Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial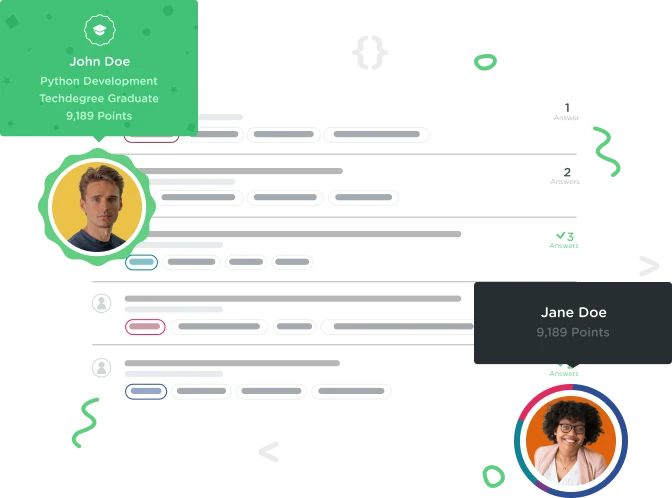

Shivam Barthwal
2,365 PointsHow can a boolean variable store a statement, as it is supposed to store only boolean values: either true/false, or 0/1;
public boolean applyGuess(Char letter) {
boolean isHit = answer.indexOf(letter) != -1;
if (isHit) {
hits += letter;
} else {
misses += letter;
}
return isHit;
}
Further, how come an if statement is checking for a variable name without any expression inside angular brackets. Like for instance from the above code: if (booleanvariable), Why?
5 Answers
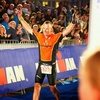
Steve Hunter
57,712 PointsHi Shivam,
The expression to the right of the equals sign evaluates to a true/false value. The first part of the expression answer.indexOf(letter)
returns either the index position of letter
within answer
or -1 if the letter isn't contained within the string. So, this is then compared using !=
to -1.
If the first part of the expression evaluates to, say 2, if the letter is at the third character of the answer string, then this comparison will evaluate to true
as the whole expression is 2 != -1
. This is what gets assigned into the boolean isHit
variable.
Secondly, the if
statement tests for a boolean condition. So, if(isHit)
is fine, as we have just established that isHit
will be either true or false. We could write it long-hand:
if(isHit == true)
But this adds nothing. The result of the condition is identical.
Make sense?
Steve.

Alex Ni
551 Pointswhat if isHit is false?
if(isHit){
hits += letter;
}
does this mean that if the condition is false then the wrong letter will be appended to hits?
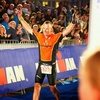
Steve Hunter
57,712 PointsHi Alex.
With the code you posted. isHit
is false then what is inside the curly braces never gets executed. That line of code only runs if isHit
evaluates to true
.
Does that help, or do you need more? shout if you do.
Steve.

Alex Ni
551 PointsHey Steve,
So if I write:
if (isHit == false){
misses += letter;
}
will this work?
Thanks.
[MOD: edited code block and added semicolon - srh]
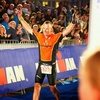
Steve Hunter
57,712 PointsTry it!
But, yes. You can do that. Or you can test for not true
:
if(!isHit){
misses += letter;
}
BUT this is a mutually exclusive scenario - isHit
can only have one of two values. So you can use the else
clause of your initial if
statement. If the first condition isn't met, i.e. you're no adding letter
to hits
, you must be adding letter
to misses
- there's no other scenario. So:
if(isHit){
hits += letter;
} else {
misses += letter
}
Make sense?
Steve.

Alex Ni
551 Pointsso..in these two cases:
if I write:
boolean isHit = false;
if (isHit){//statement}
this will not work.
but in this case:
boolean isHit = true;
if (!isHit){//statement}
this will work.
Why is that? Aren't these two cases in the same format--
if(false){//statement}--?
[MOD: edited code blocks - srh]
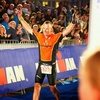
Steve Hunter
57,712 PointsHi Alex,
In short, no; neither of those will work.
Let's talk through my use of the not operator, !
, as that's what's caused this confusion.
An if
statement executes the statement
when the condition is true
. So:
if(condition == true){
// execute these statements
}
Your initial question was (I don't know if this formatting will work so an edit may be required!):
what if isHit is false?
if(isHit){ hits += letter; }does this mean that if the condition is false then the wrong letter will be appended to hits?
You wanted to know how to handle a false
answer. I suggested three ways. First, you came up with the idea of testing for isHit == false
- that's fine, yes. I then added that you could test for !isHit
- this is the same thing.
Going to your last two examples:
boolean isHit = false;
if (isHit){
// this won't execute as isHit isn't true
}
and:
boolean isHit = true;
if (!isHit){
// this won't execute either
}
Neither will execute the statements as, in both scenarios, the condition isn't true
. The statement only executes if the condition is true
.
You can test specifically to handle the false
scenario, negate the true
scenario or just add an else
clause to your if
conditional. These are the same in the management of the false
scenario:
if(isHit == false){
// executes when isHit is false
}
or:
if(!isHit){
// executes if isHit is false
}
and:
if(isHit){
// executes when isHit is true
} else {
// executes when isHit is false
}
Make sense? Let me know!
Steve.

Alex Ni
551 Pointso that makes so much sense. thank you Steve!
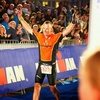
Steve Hunter
57,712 PointsNo problem!
Shivam Barthwal
2,365 PointsShivam Barthwal
2,365 PointsPerfect! Makes sense
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsShivam Barthwal
2,365 PointsShivam Barthwal
2,365 PointsHi Steve,
Do you know how to attach a link to workspace for code readability to others in our community as and when required?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsYep - click the "camera" icon in the top right of your workspace (it looks like a suitcase, to me!). And that'll get you a link to share, like: http://w.trhou.se/0o9djkculx
Other users can then 'fork' your code and use it in one of their workspaces.
omkar chendwankar
1,668 Pointsomkar chendwankar
1,668 Pointswhy it is supposed to return ishit;? can u explain?