Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial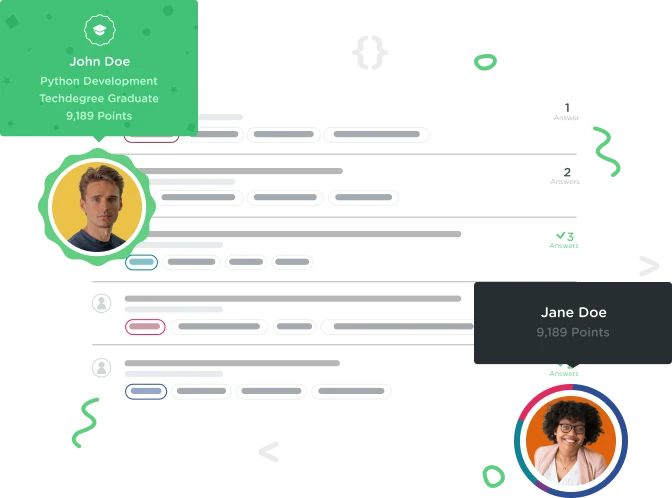

Jason Swoboda
Full Stack JavaScript Techdegree Student 1,525 PointsHow are you calling value() as a function inside randomRGB(value) if it's not a function?
Inside function randomRGB(value), you define the variable const color as a template literal that interpolates the argument value, but you add a () thus making it look like a function.
How does this work?
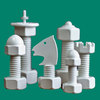
Steven Parker
231,268 PointsTony Shangkuan - always create a fresh question instead of asking one as a comment or an answer to another question, since the latter will generally only be seen by the original poster and answerer(s).
I see you did ask this as a separate question, you can see my answer there.
3 Answers
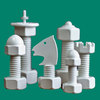
Steven Parker
231,268 PointsWhen randomRGB is called in the main program, the argument passed to it is randomValue, which is the name of a function. So for the duration of the randomRGB function, the parameter name "value" is equal to "randomValue", which means it can be called as a function.
When a function reference is passed as an argument to another function, this is often referred to as a "callback".

Charles Sok
Front End Web Development Techdegree Student 7,860 PointsHow is the argument randomValue being passed to it? I understand it is getting that value I just don't understand what is causing randomRGB to get the randomValue passed to it? to me it looks like it would be a broken loop of value trying to give the other three values a value of value. I've been on this video for about 5 hours now and it has me questioning if I can finish this course.
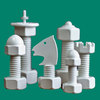
Steven Parker
231,268 PointsPerhaps this will help:
const randomValue = () => Math.floor(Math.random()*256);
function randomRGB(value) {
// ^^^^^
// This parameter name (value) will become
// a reference to the randomValue function.
const color = `rgb( ${value()}, ${value()}, ${value()} )`;
// ^^^^^^ ^^^^^^ ^^^^^^
// Each call to value() will actually be
// a call to randomValue(), and be
// replaced by a number.
return color;
}
for (let i=1; i<=10; i++) {
html += `<div style="background-color: ${randomRGB(randomValue)}">${i}</div>`;
// ^^^^^^^^^^^
// Here, randomValue is being passed as an argument.
// This passes a REFERENCE to the function but does not call it.
}

Charles Sok
Front End Web Development Techdegree Student 7,860 PointsSteven Parker So when we add the (randomValue) at the bottom it then passes it's argument to the randomRGB above it. why not add this argument to it to begin with instead of the (value)? is this something we learned before and I'm just not getting it?
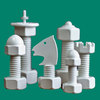
Steven Parker
231,268 PointsI'm not sure I understand the question. Please show me what the code would look like if you "add this argument to it to begin with".

Charles Sok
Front End Web Development Techdegree Student 7,860 PointsIt doesn't matter I can't figure this out.
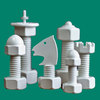
Steven Parker
231,268 PointsIf you show me what you are asking with code I can probably explain it. But the basic idea is that when you pass a function name as an argument, the function is not called right then. But when the other function it was passed to calls its parameter (by adding parentheses to the name), then it gets called.
Tony Shangkuan
7,200 PointsTony Shangkuan
7,200 PointsI do have the same question:
function randomRGB(value) { const color =
rgb (${value()}, ${value()}, ${value()},)
return color; }My question is there has not been a function value() created, and how does value() work in this kind of situation?