Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial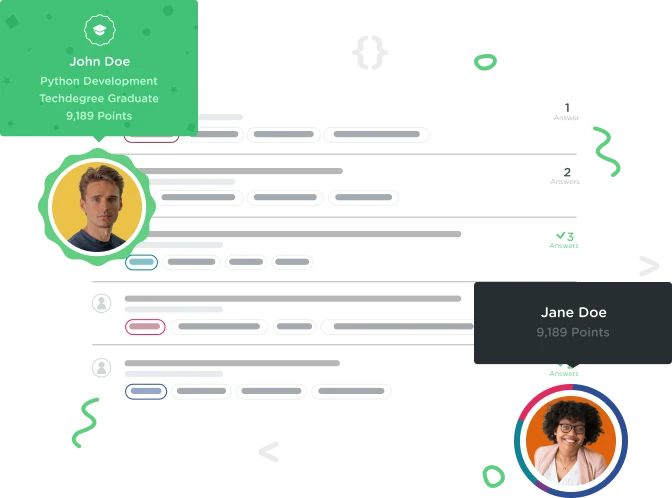
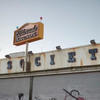
Vic A
5,452 Pointshonestly, i don't know where to begin on this one.
need help.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(*args):
for x in (*args):
3 Answers
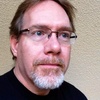
Chris Freeman
Treehouse Moderator 68,423 PointsFirst, there will be two arguments, so you can explicitly use def combo(arg1, arg2)
or something similar.
One approach would be to
- loop over the length of one input argument
- use the next element of each argument to create a tuple
- append that tuple to the list that can be returned when done
- return created list
Post back if you have questions. Good luck!!!
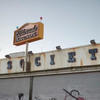
Vic A
5,452 Pointsthanks for the help. I was successfully with this code, but I know it the scenic route to solving it. Do you know a more concise way of writing it?
def combo(x, y):
combol = []
w = 0
for i in range(len(x)):
xin = x[w]
yin = y[w]
combol.append((xin, yin))
w += 1
return combol
thanks
[MOD: added ```python formatting -cf]
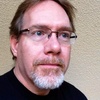
Chris Freeman
Treehouse Moderator 68,423 Points# first, realize w equals i so use i instead:
def combo(x, y):
combol = []
for i in range(len(x)):
xin = x[i]
yin = y[i]
combol.append((xin, yin))
return combol
# next, can used index item directly
def combo(x, y):
combol = []
for i in range(len(x)):
combol.append((x[i], y[i]))
return combol
Otherwise, you could also use a list comprehension or the built-in function zip
# list comprehension
def combo(x, y):
return [(x[i], y[i]) for i in range(len(x))]
# Using zip() [no longer acceptable answer]
def combo(x, y):
return list(zip(x, y))
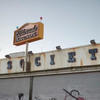
Vic A
5,452 Pointsawesome thanks.
Evan Huddleson
12,623 PointsChris, can you explain step by step exactly what is being executed in the # list comprehension?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsMoving these examples under posted answer:
Starting with Vic A's solution, this is how I'd improve it.
Otherwise, you could also use a list comprehension or the built-in function
zip
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsAdding detail of the list comprehension solution:
I read the list comprehension as Generate a list of items where
(a, b)
.a
" is replaced withx[i]
and "b
" is replaced withy[i]
.i
" is taken from thefor
loop wherei
is each value in the range from0
to thelen(x)
.range
generator stops one short of the last element, so effectively the range is [0 to len(x) -1].So, take the index values, i, from 0 to
len(x) - 1
, to build a list of tuples consisting of the ith elements fromx
andy
containers