Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial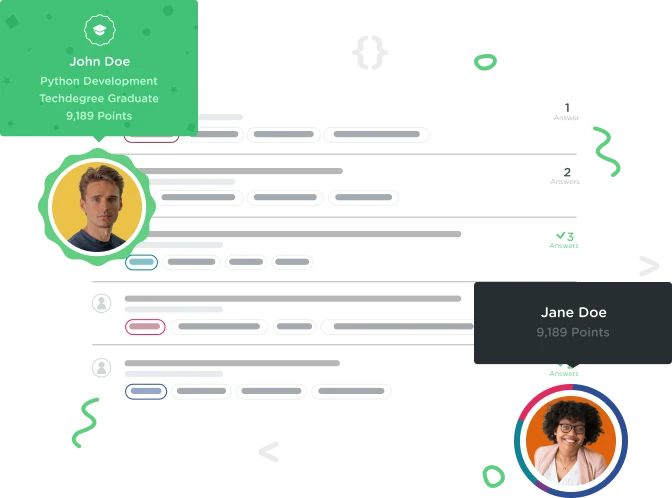

tqqpljmgzy
3,430 PointsHi why is this not formatting correctly?
When I run this in a python 3.5 shell I get the incorrect formatting.
import datetime
datetime.datetime.strptime("19/01/2016", "%d/%m/%Y")
In python 3.5 I get the follwing: datetime.datetime(2016, 1, 19, 0, 0) the correct format should be day/month/year - the shell is ignoring the formatting. What have I done wrong? Thanks.
4 Answers
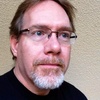
Chris Freeman
Treehouse Moderator 68,423 PointsIf you're trying to produce a string use str*ftime. To create a new datetime
From a string use strp*time. See docs strftime strptime behavior
Update: so say you have a datetime
object. When you inspect this object by printing it out, the value printed is the __repr__
representation which is a text string that will re-create the datetime
object if used as an argument to the eval()
function.
The string
datetime.datetime(2016, 1, 19, 0, 0)
is the text representation of the datetime
object. Playing with int in the REPL....
>>> import datetime
>>> datetime.datetime.strptime("19/01/2016", "%d/%m/%Y")
datetime.datetime(2016, 1, 19, 0, 0)
>>> dtime = datetime.datetime.strptime("19/01/2016", "%d/%m/%Y")
>>> type(dtime)
<class 'datetime.datetime'>
>>> dtime
datetime.datetime(2016, 1, 19, 0, 0)
>>> dtime.strftime("%d/%m/%Y")
'19/01/2016'
>>>
EDIT: Adding chaining comment from below
You could chain this way:
# from this
date = datetime.datetime(day=19, month=01, year=2016)
string = date.strftime("%d/%m/%Y")
# to this
string = datetime.datetime(day=19, month=01, year=2016).strftime("%d/%m/%Y")
# or this
string = datetime.datetime.strptime("19/01/2016", "%d/%m/%Y").strftime("%d/%m/%Y")

tqqpljmgzy
3,430 PointsThanks for the reply.
Yes I want a new datetime from a string.
So why is it not getting formatted correctly. The docs state "Return a datetime corresponding to date_string, parsed according to format." However the python shell is ignoring the format provided, it is simply using the default for some reason.
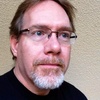
Chris Freeman
Treehouse Moderator 68,423 PointsReferring to a datetime
object and getting a specific string representation of that datetime
object are two separate things. I've updated my previous answer.

tqqpljmgzy
3,430 PointsOk so they are two different things. But why can't I do it is one step via chaining?
That is;
date = datetime.datetime.strptime("19/01/2016", "%d/%m/%Y") << and get the result '19/01/2016'
Opposed to
date = datetime.datetime(day=19, month=01, year=2016)
date = date.strftime("%d/%m/%Y") to get the desired result '19/01/2016'
Thanks
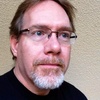
Chris Freeman
Treehouse Moderator 68,423 PointsYou could chain this way:
# from this
date = datetime.datetime(day=19, month=01, year=2016)
string = date.strftime("%d/%m/%Y")
# to this
string = datetime.datetime(day=19, month=01, year=2016).strftime("%d/%m/%Y")
# or this
string = datetime.datetime.strptime("19/01/2016", "%d/%m/%Y").strftime("%d/%m/%Y")

tqqpljmgzy
3,430 PointsGreat thanks.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsFunny. I just came across a code challenge that uses code similar to your code. The challenge asks to convert a 12-hour time into a 24-hour time.
https://www.hackerrank.com/challenges/time-conversion
Solution: