Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial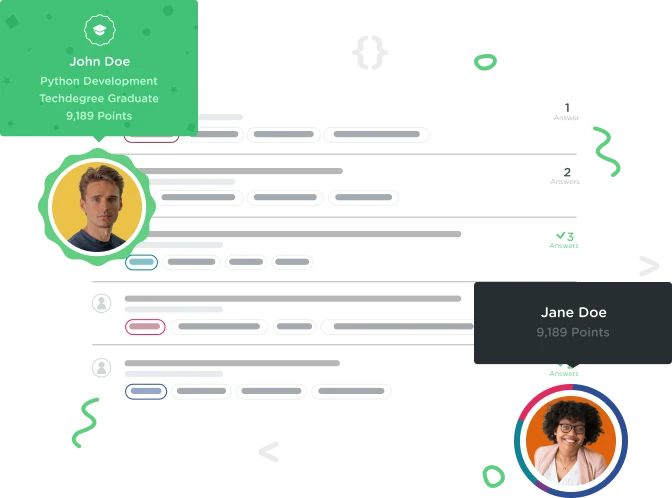
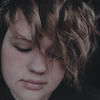
Ryan Maneo
4,342 PointsHmm... what am I missing?
What am I missing here?
class ViewController: UIViewController {
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}
let blueColor = UIColor(red: 0, green: 0, blue: 255, alpha: 1.0)
backgroundColor() = blueColor
2 Answers
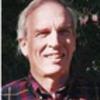
jcorum
71,830 PointsTwo problems. First, you code needs to be inside the viewDidLoad() method. Second, you need an object for the backgroundColor property, i.e., the view:
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
let blueColor = UIColor(red: 0, green: 0, blue: 255, alpha: 1.0)
view.backgroundColor = blueColor
}
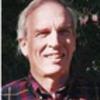
jcorum
71,830 PointsActually, there was a third problem. You can't have () after **backgroundColor*8, as it's not a method.
As to where to put things, my code was wrong (but accepted by the editor!!). The challenge asked for a stored property to the view controller (i.e., the ViewController class). So here's what I should have done:
class ViewController: UIViewController {
let blueColor = UIColor(red: 0, green: 0, blue: 255, alpha: 1.0) //Task 1 stored property
override func viewDidLoad() {
// Do any additional setup after loading the view, typically from a nib.
view.backgroundColor = blueColor //assign color to view's background //Task 2
}
override func didReceiveMemoryWarning() {
// Dispose of any resources that can be recreated
}
}
Re where to put things: stored properties go in the class (and usual practice is to put them first, above methods).
But the view.backgroundColor = . . . code can't go there because, in part, there's not yet a view object to assign a color to. So this code has to be in the viewDidLoad() method, i.e., in a method that doesn't run until after the view has loaded (and therefore exists and can be changed).
Ryan Maneo
4,342 PointsRyan Maneo
4,342 PointsI forgot view... dang... also how do I know where to put what? In this case, to put blueColor in the viewDidLoad method.