Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial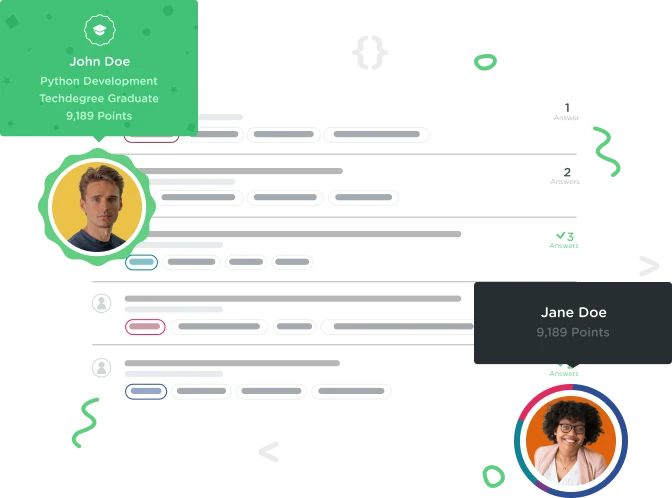

Travis Dalhoff
21,035 PointsHi....I've been stuck on this for awhile. My code is not compiling. Any suggestions? Thanks!
Thanks for your help!
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
namespace Treehouse.CodeChallenges
{
public class Course
{
public Course()
{
Students = new List<CourseStudent>();
}
public int Id { get; set; }
public int TeacherId { get; set; }
[Required, StringLength(200)]
public string Title { get; set; }
public string Description { get; set; }
public int Length { get; set; }
public Teacher Teacher { get; set; }
public ICollection<CourseStudent> Students { get; set; }
}
}
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public static class Repository
{
public static List<Course> GetCourses()
{
using (var context = new Context())
{
return context.Courses.ToList();
}
}
public static List<Course> GetCoursesByTeacher(string lastName)
{
using (var context = new Context())
{
var Courses = context.Courses.Where(cb => cb.Teacher.lastName == lastName).ToList();
return Courses;
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Teacher
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
}
1 Answer

James Churchill
Treehouse TeacherTravis,
When you're looking for help with a compiler error, it's helpful to include the error message that you're receiving.
Scanning your code, I noticed in the GetCoursesByTeacher
method the following line of code:
var Courses = context.Courses.Where(cb => cb.Teacher.lastName == lastName).ToList();
Teacher.lastName
doesn't reference a property on the Teacher class. The actual property name is LastName
(starting with a capital "L"). I hope this helps.
Thanks ~James