Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial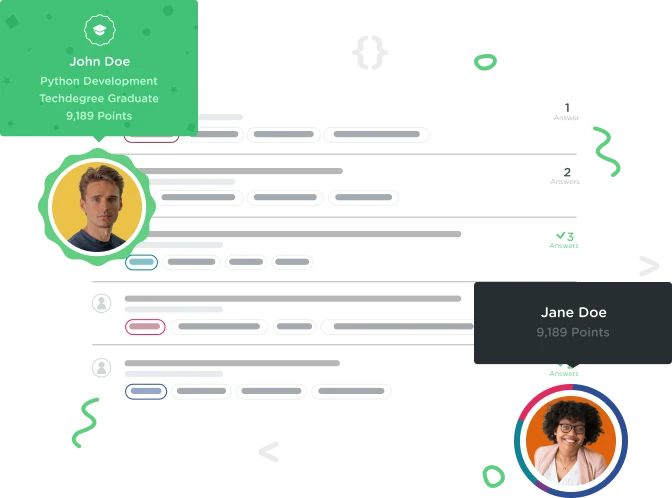
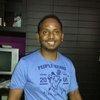
Karrtik Iyer
3,738 PointsHibernate, how would we handle if we had two classes implementing the service interface?
In this video it was mentioned that Hibernate/Spring would identify the implementation class based on the auto wiring. Suppose if I have a base implementation of a service which I would like to extend, so that if my URI/controller is hit I would like to use my implementation of service else if base URI or controller is hit, it should use the base implementation of the service, how would we achieve that, is there some parameter in auto wiring which we can specify which tells against which implementation the auto wiring should happen?
3 Answers

Chris Ramacciotti
Treehouse Guest TeacherYou could accomplish this with Spring qualifiers. Consider these services:
@Service("parent")
public SomeServiceImpl implements SomeService { ... }
and this for the child class:
@Service("child")
public SomeChildServiceImpl extends SomeServiceImpl { ... }
When autowiring, use the @Qualifier
annotation to specify which version you'd like:
@Autowired
@Qualifier("parent")
private SomeService parent;
@Autowired
@Qualifier("child")
private SomeService child;
Maybe you asked this question completely out of curiosity - there is serious value there. :) But, if you're looking to actually use this approach in the scenario you mentioned, I'd think carefully about object-oriented and service-DAO design principles. Typically you'd roll your service operations for a single resource and for collections of that resource into a single class. You could definitely create a base class for all your services, but that's about reducing the amount of code duplication by consolidating logic. Your controller shouldn't have to make a decision on which service "version" to use. Doing this forces your controller layer to know more about the service layer, which is a step away from "loose coupling".
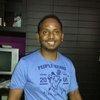
Karrtik Iyer
3,738 PointsThanks Chris for your response, I shall go through the same and get back to you about my thoughts on the same. In our current project, we are faced with a situation where we have extended a REST endpoint provided by the base framework to add some extra attributes which base service doesn't support. And we are getting a unique bean exception for DAO when we access the base REST resource, while when using the specialized one, it works fine, hence was wondering how this works out. So does it mean that ideally we would never have an interface implemented by more than 1 class in Spring MVC/Service - DAO design principles?

Chris Ramacciotti
Treehouse Guest TeacherIn general, yes. But as I said above, you have some flexibility in implementation with qualifiers, and even Spring profiles. Without seeing your source code, it's hard to say what the best approach would be.