Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial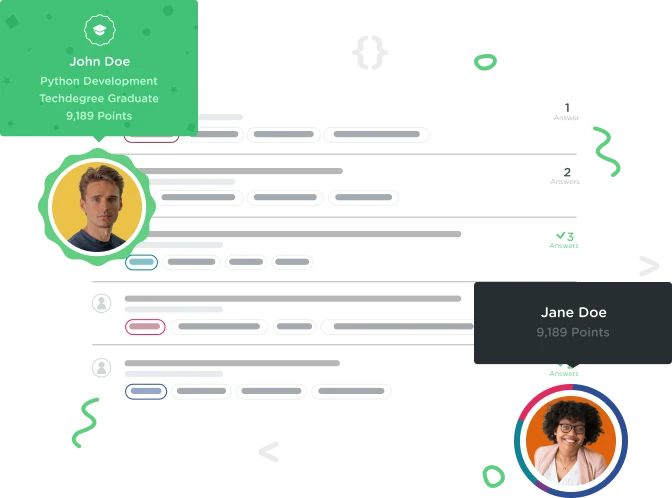

Bryce Hunter
1,186 Pointshow am i supposed to do this? Don't really get it.
can someone help me as to how I'm supposed to do this. Thanks!!!
import random
def random_member(list):
return len(list)
5 Answers
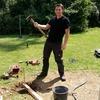
Nejc Vukovic
Full Stack JavaScript Techdegree Graduate 51,574 Pointsimport random
def random_member(list):
x = len(list) - 1
num = random.randint(0, x)
return list[num]

Bryan Laraway
13,366 PointsHi Bryce,
You want to avoid using "list" as a variable, as list is a keyword. I would recommend using something like "my_list" or "lst" instead. Are you having an issue with task 2 or task 3 for this challenge? Other than avoiding use of the list keyword, it looks fine for task 2.

rdaniels
27,258 PointsThis is how I did it. It passed the 3 challenges
import random
def random_member(my_list):
return len(my_list)
return random_member(len(my_list) - 1)
Hope this helps!!

Bryan Laraway
13,366 PointsTo pass the third challenge you need to alter what is being returned by the random_member function. Here's how I solved it:
import random
def random_member(my_list):
random_index = random.randint(0, (len(my_list)-1))
return my_list[random_index]
Note that I have added step where I pass the random number, between 0 and the length of the list -1, to the random_index variable. I then use this variable to return the item at that index from the my_list list.
Hope that helps!

Bryan Laraway
13,366 PointsNejc's answer above should complete Task 3 for you. The key is to remember that you want to get the item at the list index, which is why you return list[num] instead of just the random number returned from the prior step.
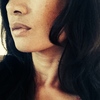
brightcricket
4,590 PointsI tried the code above and while it makes sense and should work, I am not able to pass the 3rd challenge. (Same code as Rodney's)
Bryce Hunter
1,186 PointsBryce Hunter
1,186 PointsIm on task 3 which is: Now, make random_member pick a random number between 0 and the length of the list, minus 1. So if the length was 5, get a number between 0 and 4. Return the list item with that index.