Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial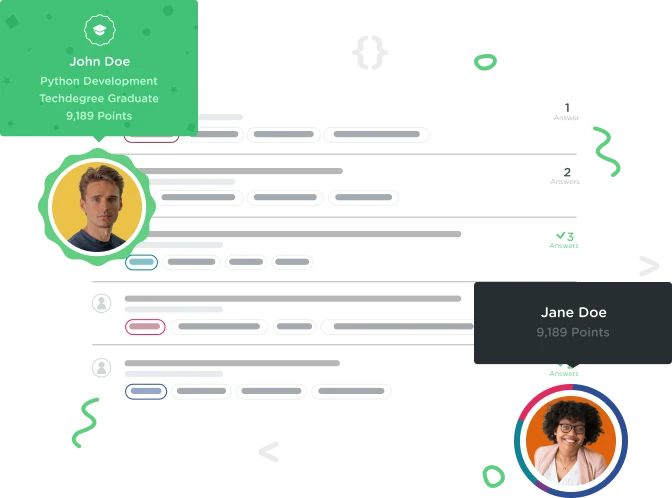
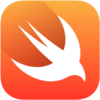
Antonija Pek
5,819 PointsHow bad did I miss the result?
// Enter your code below
func isOdd(num: number) -> Bool {
var odd = numbers.map({ $0 % 3 == 0 })
return odd
}
This is my try. What did I do wrong?
2 Answers
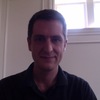
Max Hirsh
16,773 PointsWoah, I have been summoned! I haven't taken this course yet but I went ahead and took a look at the challenge. It looks like you are on the right track, but there are a couple things that are throwing off your code. The first is that "number" isn't a variable type that swift recognizes, so I would try using "Int". Next, the problem is asking you to write a function that will examine one number at time, not an array, so the first line of the function should be called on "num". The last point is that determining whether a number is odd or even is a matter of whether or not is divisible by 2. So that logic test should look at whether num % 2 is 1 or 0. Putting everything together to modify your function would look like this:
func isOdd(num: Int) -> Bool {
var odd = num % 2 == 1
return odd
}
Hope this helps!
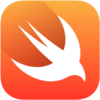
Antonija Pek
5,819 PointsHi, thanks for answering. I haven't had a chance to test this code, but I will.
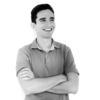
Alejandro Machado
16,091 PointsI'm late to the party, but Max is right! The function can also be written even more concisely like this:
func isOdd(num: Int) -> Bool {
return num % 2 == 1
}
That's right, you don't even need to store the "oddness" of the number in a variable if all you're going to do is return it.
Also, keep in mind that the map
function applies a given function to every element in an array and returns that array. Your isOdd
function is supposed to return a (single) Bool
, so that's why the compiler is giving you an error.