .Done("Done"):
return UIBarButtonItem(title: "Done", style: UIBarButtonStyle.Done, target: nil, action: nil)
case .Edit("Edit"):
return UIBarButtonItem(title: "Edit", style: UIBarButtonStyle.Plain, target: nil, action: nil)
}
}
}
let doneButton = Button.Done
7 Answers
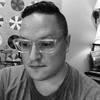
Joe Hill
15,063 PointsHi everyone. I had a real hard time with this one in the Swift 3.0 one as well. But here is the answer I got for it:
// Example of UIBarButtonItem instance
// let someButton = UIBarButtonItem(title: "A Title", style: .plain, target: nil, action: nil)
enum BarButton {
case done(title: String)
case edit(title: String)
func button() -> UIBarButtonItem {
switch self {
case .done(let title): return UIBarButtonItem(title: title, style: .done, target: nil, action: nil)
case .edit(let title): return UIBarButtonItem(title: title, style: .plain, target: nil, action: nil)
}
}
}
let done = BarButton.done(title: "Save")
let button = done.button()
Ethan Neff
27,058 Pointstry this:
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case .Done(let str): return UIBarButtonItem(title: str, style: .Done, target: nil, action: nil)
case .Edit(let str): return UIBarButtonItem(title: str, style: .Plain, target: nil, action: nil)
}
}
}
let done = Button.Done("Done")
let doneButton = done.toUIBarButtonItem()
you're missing the part where you are passing the associated value through the switch statement .Done(let str)
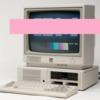
kols
27,007 PointsJoe Hill's above answer is correct (thanks, Joe!). You can alternatively move the constant declaration keyword (i.e., "let") outside of the parentheses (code shown below). For more details, check out Apple's Swift documentation on Enums (particularly focus on Associated Values and Recursive Enumerations sections).
enum BarButton {
case done(title: String)
case edit(title: String)
func button() -> UIBarButtonItem {
switch self {
case let .done(title): return UIBarButtonItem(title: title, style: .done, target: nil, action: nil)
case let .edit(title): return UIBarButtonItem(title: title, style: .plain, target: nil, action: nil)
}
}
}
let done = BarButton.done(title: "Save")
let button = done.button()
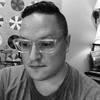
Joe Hill
15,063 PointsYes it did. I had the titles mixed up. I updated my answer above so it should be correct now. My cases should have just said:
case .done(let title): return UIBarButtonItem(title: title, style: .done, target: nil, action: nil)
case .edit(let title): return UIBarButtonItem(title: title, style: .plain, target: nil, action: nil)
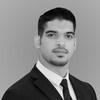
Laith Mohammad
6,569 PointsThis is a pointless, unclear example, there's no need to use UI component in explaining a programming language, this is a swift course , not an xcode IOS development course.
Dear treehouse team, please use a straight forward example instead.
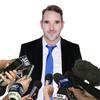
Kristopher Wood
iOS Development Techdegree Student 5,552 PointsThis course is also used in the iOS development Techdegree. It's a valid example there.

Conor Mosier
3,866 PointsI'm curious as to why after .done you guys put (let title). can someone explain this for me please?
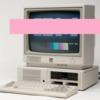
kols
27,007 PointsFor anyone curious:
We do this — declare a local constant, e.g., .done(let title)
or let .done(title)
— because in order to use an enum member's associated value in the switch statement, you must extract it by creating a local constant to work with. We want to be able to pass this value through and utilize it in the return statement, so first we must name it ("Bastian, please; save us!") so that we can reference it (in this case, passing its value through for the title).
For more, check out the Swift documentation on Enums, particularly the Associated Values section.
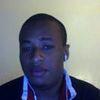
Abdoulaye Diallo
15,863 Pointsenum BarButton { case done(title: String) case edit(title: String)
func button() -> UIBarButtonItem {
switch self {
case .done(let title): return UIBarButtonItem(title: title, style: .done, target: nil, action: nil)
case .edit(let title): return UIBarButtonItem(title: title, style: .plain, target: nil, action: nil)
}
}
}
let done = BarButton.done(title: "Save") let button = done.button()
Dan Hickey
3,016 PointsDan Hickey
3,016 PointsDid this pass the challenge?
Evans Attafuah
18,277 PointsEvans Attafuah
18,277 Pointssamson akisanya
iOS Development Techdegree Student 6,329 Pointssamson akisanya
iOS Development Techdegree Student 6,329 Pointsyea this works. but couldn't find any documentation on UIBarButtonItem