Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial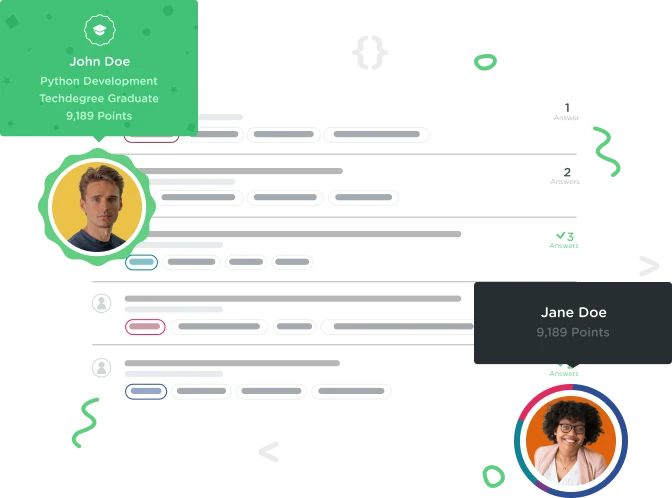
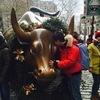
Jeremy Yi
9,512 PointsHi, Pasan. What is the static key word stand for within the Formatter struct?
I am just curious about when do we need the key word static. I checked the book but still not quiet understand.
1 Answer

Martin Wildfeuer
Courses Plus Student 11,071 PointsBasically, there are two different types of methods and properties:
- Instance methods and instance properties
- Type methods and type properties
Instance methods and instance properties
These can only be called or accessed on an instance of a class. With the first example, you have to create and instance of UIColor first, before you can call the blackColor
method or access the one
variable. That is a concept you might be already familiar with. You have full access to all other methods and properties.
class UIColor {
var one: Int = 1
func blackColor() -> UIColor {
// Create black color here and return it...
}
}
// Create an instance of UIColor
let color = UIColor()
// Call method on instance of UIColor
let blackColor = color.blackColor()
// Access property on instance of UIColor
let one = color.one
Type methods and type properties
Sometimes referred to class methods // properties. These can be called and accessed on the class itself, no need to create an instance of UIColor first. This is often used for convenience methods and properties, just as UIColor. The below example shows the actual implementation of the convenience method to create a black color.
class UIColor {
// Note this uses the static keyword,
// not class. Find the explanation below
static var one: Int = 1
class func blackColor() -> UIColor {
// Create black color here and return it...
}
}
// Access the blackColor method on the class
// without having to create an instance of UIColor
let blackColor = UIColor.blackColor()
// Access the one property directly
let one = UIColor.one // 1
Important!
It is important to know that class and static methods and properties don't have access to instance methods and properties! The following example does not compile:
struct Test {
let testing = "Testing"
static func test() -> String {
// In static or class methods, we cannot access
// instance methods and properties
return testing
}
}
Class vs. Static methods and properties
As the name suggests, you can have class methods only with classes, not structs. That makes sense, as structs don't have the concept of inheritance, unlike classes. However you can have static methods and properties on classes. The key difference is that class methods and properties can be overwritten in subclasses whereas static methods and properties can't be overridden.
Hope that helps :)
Jeremy Yi
9,512 PointsJeremy Yi
9,512 PointsThanks Martin. It is a big help.