Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial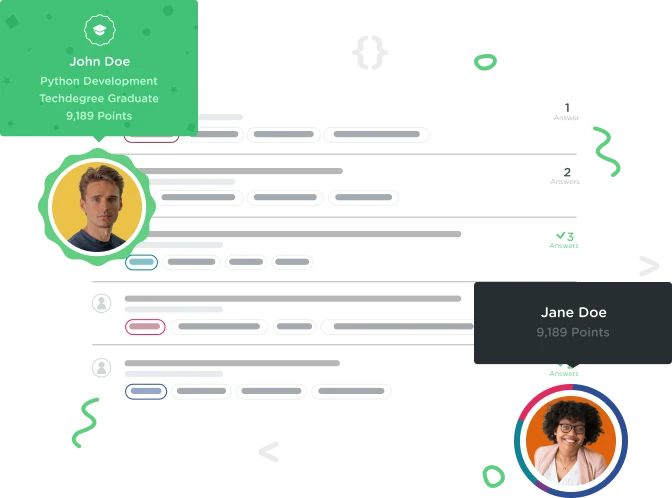

Ned Grossy
Courses Plus Student 3,292 PointsHow add own functionality to standard Python function ?
Hello everyone.
I have watched a lecture about how to remove items from a list and decided to add own keyword optional argument to remove all instances of specified argument from a list.
For example:
a = [1, 2, 3, 1] a.remove(1) # a = [2, 3, 1]
I want: a = [1, 2, 3, 1] a.remove(1, all = True) # a = [2, 3]
I saw many solutions how we can do this in Pythonic way using list comprehension and so on. But for education purpose I want to now how to add something in Python.
So the question is: what the next step ?
I have downloaded and compiled Python. Can somebody help me with the next step ? To my mind this is quite interesting task.
Many thanks!
3 Answers
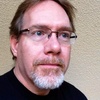
Chris Freeman
Treehouse Moderator 68,457 PointsThe short answer is: No. Types defined in C cannot be monkeypatched. Trying to overwrite the list.remove
method lead to this error:
In [62]: list.remove = newremove
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
/home/chrisf/devel/pydx-volunteer-2015/pydx_volunteer/<ipython console> in <module>()
TypeError: can't set attributes of built-in/extension type 'list'
This is discussed more on StackOverflow. This SO answer does give a possibility using pypy/forbiddenfruit
module.
A better solution would be to create your own list class that has your version of remove:
class MyList(list):
'''Create new List class with altered remove method'''
def remove(self, item):
'''Remove all instances of item from list'''
# "self" is the list
# create new self using list comprehension omitting item
self[:] = [x for x in self if x != item]
Using it:
In [67]: lst = MyList([3, 4, 5, 6, 3, 4, 1, 8, 9, 3, 7, 1, 3])
In [68]: lst
Out[68]: [3, 4, 5, 6, 3, 4, 1, 8, 9, 3, 7, 1, 3]
In [69]: lst.remove(1)
In [70]: lst
Out[70]: [3, 4, 5, 6, 3, 4, 8, 9, 3, 7, 3]
In [71]: lst.remove(3)
In [72]: lst
Out[72]: [4, 5, 6, 4, 8, 9, 7]
# Changing regular list to MyList:
In [73]: lst2 = [1, 0, 1, 0, 1, 0]
In [74]: lst2 = MyList(lst2)
In [75]: lst2
Out[75]: [1, 0, 1, 0, 1, 0]
In [76]: lst2.remove(0)
In [77]: lst2
Out[77]: [1, 1, 1]

Ned Grossy
Courses Plus Student 3,292 PointsYeah, definitely, it is best way to start. Many thanks!

Ned Grossy
Courses Plus Student 3,292 PointsSo,
seems like I did it.
a = [1, 2, 3, 1] a.remove(1, all = True) a # [2, 3]
Looks more attractive than
a = [1, 2, 3, 1] a[:] = (item for item in a if item != 1)
Or especially than another non-Pythonic solutions.
Is it?
Ned Grossy
Courses Plus Student 3,292 PointsNed Grossy
Courses Plus Student 3,292 PointsI mean how to start edit CPythonβs source code. I want to add this feature into Python core.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsAh, that's a different beast entirely. There is the Python Developers Guide which details how to work on the core code development.