Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial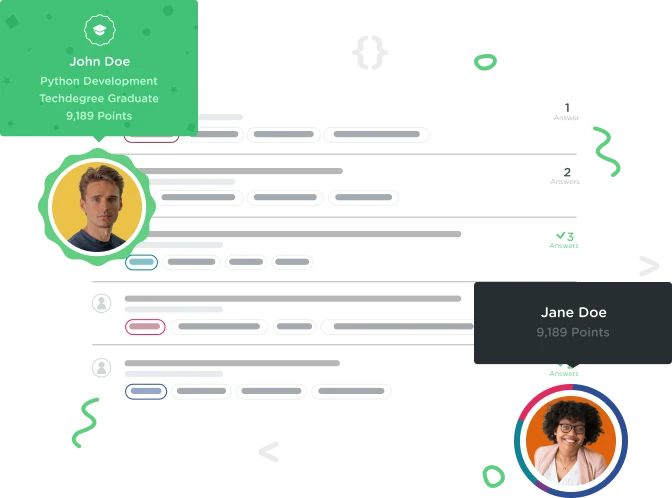
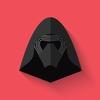
Stu Cowley
26,287 PointsHmm FunFacts Stopped Working Error
Hi everyone,
I have come across an error that I have no idea how to fix as I am getting no errors from Android Studio. I will admit I am using the latest version of Android Studio (2.3) and not the same version used in the project as I had a lot of issues installing it and was pretty much forced to use the latest version.
When I run the project with the emulator I can an error that says: "Fun Facts has Stopped" with a button to open the app again. I do this and get the same error and then I am presented with another error that says "Fun Facts keeps stopping" with a button that says Close app. Obviously this closes the app.
Can anyone shed some light on this for me as I would have no idea where to look. Please see below, I have included the code that I am using:
FunFactsActivity.java
package com.stucowley.funfacts;
import android.content.DialogInterface;
import android.graphics.Color;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends AppCompatActivity {
private FactBook mFactBook = new FactBook();
// Declare our view variables
private TextView mFactTextView;
private Button mShowFactButton;
private RelativeLayout mRelativeLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Assign the Views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factTextView);
mShowFactButton = (Button) findViewById(R.id.showFactButton);
mRelativeLayout = (RelativeLayout) findViewById(R.id.relativeLayout);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = mFactBook.getFact();
// Update the screen with our dynamic fact
mFactTextView.setText(fact);
mRelativeLayout.setBackgroundColor(Color.RED);
}
};
mShowFactButton.setOnClickListener(listener);
}
}
FactBook.java
package com.stucowley.funfacts;
import java.util.Random;
public class FactBook {
// Fields (Member Variables) - Properties about the object
private String[] mFacts = {
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built."
};
// Methods - Actions the object can take
public String getFact() {
String fact;
// Randomly select a fact
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(mFacts.length);
fact = mFacts[randomNumber];
return fact;
}
}
And for good measure I will also include the activity_fun_facts.xml:
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="50dp"
android:paddingLeft="50dp"
android:paddingRight="50dp"
android:paddingTop="50dp"
android:id="@+id/relativeLayout"
android:background="#51b46d"
tools:context="com.stucowley.funfacts.FunFactsActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Did you know?"
android:textColor="#80ffffff"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintHorizontal_bias="0.062"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.03"
tools:text="Did you know?"
android:id="@+id/textView"/>
<TextView
android:id="@+id/factTextView"
android:layout_width="0dp"
android:layout_height="186dp"
android:layout_marginEnd="8dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="8dp"
android:layout_marginStart="8dp"
android:text="Ants stretch when they wake up in the morning."
android:gravity="center_vertical"
android:textColor="@android:color/white"
android:textSize="24sp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
tools:layout_constraintLeft_creator="1"
tools:layout_constraintRight_creator="1"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:layout_constraintTop_creator="1"
tools:layout_constraintBottom_creator="1"/>
<Button
android:id="@+id/showFactButton"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginBottom="16dp"
android:layout_marginEnd="8dp"
android:layout_marginLeft="8dp"
android:layout_marginRight="8dp"
android:layout_marginStart="8dp"
android:background="@android:color/white"
android:text="Show Another Fun Fact"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
tools:layout_constraintBottom_creator="1"
tools:layout_constraintLeft_creator="1"
tools:layout_constraintRight_creator="1" />
</android.support.constraint.ConstraintLayout>
I've also just discovered that I can see the logs from the emulator so I'll attach that too. I really want to get to the bottom of this, not just for myself but for others who may come across this in future.
03-30 17:35:36.625 4695-4695/? E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.stucowley.funfacts, PID: 4695
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.stucowley.funfacts/com.stucowley.funfacts.FunFactsActivity}: java.lang.ClassCastException: android.support.constraint.ConstraintLayout cannot be cast to android.widget.RelativeLayout
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2665)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)
Caused by: java.lang.ClassCastException: android.support.constraint.ConstraintLayout cannot be cast to android.widget.RelativeLayout
at com.stucowley.funfacts.FunFactsActivity.onCreate(FunFactsActivity.java:30)
at android.app.Activity.performCreate(Activity.java:6679)
at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1118)
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2618)
at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2726)
at android.app.ActivityThread.-wrap12(ActivityThread.java)
at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1477)
at android.os.Handler.dispatchMessage(Handler.java:102)
at android.os.Looper.loop(Looper.java:154)
at android.app.ActivityThread.main(ActivityThread.java:6119)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:886)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:776)
I will continue to see if I can figure this out but I'm at the stage where I need some help. No doubt this will be a user error.
Many thanks
Stu :)
3 Answers

Krahr Chaudhury
Courses Plus Student 139 PointsRead the errors:
java.lang.RuntimeException: Unable to start activity ComponentInfo{com.stucowley.funfacts/com.stucowley.funfacts.FunFactsActivity}: java.lang.ClassCastException: android.support.constraint.ConstraintLayout cannot be cast to android.widget.RelativeLayout
at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2665)
... blah blah blah
...
Caused by: java.lang.ClassCastException: android.support.constraint.ConstraintLayout cannot be cast to android.widget.RelativeLayout
at com.stucowley.funfacts.FunFactsActivity.onCreate(FunFactsActivity.java:30)
at android.app.Activity.performCreate(Activity.java:6679)
ClassCastException is caused when there's a type mismatch and you are trying to cast one object to an incompatible object type. In this instance, you are trying to cast something defined as a ConstraintLayout as RelativeLayout (I'm not an Android expert, but I'm guessing those are incompatible).
In activity_fun_facts.xml, the layout that has ID: relativeLayout is in fact defined as android.support.constraint.ConstraintLayout.
Try changing that to android.widget.RelativeLayout (although I think just RelativeLayout is fine).

Jeremy Conley
4,791 PointsOkay so the problem is that in your activity xml file you define your layout as a constraint layout, however in your fun facts activity you are defining a relative layout and trying to assign it to your constraint layout. Hence the error message - "Caused by: java.lang.ClassCastException: android.support.constraint.ConstraintLayout cannot be cast to android.widget.RelativeLayout" Either change the field in your activity to a Constraint Layout, or do the opposite in your xml and change to a relative layout.
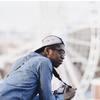
Jerome Heaven
8,641 PointsThanks Koha, You saved me from hours of troubleshooting.
Stu Cowley
26,287 PointsStu Cowley
26,287 PointsThank you so much Koha! All I needed to do was change the ConstraintLayout to RelativeLayout. I also needed to re-size and re-align the factTextView