Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial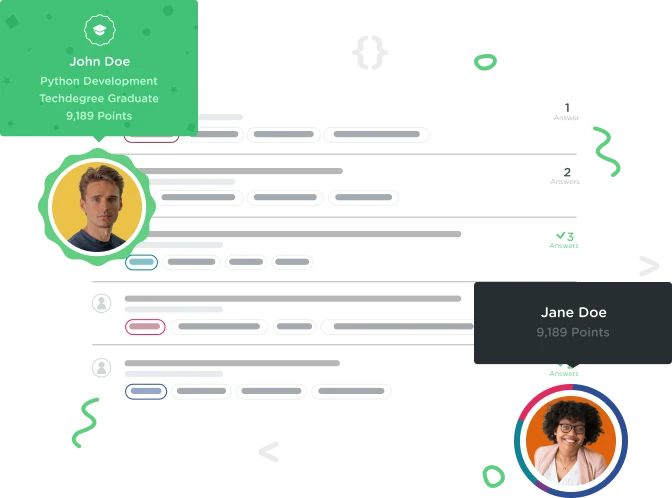
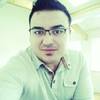
. Ali
9,799 PointsHmm thinking of DRY, Don't Repeat Yourself?
class Fish
{
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$output = "The {$this->common_name} is an awesome fish. ";
$output .= "It is very {$this->flavor} when eaten. ";
$output .= "Currently the world record {$this->common_name} weighed {$this->record_weight}.";
return $output;
}
}
class Trout extends Fish {
public $species;
function __construct ($name, $flavor, $record,$species){
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
public function getInfo (){
$output = "{$this->common_name} {$this->species} tastes {$this->flavor}.";
$output .= "The record {$this->common_name} {$this->species} weighed {$this->record_weight}";
return $output;
}
}
I am wondering that in the Trout class , which is extending the Fish class I had to reintroduce the variables in the constructor and also the getInfo( ) method needed me to put in all the information as from the parent class.. So in real world project would i need to copy all the info from the parent class ??? I dont feel like i am adhering to the DRY principle. Or is it me just being obnoxious
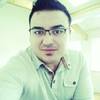
. Ali
9,799 Points Hi Leonardo,
Welcome to the world of OOP :) . Yes it does give an error which kinda makes sense as we adding an extra argument or should i say a new property in the child class as opposed to the the parent class
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
while in the child class we need to introduce an extra property
function __construct ($name, $flavor, $record,$species){
parent::__construct($name, $flavor, $record);
$this->species = $species;
}
The only thing i didn't get that if there is a way where PHP would allow us ( thinking logically not technically) to just append a new property to the already inherited properties of the parent class . Thanks for referring me to the documents but I guess at tree house they need to show us how to read the documentation properly as well i.e. PHP Docs ..
Oh well lets see :)
6 Answers
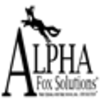
Shawn Gregory
Courses Plus Student 40,672 PointsHello Muhammad,
The way they have you reintroduce variables (which I don't necessarily believe is reintroducing the variables) is actually how you will be doing it in the real world. You are basically assigning the user input in the Fish class constructor and the class variables are accessible from the child class Trout. In order to assign the user input for the variables in the Fish class is by calling the parent class's constructor and you do that the same way you instantiate the class. This way actually prevents DRY as you are using the parent class's assigning instead of reassigning them inside your Trout class. Because all of the variables in the Fish class are public, the Trout class (Fish's child) can use them. I hope this explanation helped you understand that while it may look like you are doing double work, you are actually taking advantage of inheritance.
Cheers!
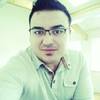
. Ali
9,799 PointsThanks Shawn it makes sense , the inheritance bit is quite clear now thinking about it as you have explained. My second question is that are we over riding the getInfo( ) method in the child class ???
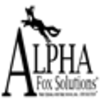
Shawn Gregory
Courses Plus Student 40,672 PointsHello,
Yes you are and for good reason. They both do the same hing except the one in the child class has the extra variable to display. There is no reason to use the parent's getInfo() function in the child class as it doesn't have the ability to use the new variable in the child class. Something that you will learn in OOP PHP is that with inheritance, it's only a one-way street. Children can inherit characteristics of its parent, but the parent class can't inherit anything from the child class. Hope this helps you understand better.
Cheers!
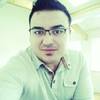
. Ali
9,799 Pointsthanks for your time shawn .. :) its a lot clearer now

Annie Scott
27,613 PointsInclude task 1 and 2 <?php
class Fish { public $common_name; public $flavor; public $record_weight;
TASK 2
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
}
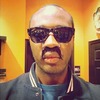
Elijah Collins
19,457 PointsThis might help
<?php
class Trout extends Fish {
function __construct($name, $flavor, $record) {
parent::__construct($name, $flavor, $record);
}
?>
Leonardo Hernandez
13,798 PointsLeonardo Hernandez
13,798 PointsI don't have an answer, but this interests me as well. I am new to OOP.
When you say that you had to reintroduce the variables in the constructor, did you get errors without introducing them again?
Check out the user submitted examples here: http://www.php.net/manual/en/keyword.extends.php
These might help. OH! I do not think that you are being obnoxious :)