Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial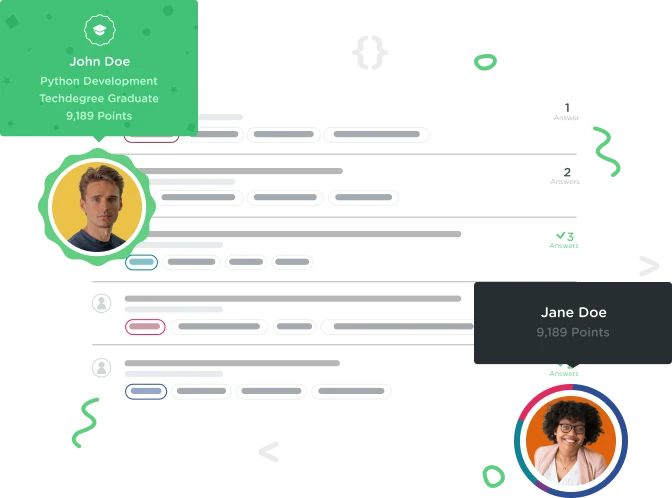

Abua KC
5,932 PointsHmmm, the tiles were "sreklahc" and I passed in 'r' expected 1, but I got 114
I am trying to increment a counter every time tiles match with the letter. I also need to have it return a number representing count of tiles that match the letter that was passed. Its not passing correctly, What do I do ? thanks
public class ScrabblePlayer { // A String representing all of the tiles that this player has private String tiles;
public ScrabblePlayer() { tiles = ""; }
public String getTiles() { return tiles; }
public void addTile(char tile) { tiles += tile; }
public boolean hasTile(char tile) { return tiles.indexOf(tile) != -1; } public int getCountOfLetter(char letter){ int count = 0;
for (char c : tiles.toCharArray()){
c++;
if ( c == tiles.length()){ // letter matches the tiles , then increment
count++;
return getCountOfLetter(letter); //
}
}
return letter;
} }
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter){
int count = 0;
for (char c : tiles.toCharArray()){
c++;
if ( c == tiles.length()){
count++;
return getCountOfLetter(letter);
}
}
return letter;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers
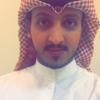
Fahad Mutair
10,359 Pointshi Abua Odumefune ,
public int getCountOfLetter(char letter){
int count = 0;
for (char c : tiles.toCharArray()){
you did great with those lines
since your c variable is char why do u put ++ to it? its not integer . so remove this line we dont need it
c++;
in your if statement you have to check is your variable c (char) equals to char letter
if ( c == tiles.length()){ // change this line
if ( c == letter){ // to this line
Now inside if statement body we need to increment count and we don't have to return anything so we gonna remove the return statement
count++;
return getCountOfLetter(letter); // remove this line
}
last thing your method need to return int variable not char so
}
return letter; // change this
return count; // to this line
}
so final code
public int getCountOfLetter(char letter){
int count = 0;
for (char c : tiles.toCharArray()){
if ( c == letter){
count++;
}
}
return count;
}

Abua KC
5,932 PointsHello Fahad Mutair,
You are a life saver. Thanks