Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial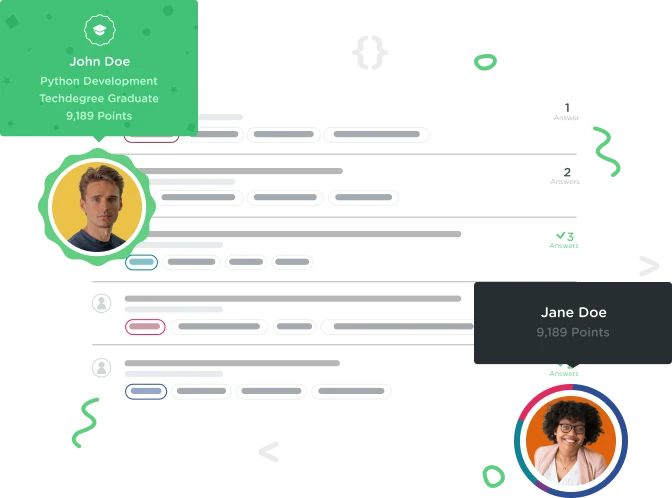
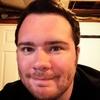
donald stouffer
7,630 PointsHow are we saving a string (hashTag) to Integer count with code: Integer count =hashTagCounts.get(hashTag);?
We are declaring a variable count type Integer. We are assigning the value of hashTagCounts.get(hashTag) to it. Doesn't hashTagCounts.get return a string? How is that being saved as an int?
4 Answers

Craig Dennis
Treehouse TeacherIf a Map doesn't contain the key and you call get
it will return null
. When you create a new Map
it has no keys.
Map<String, Integer> hashTagCounts = new HashMap<>();
Integer value = hashTagCounts.get("#jklol"); // This will be null, because jklol doesn't exist in the keys.
// A common pattern is to check if the return value is null, this means the key didn't exist.
if (value == null) {
value = 1;
// So therefore, we set it.
hashTagCounts.put("#jklol", value);
}
Now in that example the null check is pretty silly, because we know that the Map
is empty, because we just created it. But as we loop through values, the key might eventually exist, and therefore we want to increment the value.
That clear things up?

Timothy Morrison
7,720 PointsHey Donald. I had trouble with this too. I think what you are misunderstanding is that hashTagCounts.get(hashTag); is actually returning an Int.
hashTagCounts is our Map. It was declared in the code like this <String, Integer>. Which means The KEY is a STRING and the VALUE that it is "mapped" to is an INTEGER. So when we use the .get method we are giving it a STRING (i.e. hashTag) and expecting it to return the mapped/hashed INTEGER. We then store that INTEGER in Count.
I hope this was clear. It confused the heck out of me for a while. Very frustrating. Good Luck!

Jimmy Palelil
9,565 PointsBut the MAP-hashTagCounts is an empty map. how do we get info from it?

tomtrnka
9,780 PointsThanks, now it makes sence. I was wondering why do we call get() method over empty HashMap.
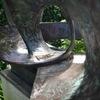
Stephen Shine
5,991 PointsI can't remember the full code and I didn't do the exercise in workspaces, I did it my own machine. I imagine it has something to do with the method hashTagCounts returning an int and performing a count function. The get method probably identifies all of the unique HashTags that have been made, and then the hashTagCount function may store the sum of the unique hashtags.

Isaias Valdez
1,412 PointsThe get() method in hashTagCounts.get(hashTag) does not return a String. The method returns the value(Integer) of the key(String) that is passed in.