Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial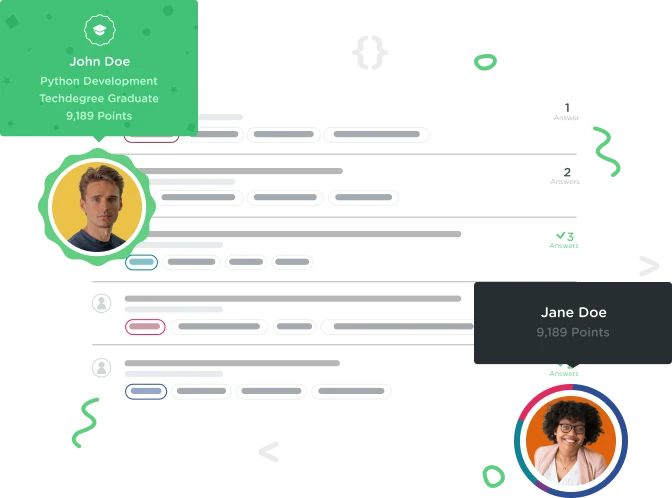
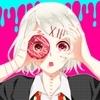
Ronnelle Torres
5,958 PointsHow about adding a eraser?
The drawing app is really good. Though, it would be awesome if we can add an eraser. ANy one up for the challenge?
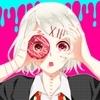
Ronnelle Torres
5,958 PointsHere it is Steven :)
I used sublime text (created a new project so that I can also review it) that's why it's a single document. Here it is :)
<!DOCTYPE html>
<html>
<title>Drawing board</title>
<meta charset="utf-8">
<style>
* {
box-siing: border-box;
}
body {
text-align:center;
font-family:sans-serif;
}
canvas {
background-color:black;
margin-top:20px;
border-radius:10px;
}
ul {
list-style-type:none;
padding:0;
}
.controls li {
width:50px;
height:50px;
border-radius:100%;
display:inline-block;
margin:0 5px;
cursor:pointer;
}
.red {
background-color:firebrick;
}
.yellow {
background-color:yellow;
}
.blue {
background-color:turquoise;
}
#revealColor {
display:none;
text-align:center;
width:400px;
margin:20px auto;
background:#333;
padding:10px;
position:relative;
}
#newColor {
margin: 40px 20px 20px 10px;
width:80px;
height:80px;
display:inline-block;
border-radius:10px;
border:2px solid white;
}
#colorRange{
display:inline-block;
}
#colorRange p {
vertical-align: middle;
}
.selected {
box-shadow:inset 0 0 0 5px rgba(0,0,0, .5);
}
label {
vertical-align:super;
display: inline-block;
margin: 0 10px 0 0;
width: 40px;
font-size: 14px;
color: white;
}
#addColor {
display:block;
margin:0 auto;
}
button {
cursor:pointer;
}
.eraser {
border:2px solid white;
background:transparent;
padding:5px;
border-radius:5px;
cursor:pointer;
color:white;
}
</style>
<body>
<canvas width="300" height="175"></canvas>
<div class="controls">
<ul>
<li class="red selected"> </li>
<li class="yellow"> </li>
<li class="blue"> </li>
</ul>
<button id="showDashboard">Add More Color </button>
<div id="revealColor">
<span id="newColor"> </span>
<div id="colorRange">
<p>
<label for="red">RED</label>
<input id="red" name="red" type="range" min="0" max="255" value="0">
</p>
<p>
<label for="green">GREEN</label>
<input id="green" name="green" type="range" min="0" max="255" value="0">
</p>
<p>
<label for="blue">BLUE</label>
<input id="blue" name="blue" type="range" min="0" max="255" value="0">
</p>
</div>
<button id="addColor">add Color</button>
<button id="eraser">Eraser on </button>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.1.0.min.js" integrity="sha256-cCueBR6CsyA4/9szpPfrX3s49M9vUU5BgtiJj06wt/s=" crossorigin="anonymous"></script>
<script>
var color = $(".selected").css("background-color");
var $context = $("canvas")[0].getContext("2d");
var $canvas = $("canvas");
var lastPoint;
var mouseDown = false;
$(".controls").on("click", "li", function(){
$(this).siblings("li").removeClass("selected");
$(this).addClass("selected");
color = $(this).css("background-color");
});
$("#showDashboard").click(function(){
$("#revealColor").slideToggle("slow");
$("body").animate({scrollTop: 700}, 1500);
getColor();
});
function getColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
var rgb = "rgb(" + r + "," + g + "," + b + ")";
$("#newColor").css("background-color", rgb);
};
$("input[type=range]").on("input", function(){
getColor();
});
$("#addColor").click(function(){
var newList = $("<li></li>");
var spanColor = $("#newColor").css("background-color");
newList.css("background-color", spanColor);
$(".controls ul").append(newList);
newList.click();
});
$("#eraser").click(function(){
$(this).toggleClass("eraser");
});
function isEraserOn(){
return $("#eraser").hasClass("eraser");
};
var $canErase = false;
$canvas.mousedown(function(e){
lastPoint = e;
if (isEraserOn()) {
$context.clearRect(lastPoint.offsetX, lastPoint.offsetY, 20, 20);
$canErase = true;
mouseDown = false;
} else {
mouseDown = true;
$canErase = false;
};
}).mousemove(function(e){
if(mouseDown && !$canErase){
$context.beginPath();
$context.moveTo(lastPoint.offsetX, lastPoint.offsetY);
$context.lineTo(e.offsetX, e.offsetY);
$context.strokeStyle = color;
$context.lineWidth = 5;
$context.stroke();
lastPoint = e;
} else if (!mouseDown && $canErase){
$context.moveTo(lastPoint.offsetX, lastPoint.offsetY);
$context.clearRect(e.offsetX, e.offsetY, 20, 20);
lastPoint = e;
}
}).mouseup(function(){
mouseDown = false;
$canErase = false;
}).mouseleave(function(){
$canvas.mouseup();
});
</script>
</body>
</html>
2 Answers
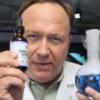
Justin Coen
Full Stack JavaScript Techdegree Student 12,829 PointsThis is a quick solution I threw together - with bugs and all - of my take on an eraser.
