Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial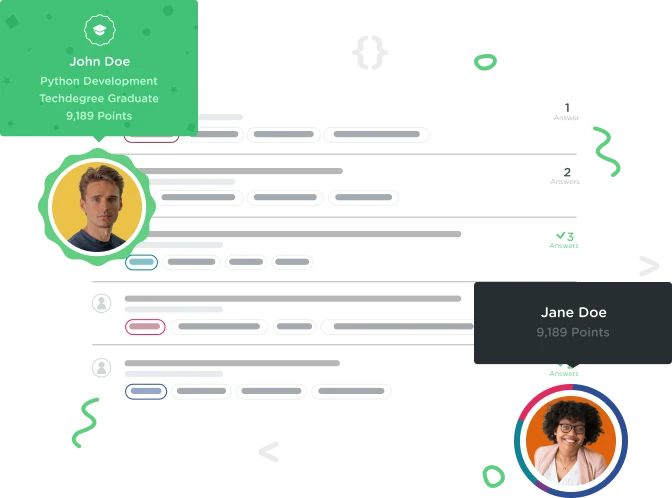
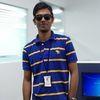
Udayakumar T
11,544 PointsHi please clarify the question, how to calculate the remaining characters??
How to find the remaining character value from the maximum constant value.
public class Tweet {
private String text;
public static final int MAX_CHARS = 140;
public Tweet(String text) {
this.text = text;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public int getRemainingCharacterCount()
{
int textlength = text.length();
int result = MAX_CHARS - textlength;
return result;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi there....
You can just directly return the result of MAX_CHARS subtracted by text.length() method:
public int getRemainingCharacterCount() {
return MAX_CHARS - text.length();
}
I hope this can help a little
PS: It will be easier if you keep in mind that String is also an object like class. String also has methods one of them is String.length(). When you read the Oracle Java Doc on String you will see a list of methods related to String class. Basically this length() method also written in code like any other Java class method:
public int length() {
//bla.... bla.....
}
The main point is this is an abstraction meaning that you do not need (and it will be better that you do not) knowing how the methods works. You only need to understand that it returns the length of your String in integer format. Therefore since the constant MAX-CHARS is also an integer you can perform mathematical operations on them. Thus you can find how many remaining character left can be submitted inside a tweet by subtracting the MAX_CHARS with the text.length();
Udayakumar T
11,544 PointsUdayakumar T
11,544 Pointsthx ...