Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial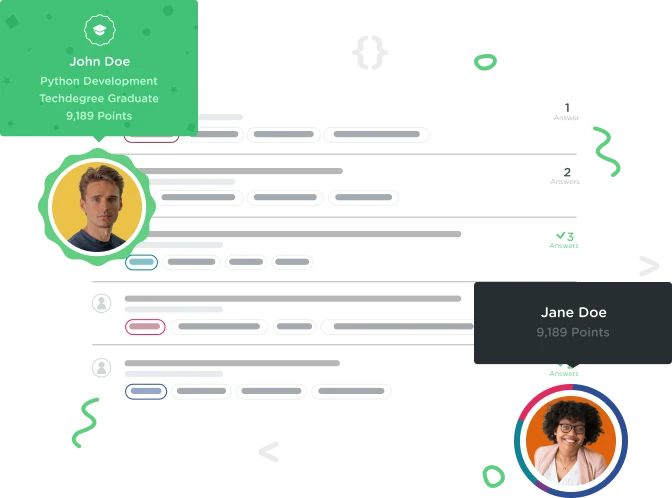

Mendy Berrebi
1,856 PointsHour, Minutes, Seconds
Below is my code: I don't want ruby to round up my minutes when I divide by 60. How do I come about this?
x is the speed of the car (in mph) and y is the distance (in miles) z is the time (in hours)
print "Enter distance(in miles): " d = gets.chomp.to_i print "Enter Hours: " h = gets.chomp.to_i print "Enter Minutes: " m = (gets.chomp.to_i % 60)
x = d / (h + m)
if (d >= 0) && (h) && (m % 60) puts "In (#{d} miles), you will arrive to your destination in #{h} hours and #{m} minutes if you have a speed of approximately #{x}mph" end
1 Answer
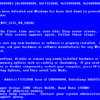
Daniel Powell
23,321 PointsIf Ruby encounters an equation that only uses integers, it will assume you want your answer to also be an integer. This can lead to some odd rounding errors if you aren't careful. A really basic example that demonstrates this:
puts 10 / 3 -> 3
puts 10.0 / 3 -> 3.3333333333333335
To avoid this, make sure you are working with floating point numbers when performing calculations. You can then round your results to easier to read numbers when displaying them for the end user.
I also see your code has some math issues that might be leading to the unexpected results you are seeing. You'd probably get better results in this instance by avoiding the mod operator completely and converting all time-related input to similar units. You could try approaching the problem more like this:
print "Enter distance (in miles): "
distance = gets.chomp.to_f #Converts input into a floating point number
print "Enter Hours: "
hours = gets.chomp.to_f
print "Enter minutes: "
minutes = gets.chomp.to_f
travel_time = ((hours * 60) + minutes) / 60 #Converts all user input into a singular unit type.
speed = distance / travel_time #Standard speed formula
if (distance >= 0) && (hours >= 0) && (minutes >= 0)
puts "In #{distance} miles, you will arrive at your destination in #{hours.to_i} hours and #{minutes.to_i} minutes if you maintain a speed of approximately #{speed.round(2)}mph"
end
Mendy Berrebi
1,856 PointsMendy Berrebi
1,856 PointsThank you so much Daniel!! Much appreciated!
Alphonse Cuccurullo
2,513 PointsAlphonse Cuccurullo
2,513 PointsMind i ask what that extra perentacy is for before the travel_times hours?
Daniel Powell
23,321 PointsDaniel Powell
23,321 PointsAre you asking about this bit of code?
travel_time = ((hours * 60) + minutes) / 60
If so, this is just a way I like to write out calculations. Nesting the statements in parentheses, although not always necessary, makes the order of operations explicit and easier to read (at least I think so). Each set of parentheses is one step in the calculation. This way I can ensure (hours * 60) is calculated before being added to the minutes variable. If I wanted to be especially consistent, I could have even written the entire line of code like this:
travel_time = (((hours * 60) + minutes) / 60)
Also, since nearly all modern programming languages work from the inner set of parentheses to the outer sets, I can ensure/force my own order of the operations (PEMDAS be damned).
In the end, this is just a style of coding I like. I try write very explicit and clear code and this contributes to that goal. I think this style communicates my intent more clearly.
I hope that answers your question.