SnapShot: https://w.trhou.se/x0ejqwt2at
I used a simple solution of setting the eraser to whichever color the canvas' background-color is, a bit of a work around but it can still get the job done :)
Once the eraser is selected a boolean is set to true and the canvas' lineWidth is increased to 10 to make erasing a bit easier.
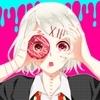
Ronnelle Torres
5,958 PointsNIce :). I was also able to do it but using a different method. I used sublime text (created a new project so that I can also review it) that's why it's a single document. Here it is :)
<!DOCTYPE html>
<html>
<title>Drawing board</title>
<meta charset="utf-8">
<style>
* {
box-siing: border-box;
}
body {
text-align:center;
font-family:sans-serif;
}
canvas {
background-color:black;
margin-top:20px;
border-radius:10px;
}
ul {
list-style-type:none;
padding:0;
}
.controls li {
width:50px;
height:50px;
border-radius:100%;
display:inline-block;
margin:0 5px;
cursor:pointer;
}
.red {
background-color:firebrick;
}
.yellow {
background-color:yellow;
}
.blue {
background-color:turquoise;
}
#revealColor {
display:none;
text-align:center;
width:400px;
margin:20px auto;
background:#333;
padding:10px;
position:relative;
}
#newColor {
margin: 40px 20px 20px 10px;
width:80px;
height:80px;
display:inline-block;
border-radius:10px;
border:2px solid white;
}
#colorRange{
display:inline-block;
}
#colorRange p {
vertical-align: middle;
}
.selected {
box-shadow:inset 0 0 0 5px rgba(0,0,0, .5);
}
label {
vertical-align:super;
display: inline-block;
margin: 0 10px 0 0;
width: 40px;
font-size: 14px;
color: white;
}
#addColor {
display:block;
margin:0 auto;
}
button {
cursor:pointer;
}
.eraser {
border:2px solid white;
background:transparent;
padding:5px;
border-radius:5px;
cursor:pointer;
color:white;
}
</style>
<body>
<canvas width="300" height="175"></canvas>
<div class="controls">
<ul>
<li class="red selected"> </li>
<li class="yellow"> </li>
<li class="blue"> </li>
</ul>
<button id="showDashboard">Add More Color </button>
<div id="revealColor">
<span id="newColor"> </span>
<div id="colorRange">
<p>
<label for="red">RED</label>
<input id="red" name="red" type="range" min="0" max="255" value="0">
</p>
<p>
<label for="green">GREEN</label>
<input id="green" name="green" type="range" min="0" max="255" value="0">
</p>
<p>
<label for="blue">BLUE</label>
<input id="blue" name="blue" type="range" min="0" max="255" value="0">
</p>
</div>
<button id="addColor">add Color</button>
<button id="eraser">Eraser on </button>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.1.0.min.js" integrity="sha256-cCueBR6CsyA4/9szpPfrX3s49M9vUU5BgtiJj06wt/s=" crossorigin="anonymous"></script>
<script>
var color = $(".selected").css("background-color");
var $context = $("canvas")[0].getContext("2d");
var $canvas = $("canvas");
var lastPoint;
var mouseDown = false;
$(".controls").on("click", "li", function(){
$(this).siblings("li").removeClass("selected");
$(this).addClass("selected");
color = $(this).css("background-color");
});
$("#showDashboard").click(function(){
$("#revealColor").slideToggle("slow");
$("body").animate({scrollTop: 700}, 1500);
getColor();
});
function getColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
var rgb = "rgb(" + r + "," + g + "," + b + ")";
$("#newColor").css("background-color", rgb);
};
$("input[type=range]").on("input", function(){
getColor();
});
$("#addColor").click(function(){
var newList = $("<li></li>");
var spanColor = $("#newColor").css("background-color");
newList.css("background-color", spanColor);
$(".controls ul").append(newList);
newList.click();
});
$("#eraser").click(function(){
$(this).toggleClass("eraser");
});
function isEraserOn(){
return $("#eraser").hasClass("eraser");
};
var $canErase = false;
$canvas.mousedown(function(e){
lastPoint = e;
if (isEraserOn()) {
$context.clearRect(lastPoint.offsetX, lastPoint.offsetY, 20, 20);
$canErase = true;
mouseDown = false;
} else {
mouseDown = true;
$canErase = false;
};
}).mousemove(function(e){
if(mouseDown && !$canErase){
$context.beginPath();
$context.moveTo(lastPoint.offsetX, lastPoint.offsetY);
$context.lineTo(e.offsetX, e.offsetY);
$context.strokeStyle = color;
$context.lineWidth = 5;
$context.stroke();
lastPoint = e;
} else if (!mouseDown && $canErase){
$context.moveTo(lastPoint.offsetX, lastPoint.offsetY);
$context.clearRect(e.offsetX, e.offsetY, 20, 20);
lastPoint = e;
}
}).mouseup(function(){
mouseDown = false;
$canErase = false;
}).mouseleave(function(){
$canvas.mouseup();
});
</script>
</body>
</html>

jennyhan
36,121 PointsWithout adding an element in HTML, you can still set an eraser by just adding a white color with context.linewidth border nearby the color list.
Steven Parker
231,275 PointsSteven Parker
231,275 PointsThat sounds like a good extra-practice project.
You could do it and then show us your revised code.