Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial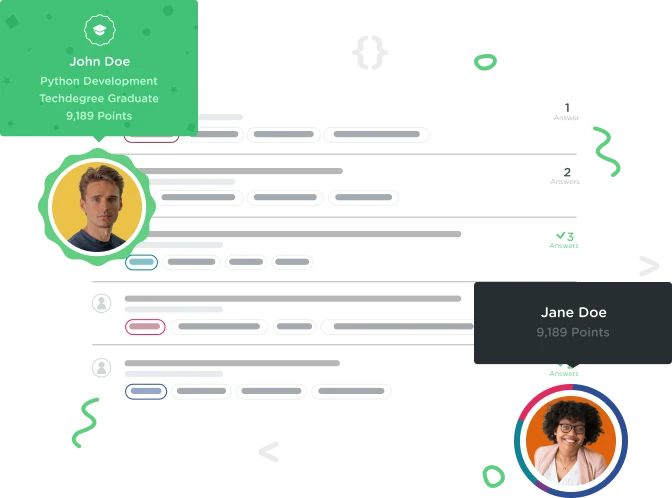

Jason Woo
11,730 PointsHi there I redid the contact_list project on my own and cannot diagnose this issue. Could you tell me what's wrong?
def ask(question, type = "string") puts question + " " answer = gets.chomp if type == 'number' answer = answer.to_i end return answer end
contact_list = []
def add_contact contact = {'name' => '', 'phone_numbers' => []} contact['name'] = ask('What is the name of the contact? ') add_number ="" until add_number == 'n' add_number = ask("Add a number for #{contact['name']}? (y/n)") if add_number == 'y' contact['phone_numbers'].push(ask('Enter a number for ' + contact['name'])) end end return contact end
def show contact_list.each do |contacts| puts "Name: #{contacts['name']}" contacts['phone_numbers'].each do |numbers| puts "Number: #{numbers}" end puts "------------ \n" end end
answer = "" until answer == 'n' contact_list.push(add_contact) answer = ask('Would you like to add another contact? (y/n)') end
show
4 Answers
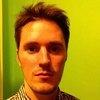
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI ran your code and I got this error when I got to the end:
contact_list.rb:26:in `show': undefined local variable or method `contact_list' for main:Object (NameError)
from contact_list.rb:41:in `<main>'
Is that the problem you're referring to? If so, this seems to be a problem with variable scope. This stack overflow answer was enlightening: https://stackoverflow.com/questions/9671259/ruby-local-variable-is-undefined
I applied this to "define method" change to your code and it solve that error, see line line 26 in the code below:
def ask(question, type = "string")
puts question + " "
answer = gets.chomp
if type == 'number'
answer = answer.to_i
end
return answer
end
contact_list = []
def add_contact
contact = {'name' => '', 'phone_numbers' => []}
contact['name'] = ask('What is the name of the contact? ')
add_number =""
until add_number == 'n'
add_number = ask("Add a number for #{contact['name']}? (y/n)")
if add_number == 'y'
contact['phone_numbers'].push(ask('Enter a number for ' + contact['name']))
end
end
return contact
end
define_method :show do
contact_list.each do |contacts|
puts "Name: #{contacts['name']}"
contacts['phone_numbers'].each do |numbers|
puts "Number: #{numbers}"
end
puts "------------ \n"
end
end
answer = ""
until answer == 'n'
contact_list.push(add_contact)
answer = ask('Would you like to add another contact? (y/n)')
end
show

Jason Woo
11,730 PointsYes, I received the same error.
I don't understand why contact_list is unavailable when I already defined it in the outermost scope (aka local variable correct?). My previous understanding was that methods and classes should be able to access them.
Based on what I read in your link, my new understanding is methods (written as def or class) can only access variables defined within themselves, whereas classes can access outermost/local variables. Is that right?
Your edit on line 26 allows for the method to take from local variables (like classes), and can be called upon like a normal method without the :(colon). Correct?
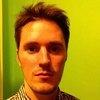
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsHonestly, it surprised me too. I'm more of a JavaScript guy, so my understanding of scope is from that language, and this isn't how I expected it to behave. But I guess that's ruby.

Jason Woo
11,730 PointsI also want to make sure I have the syntax of define_method nailed down.
1) The colon is necessary (I tried it without and ran an error when called) 2) Requires the word 'do', unlike a regular method definition. 3)In order to call the method, it has to be without the colon (so its show, not :show in our case).
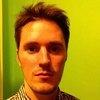
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI'm sorry I can't speak with very much authority about Ruby syntax.

Jason Woo
11,730 PointsNo worries, those were just the conclusions I came to from experimenting.
Thank you so much for help!