Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial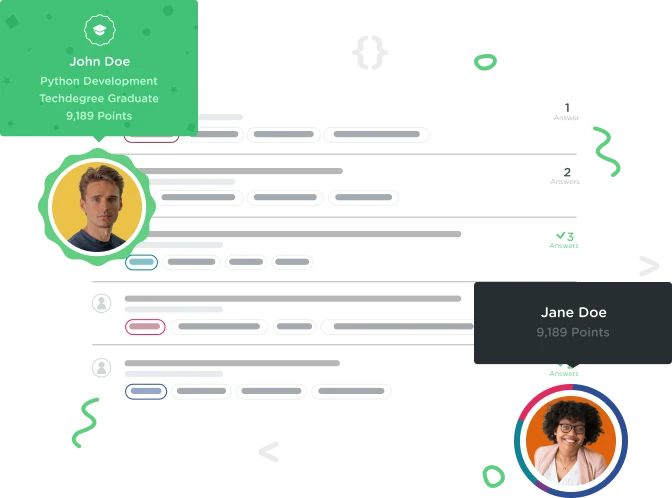
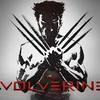
Wolverine .py
2,513 Pointshiya i am stuck how do i make a function called add_list with only numbers in it and should return the total
Make a function named add_list that takes a list. The function should then add all of the items in the list together and return the total. Assume the list contains only numbers. You'll probably want to use a for loop. You will not need to use input().
add_list = list([1, 2, 3])
def add_list([1, 2, 3]):
return 1+2+3
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
3 Answers
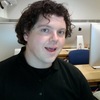
Nathan Smutz
9,492 PointsIt looks like you're having trouble with functions. Here's how they look:
def i_am_a_function( i_am_an_argument ):
... (we do something with the i_am_an_argument here)...
return i_am_the_thing_the_function_returns
When you use the function it looks like this:
i_will_equal_the_thing_the_function_returns = i_am_a_function( a_variable_or_something )
or if you just want to print things out:
print(i_am_a_function( a_variable_or_something ))
Here's how your function will look in usage:
my_list = [1, 2, 3, 4]
sum_of_my_list = add_list(my_list)
print(sum_of_my_list)
>>>10
or:
add_list([1,2,3,4])
>>> 10
So we've been told that our function needs to take a list and add up the numbers in the list and return the total. So we know this much:
def i_am_a_function( i_am_a_list ):
...(add up the numbers in the list)...
return the_total_of_the_numbers_in_the_list
Let's rename things a little to match what you're doing:
def add_list( list_of_numbers ):
...(add all the numbers together so that the sum is named "total")...
return total
Here is is fleshed out:
def add_list ( list_of_numbers ):
total = 0; # We will add all the numbers to this variable
for i in list_of_numbers: # For each number in the list
total = total + i # add that number to the sum of all the previous numbers
return total # Return the total
Used like this:
my_list = [1, 2, 3, 4]
sum_of_my_list = add_list(my_list)
print(sum_of_my_list)
>>>10
or:
add_list([1,2,3,4])
>>> 10
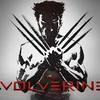
Wolverine .py
2,513 PointsThank you very helpful. i got the concept, i have done everything you have taught me.
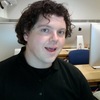
Nathan Smutz
9,492 PointsNice :-) Did the rest of the exercise work out well?
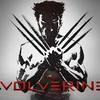
Wolverine .py
2,513 Pointsthank you Nathan, you pretty much helped me with everything. and it worked. you the man!!!!!!!!. i will ask you any questions if i am stuck hey.