Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial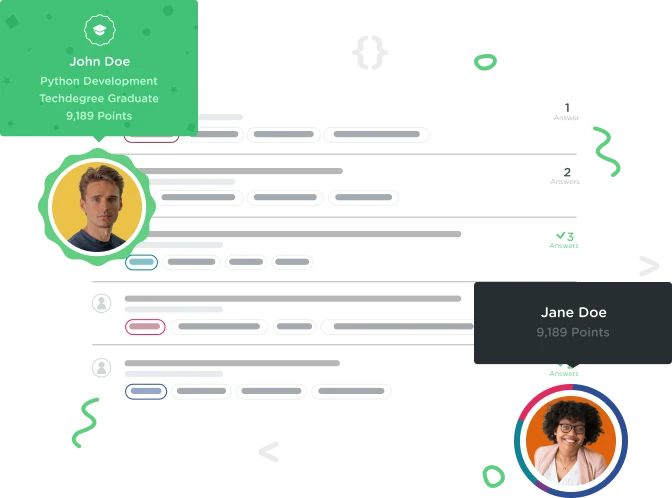
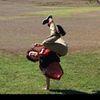
Koki Tanaka
3,839 PointsHow about using [Any] type instead of generic types?
I use Xcode ver8.0.
Gabe said we can use a generic type in the class “class StatGroup” but just for a try, I used Any type in the property “member” as below:
import UIKit
struct Team {
var name: String
var city: String
var winPCT: Double = 0.0
init(name: String, city: String){
self.name = name
self.city = city
}
}
struct Player {
var name: String
var height: Double
init(name: String, height: Double) {
self.name = name
self.height = height
}
}
class StatGroup {
var members = [Any]()
var title: String
init(title: String, members: [Any]) {
self.title = title
self.members = members
}
}
var blazers = Team(name: "Blazers", city: "Portland")
var nuggets = Team(name: "Nuggets", city: "Denver")
var hoopsUS = StatGroup(title: "NBA West", members: [blazers, nuggets])
hoopsUS.members
var star = Player(name: "Pasan", height: 88)
var benchwarmer = Player(name: "Andrew", height: 66)
var iceFencingUS = StatGroup(title: "Olympic Ice Fencing(US)", members: [star, benchwarmer])
iceFencingUS.members
I think it worked exactly the same as the code in this lesson. It would be great if you could tell me what’s the difference.
1 Answer
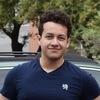
James Estrada
Full Stack JavaScript Techdegree Student 25,866 PointsYou use Generics for Type Safety. If you use "Any" the compiler will not warn you of any possible mistake that you might do in declaring and using different types in your objects (e.g passing a Double but using a String) and your app will crash during runtime. Check this out for more information.