Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial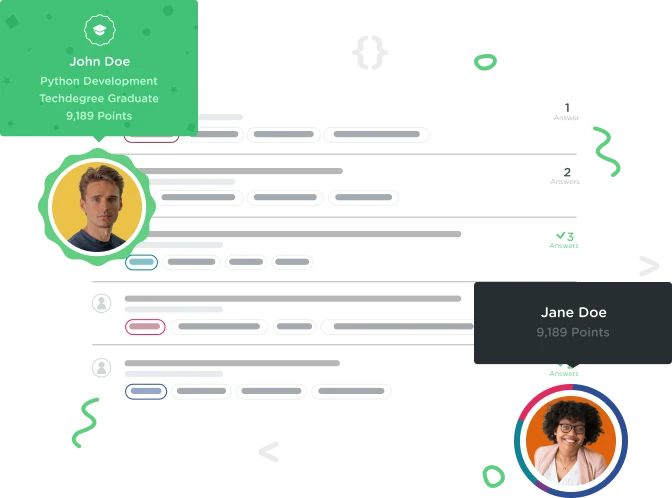

Tiemoko Bamba
1,923 PointsHi would someone help me solve this problem Please?
I've provided a base class Person in the editor below. Once an instance of Person is created, you can call getFullName() and get a person's full name.
Your job is to create a class named Doctor that overrides the getFullName() method. Once you have a class definition, create an instance and assign it to a constant named someDoctor.
For example, given the first name "Sam", and last name "Smith", calling getFullName() on an instance of Person would return "Sam Smith", but calling the same method on an instance of Doctor would return "Dr. Smith".
2 Answers
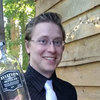
Evan Demaris
64,262 PointsYou're REALLY close with that code; you don't need the super.getFullName() part of it, though. Also, you wouldn't want your code to specify the doctor's name as Smith, because the doctor's last name will be the lastname
that the class takes as an argument when it's instantiated.
Here's what this code would look like if you let the lastName be the argument and remove the super.getFullName; this should pass the Challenge.
class Doctor : Person {
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
someDoctor.getFullName()
Also, you might want to check out this link to correctly format posted code next time:
https://teamtreehouse.com/community/posting-code-to-the-forum
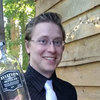
Evan Demaris
64,262 PointsHi Tiemoko,
This question is asking for you to make a subclass, Doctor, which has its own getFullName() method. Below is how class inheritance works in Swift. Doctor would be the Subclass, and Person would be the Superclass.
class SomeSubclass: SomeSuperclass {
// subclass definition goes here
}
Once you've created the doctor Subclass and given it an updated getFullName method, you'll need to make a variable called someDoctor with an instance of the Doctor Subclass assigned to it - make sure to include a name when you call that instance.

Tiemoko Bamba
1,923 PointsHi Evan! hmm would this look a little bit more of what you were talking about ? and thanks for your responds!
OH! and one more thing when ever I Recheck Work my work. I get this... "Bummer! Double check the implementation of the overridden method to match the directions specified" and I'm pretty much a little confuse on the implementation part lol ?
class Person { let firstName: String let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below class Doctor: Person {
override func getFullName() -> String {
super.getFullName()
return "Dr. Smith"
}
} let someDoctor = Doctor(firstName: "Sam",lastName: "Smith") someDoctor.getFullName()
Tiemoko Bamba
1,923 PointsTiemoko Bamba
1,923 PointsWow! I was close lol. I Totally forgot that I could have used the String interpolation for the lastName inside the the return... I wouldn't have figure that out if it wasn't for your HELP. Thank you Evans!
Also Thanks for the link