Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial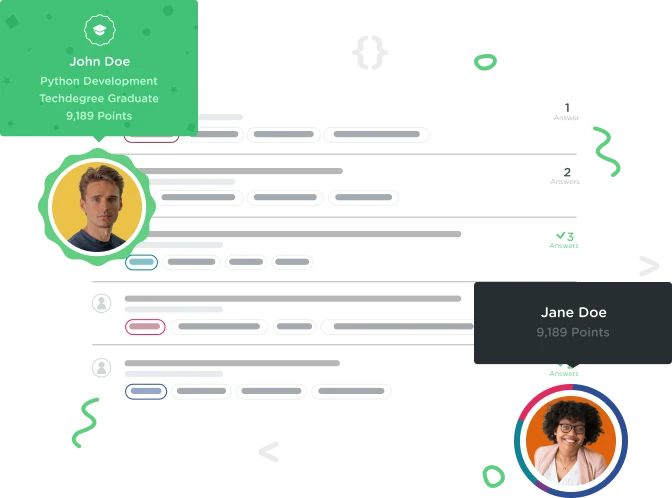

Tom Studer
8,098 PointsHow cam you assign a variable to a product SKU number that may start with a 0. example: SKU# 012- 6lb bag of sand.
If you have a product catalog and you want 012 to represent a bag of sand.
SKU# 012 - 6lb. bag of sand
How can you associate "012" with "6lb. bag of sand" without JS by default converting "012" to a different number type?
4 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Tom,
I don't know if this will meet your needs but one way you could do it is to store it as a property/value pair in an object.
var products = {"012" : "6lb. bag of sand"};
console.log(products["012"]); // outputs "6lb. bag of sand"

Tom Studer
8,098 PointsThank You!

Eric Butler
33,512 PointsFor what it's worth, Marcus Parsons's answer above (about storing SKUs as strings even if they're all-numeric) is considered the "best practice" in the real world, and you should do it that way.
Numbers are every bit as sortable/filterable/searchable when they're strings as they are when they're numbers, so there's no loss of use doing so, and then there's no problem with leading-zero-loss, rounding errors, etc. Plus if your company later switches to alphanumeric SKUs (which is not at all uncommon), you have no problems or extra work/headaches in doing so.
So please store SKUs as strings in your application.
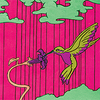
Christopher Bijalba
12,446 PointsAlso, if you needed the SKU as an int and not a string, you can use parseInt()
var sku ="0000000001234567890";
var sku_int = parseInt(sku);

Jason Anello
Courses Plus Student 94,610 PointsYou lose the leading zeroes if you do this.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsHere's some more code on how you might interact with this object:
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsJust to go into further detail about Jason's answer: unless you are using numbers for mathematical equations, you should store the number as a string, instead of a number type just as Jason did in his answer. Strings are safer for numbers that will not have any math applied to them because just as Jim shows in this video, Javascript has some wonky conversion factors and rounding errors for numbers because of the way it maps floating point numbers.
SKU numbers don't really need to have any kind of mathematical operations applied to them, so it is safe to store them as strings. If you were to, for example, have SKU numbers generated that were in numerical order, starting with 0, however, you could store them as strings, convert to integer number, apply mathematical operation, and then convert back to string. Or you can simply use a number type for a variable and start the SKU numbers with 1-9.