Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial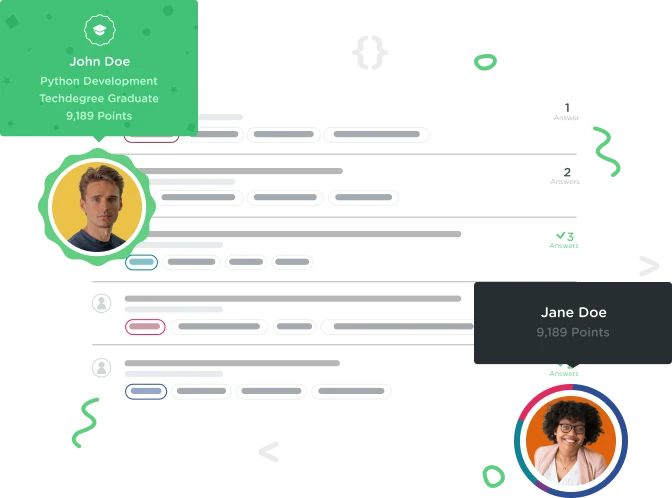
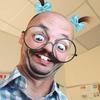
Van Sanders
16,087 PointsHow am I not setting the private fields correctly here?
In step 2 of the "Wrapping Up" exercise I keep getting an error indicating that the way I'm setting the private fields is incorrect or out of place.
Directly under the note "//TODO: (...)" I have:
private String firstName; private String lastName;
I haven't written the getters yet. I don't know if that would have an impact on the syntax checker, but I thought that they could be declared and just sit there without any issue, even if there is no getter to call upon them.
I'm sure I'm making a goofy oversight. Any help would be greatly appreciated.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
public User(String firstName, String lastName) {
// TODO: Set the private fields here
private String firstName;
private String lastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
8 Answers
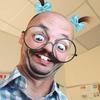
Van Sanders
16,087 PointsI found this elsewhere in the forums. The way I have it set above is indeed problematic because the
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
are pointing to something that isn't declared/set in the constructor itself.
Thanks for all of the help guys.

Craig Dennis
Treehouse TeacherLooks like you didn't add the constructor in ForumPost
.
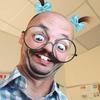
Van Sanders
16,087 PointsThanks guys! That did the trick. I'm now working on "java.lang.NullPointerException (Look around Forum.java line 14)"
I know I'll get it!

ISAIAH S
1,409 Points'Forum.java
line 14' says 'When all is ready uncomment this...'. Try uncommenting it.
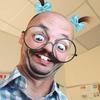
Van Sanders
16,087 PointsHaha thanks, I made sure to uncomment that code first. Still getting the error though. Its ok, I'll keep on it.

ISAIAH S
1,409 PointsDid you erase 'When all is ready uncomment this...', because that will give you errors.
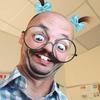
Van Sanders
16,087 PointsI did indeed. It seems like it is not recognizing "post", but I see in Example.java where it is created. I have a hunch that the error might have to do with what is going on in Example.java, but I'm not sure yet. Still coming back to it as time allows. Thanks all for the generous support!
My code from Forum.java is as follows (I actually deleted that comment line and as a result the null error now points me to line 13):
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
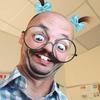
Van Sanders
16,087 PointsI get the feeling that I'm making progress now that my error messages appear to be canned responses anticipating the error that I made :) Thank you for not just giving this away. I started playing with the code in Forum.java and landed on this:
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s about %s.\n",
post.getAuthor(),
post.getAuthor(),
post.getTitle());
}
}
with a new and taunting error: "I expected to see the names I passed in through the args, but I do not. Hmm."
I feel like I'm following the crumbs. Again, thanks for all of the help!

ISAIAH S
1,409 PointsErase the second 'post.getAuthor(),
'.
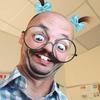
Van Sanders
16,087 PointsI tried that earlier but it gives me the same error quoted above.

ISAIAH S
1,409 PointsI think I know what it means by "I expected to see the names I passed in through the args, but I do not. Hmm." Note 'names' not just name. So I think you should keep
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
See that you don't have .getFirstName()
and .getLastName()
? You just have .getAuthor()
.
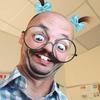
Van Sanders
16,087 PointsThanks, I changed it back to how it was, but still get the "NullPointer" error.
I wonder if my error is on the Example.java page?
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User("Van", "Sanders");
ForumPost post = new ForumPost(author, "Success", "Huzzah! I did it!");
forum.addPost(post);
}
}

ISAIAH S
1,409 PointsNow I see what it means. "I expected to see the names I passed in through the args, but I do not. Hmm." Not the names that you passed in. So it wants '(args[0], args[1])
' in author
.
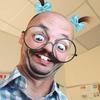
Van Sanders
16,087 PointsI totally see why that makes sense. However, it is still giving me the "java.lang.NullPointerException (Look around Forum.java line 13)" error. I can't figure out what about that line is not right.
This is my guess (though its obviously not working and must be wrong) - 'getFirstName()' is not a function within the brackets of 'getAuthor()', so it freaks out when we string them together as 'getAuthor().getFirstName().
However, when I break them down, that doesn't work either. Is there a way that I can remove 'getFirstName()' and feed 'args[1]' into 'getAuthor()' in the Forum.java code?

ISAIAH S
1,409 PointsNullPointerException
s aren't errors. they point out if there's a null. if you press preview, it will be blank, because there are no errors. In this case, it's around line 13.
Virinderpal Batth
1,793 PointsVirinderpal Batth
1,793 Pointsthe private fields in the user.java class should be declared outside of the constructor. Should look like this:
the "TODO" comment is telling you set your variables to the variables being passed in, not actually declaring them inside the constructor.