Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial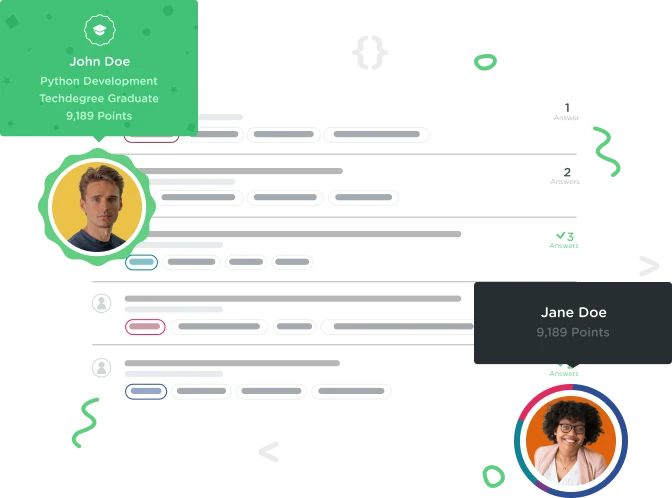

Seth Roope
6,471 PointsHow about this for a solution?
func fizzBuzz(n: Int) -> String {
if (n%3 != 0) && (n%5 != 0) {
return "\(n)"
}
var i = " "
if (n % 3 == 0) {
i += "Fizz"
}
if (n % 5 == 0) {
i += "Buzz"
}
return "\(i)"
}
for k in 1...100{
fizzBuzz(n: k)
}
9 Answers
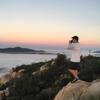
Chase Carnaroli
5,139 PointsHere's how I do it. Your solution is pretty similar to mine! Personally, I think it makes more sense to create a solution using string concatenation. It's a bit cleaner and more efficient.
func fizzBuzz(n: Int) -> String {
// output message if it is a FizzBuzz
var message = ""
// test to see if it is a FizzBuzz
if (n % 3 == 0) {
message += "Fizz"
}
if (n % 5 == 0) {
message += "Buzz"
}
// if message is still empty, return the number
// otherwise return the message
if (message == ""){
return "\(n)"
} else {
return message
}
}
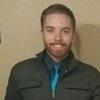
Matthew Timmons
33,804 PointsThis is a great answer and how I did it, but if you want to take it one step further, you don't even need the else block at the end.
So this:
if (message == ""){
return "\(n)"
} else {
return message
}
can be abbreviated to this:
if (message == ""){
return "\(n)"
}
return message

Mohammad Laif
Courses Plus Student 22,297 PointsSimple and clear way
Since we are inside a function, we can use the help of "return" keyword as a break. And dealing with % 15 as first condition, so %3 and %5 don't clash with each other! Finally if all conditions fails (ifs) then return the number as string
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n % 15 == 0){return "FizzBuzz"}
if (n % 3 == 0){return "Fizz"}
if (n % 5 == 0){return "Buzz"}
// End code
return "\(n)"
}
For testing
// for testing
for i in 1...100{
print(fizzBuzz(n: i))
}

Matthew Foster
7,442 PointsI've done this challenge in many languages and I don't know why I've never thought of this version. I always do the "build the output string" but this is so much cleaner.

Dean Kreseski
2,418 PointsGreat answer, clear and simple way of looking at it.

Thomas Dobson
7,511 Pointsfunc fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch (n % 3, n % 5)
{
case (0, 0): return "FizzBuzz"
case(0, _): return "Fizz"
case(_, 0): return "Buzz"
default: return "\(n)"
}
// End code
return "\(n)"
}

Seth Roope
6,471 PointsDid you not see that someone already posted the same solution? Furthermore, I am not asking what your solution is, I am trying to see what people think of trying to create a solution using string concatenation where the function returns either "fizz" or "buzz" or both, never returning "fizzbuzz"

The Duka
750 Pointssuper awesome

Alex Busu
Courses Plus Student 11,819 PointsGuys the simplest solution
func fizzBuzz(n: Int) -> String {
var result=""
if (n%3==0) { result+="Fizz" }
if (n%5==0) { result+="Buzz" }
return result.count>0 ? result : "\(n)"
}
and an even shorter answer
func fizzBuzz(n: Int) -> String {
var r = (n%3==0) ? "Fizz" : ""
if (n%5==0) { r+="Buzz" }
return r.count>0 ? r : "\(n)"
}
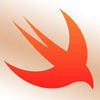
Jeff McDivitt
23,970 Pointsfunc fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
if (n % 3 == 0 && n % 5 == 0) {
return "FizzBuzz"
}
else if (n % 3 == 0) {
return "Fizz"
}
else if (n % 5 == 0) {
return "Buzz"
}
// End code
return "\(n)"
}

Seth Roope
6,471 Pointsummm thanks...
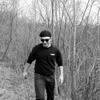
Youssef Girgeis
iOS Development Techdegree Student 10,996 Pointslet randonNumber = GKRandomSource.sharedRandom().nextInt(upperBound: 100)
switch (randonNumber % 3, randonNumber % 5){
case (0, 0): print("Fizz Buzz")
case(0, _): print("Fizz")
case(_, 0): print("Buzz")
default: print(randonNumber)
}

Anthony Attard
43,915 PointsI like this solution, even though Switch Case is probably a too elaborate solution for a simple task.

Seth Roope
6,471 PointsI like this one too Anthony Attard. I do not see any reason a switch would be 'too elaborate' for any task no matter how simple.
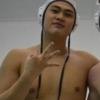
David Jeong
11,155 Pointsyour var i is starting with a space!
func fizzBuzz(n: Int) -> String {
if (n%3 != 0) && (n%5 != 0) {
return "\(n)"
}
// change here
var i = ""
if (n % 3 == 0) {
i += "Fizz"
}
if (n % 5 == 0) {
i += "Buzz"
}
return "\(i)"
}
for k in 1...100{
fizzBuzz(n: k)
}
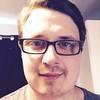
Lewis Morgans
3,167 Pointsfunc fizzBuzz(n: Int) -> String {
if n % 3 == 0 && n % 5 == 0 {
return "Fizz Buzz"
}
else if n % 5 == 0 {
return "Buzz"
}
else if n % 3 == 0 {
return "Fizz"
}
return "(n)" }

Jon Mendelson
10,897 PointsNot going to retype my entire solution, but what I wound up doing is skipping hard-coding the && part, and simply doing
"if n % 15 == 0 return fizzbuzz"
then doing the individual checks for 3 and 5
Seth Roope
6,471 PointsSeth Roope
6,471 PointsThis works although it doesn't pass the test in the built in code editor. Is there an even simpler way to do this? Something more like this that will work perhaps...?