Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial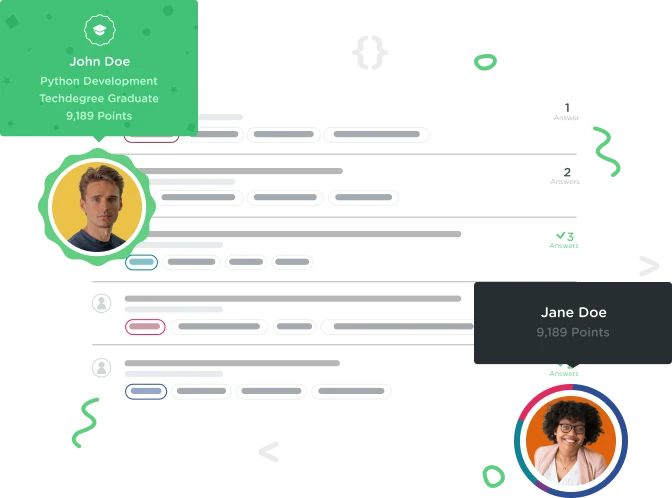

Junwen Lee
8,272 PointsHi Treehouse, Why can we see a HTML change in the browser, when we change the web URL?
Can someone explain the intuition in the code found in router.js and app.js, which leads to this effect?
Particularly, what is the logic that changes the response.write effect from "Search" to "Chalkers?", when we edit the URL in the browser?
Thanks!
5 Answers

Petar Popovic
Courses Plus Student 23,421 PointsOkay, I think I know what's confusing you. It also confused me, but then I played with console.log, read documentation, and I think I figured it out.
We can see the change because we set variable username instead of Search string. And our variable username is var username = request.url.replace("/", "");
My first thought was, ok, this will convert anything I put after domain name in empty string. But I was so wrong.
request is an object.
request.url is everything that comes after the domain or ip adress. Example: www.domain.com/requestUrl
We just take that part /requestUrl, and remove that first / to get only this requestUrl part. So we can display only that part.
Otherwise if we say this:
var username = request.url;
if(username.length > 0) {
response.writeHead(200, {'Content-type':'text/plain'});
response.write("Header \n");
response.write(username + "\n");
response.end('Footer \n');
}
We get this output:
- Header
- /requestUrl
- Footer
This is how I understand it, doesn't need to be right.
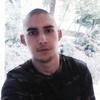
Farid Wilhelm Zimmermann
16,753 PointsAs some people already wrote previously, you want the server to respond differently, when trying to access different part of your webpage. To accomplish this via Node.JS, we use what is called Routing.
/2. Handle HTTP route GET / and POST / i.e. Home
function homeRoute(request, response) {
if(request.url === "/") {
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header\n");
response.write("Search\n");
response.end('Footer\n');
}
}
First Step: Declare a function homeRoute(), that takes two parameters, request and response.
As shown in the Node.JS Documentation, we can access the request url, by accessing the request objects url property.
We then check, if it is equal to /. This stands for our home directory. currently, all our requests direct to our home directory (as can be seen in the previous vid, where Andrew tries out adding usernames behind the /, it all showed the same content).
So if our request.url equals /, then do
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header\n");
response.write("Search\n");
response.end('Footer\n');
Second Step: Now we need to declare a second function, userRoute(), to check whether we got anything added behind our / (as this shouldnt route to our home directory anymore).
We do this by:
var username = request.url.replace("/", "");
if(username.length > 0) {
response.writeHead(200, {'Content-type':'text/plain'});
response.write("Header \n");
response.write(username + "\n");
response.end('Footer \n');
}
First, we replace any / inside our url with an empty string. We save the request.url in a new variable, username Then, we check if username.length is greater than 0. This step is needed to rule out the case, that no username is provided (like when we are trying to just access our home directory for instance).
If the username.length is greater than 0, meaning we got a username provided inside our url string, we then
response.writeHead(200, {'Content-type':'text/plain'});
response.write("Header \n");
response.write(username + "\n");
response.end('Footer \n');
meaning that instead of writing search to the webpage (as we do when accessing the home directory), we print out the username provided. All those printouts are currently just placeholders, to demonstrate that we successfully routed our server request to different "parts" of our webpage, and are also able to get hold of the username (which will be needed for further processing later on).
The main thing to take away from this lesson is, that we can create websites dynamically, by checking the url provided, and change content suited to each request.
Happy coding :)
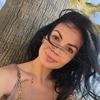
Iulia Maria Lungu
16,935 Pointsi did not understand the same thing. hoping for someone to clear this out!
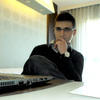
Daniel Nitu
13,272 PointsFrom what I can tell, when you use the http.createServer function, given that you create it on the Treehouse server, it will automatically generate this url: http://port-3000-63r1x632go.treehouse-app.com/ and will output to the page whatever is written in response.write(); and response.end();, if the url ends with '/'
The replace() method just takes out the slash /, because you will write it anyway when typing the username in the link. ex: http://port-3000-63r1x632go.treehouse-app.com/username.
Once the browser sees that your url doesn't end in / and that the username has a length greater than 0, it will display username instead of Search.
After the / in the url, you can basically type anything you like, for example you can get an url like: http://port-3000-63r1x632go.treehouse-app.com/asdf;jklasdf;jklasdf;kl, and this message in the browser:
Header
asdf;jklasdf;jklasdf;kl
Footer
This is jut my take on it. I might be wrong. It there's anyone else willing to explain, I'd be great.

Bartosz Kubisz
26,277 PointsHi Junwen! Sorry but I'm not familiar with Node.js yet. B.