Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial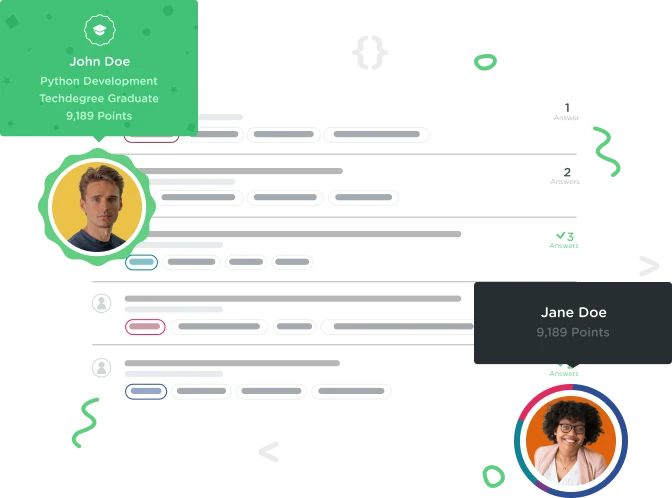

Ricard Taujenis
4,383 PointsHow about making a new test that does the same, Arrange, Act and Assert named refundingResetsAvailableFundsToZero that s
Ive been changing the @Test method multiple times the previous video didnt show a lot of insight for me how to make the solution im still quite new in Java.
package com.teamtreehouse.vending;
import org.junit.Test;
import static org.junit.Assert.*;
public class CreditorTest {
@Test
public void addingFundsIncrementsAvailableFunds() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(25);
creditor.addFunds(25);
assertEquals(50, creditor.getAvailableFunds());
}
@Test
public void refundingReturnsAllAvailableFunds() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(10);
int refund = creditor.refund();
assertEquals(10, refund);
assertEquals(0, creditor.getAvailableFunds());
}
}
@Test
public void refundingReturnsAllAvailableFundsToZero() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(0);
int refund = creditor.refund();
assertEquals(0, refund);
assertEquals(0, creditor.getAvailableFundsToZero());
}
}
package com.teamtreehouse.vending;
public class Creditor {
private int funds;
public Creditor() {
funds = 0;
}
public void addFunds(int money) {
funds += money;
}
public void deduct(int money) throws NotEnoughFundsException {
if (money > funds) {
throw new NotEnoughFundsException();
}
funds -= money;
}
public int refund() {
int refund = funds;
funds = 0;
return refund;
}
public int getAvailableFunds() {
return funds;
}
}
1 Answer
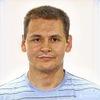
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsFirst of all there is no getAvailableFundsToZero()
method in Creditor
class...
assertEquals(0, creditor.getAvailableFundsToZero()); // no such method exists
There is only getAvailableFunds()
function
Second, all you need to do is to move asserting line
assertEquals(0, creditor.getAvailableFunds());
refundingReturnsAllAvailableFunds()
into to the new test.
This way we'll make one assertion per test if possible as Craig wants
In your test there are still two assertions.
Let me write you a hint with sudo code:
@Test
public void refundingReturnsAllAvailableFundsToZero() throws Exception {
Creditor creditor = new Creditor(); // correct
creditor.addFunds(0); // correct
int refund = creditor.refund(); // correct
// wrong, because we already asserted it,we want one assertion per test
assertEquals(0, refund);
// change name of the method, it is wrong, see above
assertEquals(0, creditor.getAvailableFundsToZero());
}
Ricard Taujenis
4,383 PointsRicard Taujenis
4,383 Points@Test public void refundingReturnsAllAvailableFundsToZero() throws Exception { Creditor creditor = new Creditor(); creditor.addFunds(0);
Added this one still didnt pass, btw is it possible to learn proper Java without a book?
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsAlexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsJoin Techdegree! :) I learned a lot of Java and never felt sorry about it.
About the code challenge. I made a video with direct posting of your code. It works.
Take a look
https://www.youtube.com/watch?v=2Dtn-7On3os