Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial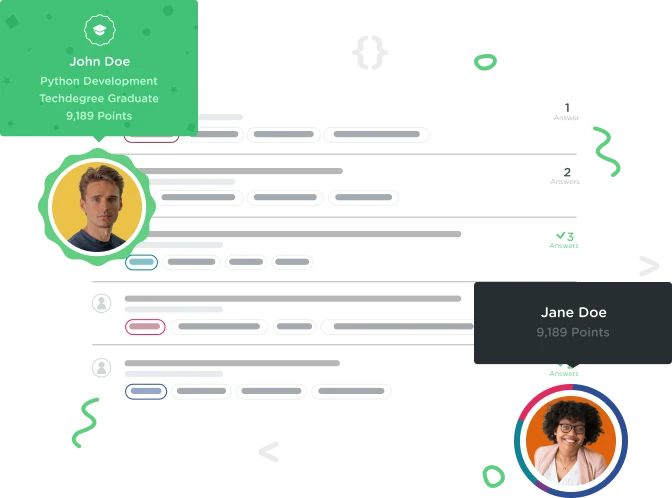
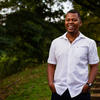
Julian Cortes
3,549 PointsHi, im using MAMP php 5.6.2, when I try to load echo de info of the object soda is showing nothing in my browser
Im using this code:
<?php class Product{
// Propiedades
public $name = 'Nombre default';
public $price;
public $desc;
// Constructor
function __construct($name, $price, $desc){
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
// Metodos
public function getInfo(){
// Return some info
return "Nombre del Producto: ". $this->name;
}
}
class Soda extends Product{
public $flavor;
// Constructor
function __construct($name, $price, $desc, $flavor){
parent::__construct($name, $price, $desc);
$this->flavor = $flavor;
}
public function getInfo(){
return "Nombre del Producto: ". $this->name . " Flavor: ". $this->$flavor;
}
}
$p = new Product(); // Nuevo objeto usando el constructor $shirt = new Product("Camisa de Julian",20,"Camisa blanca manga siza"); $soda = new Soda("Sprite",5,"Gaseosa sprite","Uva"); // Muestra en pantalla echo $soda->getInfo();
2 Answers

Joe Cochran
18,249 PointsI've identified two issues in your code that need to be fixed in order for this script to work.
first, on line 32 in Soda->getInfo:
public function getInfo(){
return "Nombre del Producto: ". $this->name . " Flavor: ". $this->$flavor;
}
The call to $this->$flavor should be $this->flavor, no dollar sign needed!
Then, on line 36, you are creating a new instance of product without passing the required arguments to the constructor.
$p = new Product(); // Nuevo objeto usando el constructor
This is generating a healthy number of PHP warnings, however, with warnings suppressed the script works as expected. I would recommend moving your default declarations to the constructor like so:
function __construct($name = 'Nombre default', $price = 0, $desc = "default description"){
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
For the big picture, here is the entire, corrected script. I'll comment where I modified things:
<?php
<?php class Product{
// Propiedades !!!! removed default name from property declaration.
public $name;
public $price;
public $desc;
// Constructor !!!!! added defaults to the passed parameters.
function __construct($name = 'Nombre default', $price = 0, $desc = "default description"){
$this->name = $name;
$this->price = $price;
$this->desc = $desc;
}
// Metodos
public function getInfo(){
// Return some info
return "Nombre del Producto: ". $this->name;
}
}
class Soda extends Product{
public $flavor;
// Constructor
function __construct($name, $price, $desc, $flavor){
parent::__construct($name, $price, $desc);
$this->flavor = $flavor;
}
public function getInfo(){ //removed unneeded dollar sign from $this->flavor
return "Nombre del Producto: ". $this->name . " Flavor: ". $this->flavor;
}
}
$p = new Product(); // Nuevo objeto usando el constructor
$shirt = new Product("Camisa de Julian",20,"Camisa blanca manga siza");
$soda = new Soda("Sprite",5,"Gaseosa sprite","Uva"); // Muestra en pantalla
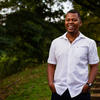
Julian Cortes
3,549 PointsAwesome explanation, i didn't figure it out... my error was $this->$flavor but i also clean my test code with this instructions so thank you !