Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial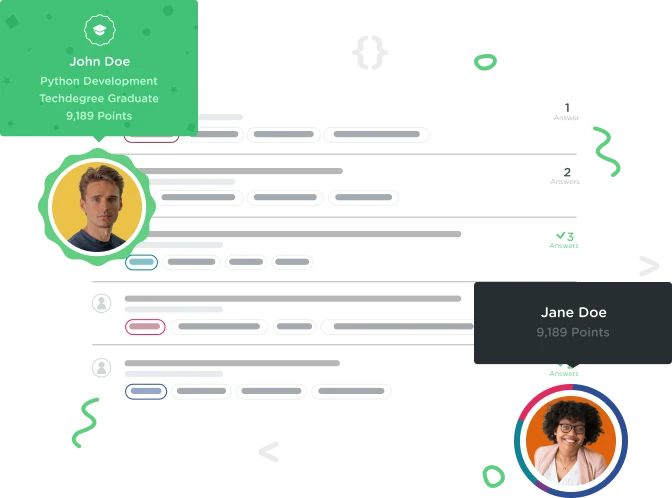
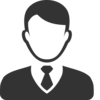
gyatmnucla
7,618 PointsHide character with css ?
Hi all, the wordpress theme i am using for a project, renders some testimonials that i input in the back-end with quotes. I have added an open-quote image of my own, but i cant remove the text quotes, as they are added by the theme. What is the best way to remove these quotes?
Thanks in advance :)
3 Answers

Brian Hayes
20,986 PointsThis has to do with the function(s) the theme is using to build the markup of the testimonial. If the theme built a filter into those functions, then you could filter the markup, but if not, then you will have to edit the function yourself, which, to be fair, is something you'll have to keep an eye on when you update the theme. A better solution would be to use a child theme.
There are a few ways to go about this, and a lot is determined by how the parent theme is coded. Here are some situations:
1) If the parent theme wraps the function you wish to change with a conditional checking if the function already exists, then you can simply define a function in your child theme's functions.php
file with the same name, and effectively replace the function. A wrapper would most likely look like this:
<?php
if ( ! function_exists( 'parent_testimonial_markup' ) ) {
function parent_testimonial_markup() {
// Create markup for Testimonial Widget/Shortcode/CPT
}
}
2) If there is no conditional wrapper, but the function is hooked into an action hook correctly, then you can remove that action, copy and paste the function into your child theme's functions.php
file, change the function prefix, make your modifications, and hook the new function into the exact same hook you just removed the parent function from.
So, let's say that here is the function you want to change in the parent theme:
<?php
/**
* Add wrapping quotations to testimonial
*
* @param string $instance Most likely the value stored in the database from the testimonial custom post page in admin.
*
* @return void
*/
function parent_testimonial_wrapping_quotes( $instance ) {
echo '<p>"' . $instance . '"</p>';
}
// Hook markup into WP
add_action( 'correct_wordpress_hook', 'parent_testimonial_wrapping_quotes' );
The first thing you should notice is the add_action()
function. This is the hook that you'll want to remove using your child theme. Do this by simply adding:
<?php
// Unhook parent theme function.
remove_action( 'correct_wordpress_hook', 'parent_testimonial_wrapping_quotes' );
Now, copy and paste over the parent function and rename the function, or simply swap the prefix on it. Meaning parent_testimonial_markup()
would be renamed to child_testimonial_markup
. The name of the function technically doesn't matter, just as long as it isn't the same name as any other defined function.
So now that function may look something like this:
<?php
/**
* Add new quotation image to testimonial markup
*
* @param string $instance Most likely the value stored in the database from the testimonial custom post page in admin.
*
* @return void
*/
function child_testimonial_wrapping_quotes( $instance ) {
echo '<p><span class="quote-image"></span>' . $instance . '</p>';
}
All you need to do now is hook this new function into the same hook that you removed the parent theme function from:
<?php
// Hook new child function into WP
add_action( 'correct_wordpress_hook', 'child_testimonial_wrapping_quotes' );
And that should be it. You should now have a new function overwriting the old one.
Now it's very possible that editing a set of functions that build out the markup for testimonials will be much more involved, since usually something like that is basically a bunch of functions working with each other ending with a final function that puts it all together and gets hooked into WordPress.
3) There is one final way I can think of to solve this, but it is super dependent on a specific method being employed by the parent theme. This is a CSS method that doesn't involve having to dive into the parent theme's functions.php
file, but is something you can find out by using Chrome Dev Tools, or Firebug.
If the testimonials have the actual quote appearing in the markup like this:
<blockquote>Pellentesque habitant morbi tristique senectus et netus.</blockquote>
Yet, you still see quotations, then the ::before
and ::after
pseudo-classes may be being used in the parent theme's CSS like this:
blockquote:before,
blockquote:after {
content: '"';
}
You could then just simply overwrite this declaration in your child theme's style.css
and be done with it. Note that the target is probably a lot more specific than that, but if you inspect the element with Chrome Dev Tools, then it will show you exactly how the testimonial text is targeted.
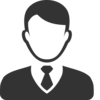
gyatmnucla
7,618 PointsOk, so I am using a child theme. The theme of this website will not be updated, thus i should change the function directly. The thing is that i don't really know how to locate the function..
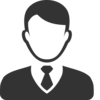
gyatmnucla
7,618 PointsCould the theme use php to add the quotes aswell ?