Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial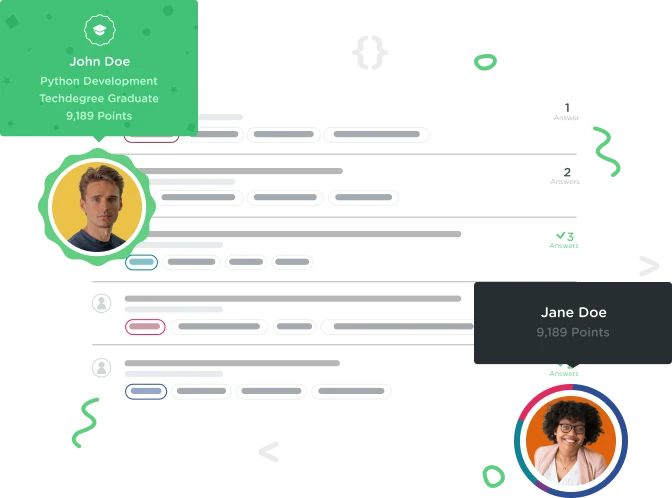

Otto linden
5,857 PointsHow are players and player connected?
Im wondering how player and players are connected? Because we take the names from players but how do they get to player? Via props I guess, but why does it change from
<App initialPlayers={players} />,
to
<Player
name={player.name}
score={player.score}
/> ?
The full code:
const players = [
{
name: "Guil",
score: 40
},
{
name: "Treasure",
score: 85
},
{
name: "Ashley",
score: 95
},
{
name: "James",
score: 80
}
];
const Header = (props) => {
return (
<header>
<h1>{props.title}</h1>
<span className="stats">Players: { props.totalPlayers }</span>
</header>
);
}
const Player = (props) => {
return (
<div className="player">
<span className="player-name">
{ props.name }
</span>
<Counter score={props.score}/>
</div>
);
}
const Counter = (props) => {
return (
<div className="counter">
<button className="counter-action decrement"> - </button>
<span className="counter-score">{ props.score }</span>
<button className="counter-action increment"> + </button>
</div>
);
}
const App = (props) => {
return (
<div className="scoreboard">
<Header
title="scoreboard"
totalPlayers={props.initialPlayers.length} />
{/* Players list */}
{props.initialPlayers.map( player =>
<Player
name={player.name}
score={player.score}
/>
)}
</div>
);
}
ReactDOM.render(
<App initialPlayers={players} />,
document.getElementById('root')
);
1 Answer

Michael Braga
Full Stack JavaScript Techdegree Graduate 24,174 PointsWe know that props.initialPlayers
is an array of players.
[{ name: "Guil", score: 40}, {name: "Treasure", score: 85}, {name: "Ashley", score: 95}, { name: "James", score: 80}];
JavaScript arrays have a function called map, that take a function and applies it or maps it to every item on that array. It then returns a new array with the mapped values. The mapping function for the video was this:
player => <Player name={player.name} score={player.score}/>;
So every object within the players array is mapped into a Players component that we declared previously. It takes the player objects's name and score properties and uses them as arguments for the Player component.
{ name: "Guil", score: 40} into this <Player name="Guil" score=40/>
The final result would look something like this :
<div className="scoreboard">
<Header
title="scoreboard"
totalPlayers={props.initialPlayers.length} />
{/* Players list */}
[
<Player name="Guil" score=40>,
<Player name="Treasure" score=85>,
<Player name="Ashley" score=95>,
<Player name="James" score=80>
]
)}
</div>
Kinda tricky but with some examples/practice you should be okay lol.
Antoine Venco
4,787 PointsAntoine Venco
4,787 PointsHi, I understood how the data was processed with the course but I can't figure out why each items of the array is renderred to the browser?
From what I understand the map array return a new array based on the data inside de players array. Since it is an array don't you need a function to render each of its items? I did some research on internet and I found some examples of arrays inside of {} in JSX returning their items but I can't found why!
I'm sure i'm missing something obvious :)
Ula Troc
1,881 PointsUla Troc
1,881 PointsTOOOP @Michael Braga THANK YOU ! YOU'RE THE BOSS