Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial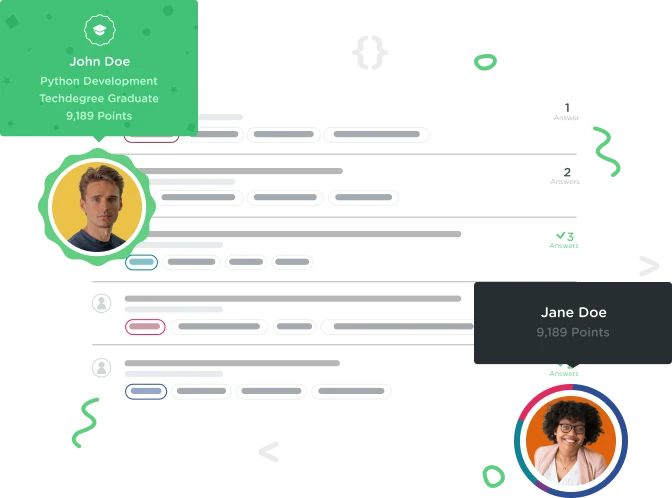

František Bakyta
1,452 PointsHow can I access the named input parameters "dividend" and "divisor" in the print out statement?
// isDivisible
func isDivisible(#dividend: Int, #divisor: Int) ->Bool? {
if dividend % divisor == 0 {
return true
} else {
return nil
}
}
if let result = isDivisible(dividend: 15, divisor: 4) {
println("\(dividend):\(divisor) is divisible.")
} else {
println("\(dividend):\(divisor) is not divisible.")
}
1 Answer

Stone Preston
42,016 PointsHow can I access the named input parameters "dividend" and "divisor" in the print out statement?
I dont think you can really accomplish that outside of the function body. if this println was occuring inside the function you could
you can assign them to constants before making the funtion call and pass the constants in as arugments:
func isDivisible(#dividend: Int, #divisor: Int) ->Bool? {
if dividend % divisor == 0 {
return true
} else {
return nil
}
}
let dividend = 15
let divisor = 4
if let result = isDivisible(dividend: dividend, divisor: divisor) {
println("\(dividend):\(divisor) is divisible.")
} else {
println("\(dividend):\(divisor) is not divisible.")
}
František Bakyta
1,452 PointsFrantišek Bakyta
1,452 PointsGreat, thank you Stone for your quick answer and help. If I don't assign them to constants before the function call, there is no way of accessing them in the code like I tried above in my answer? What is the purpose of the "named input parameter" for "external use" if I cannot use it in the code for example for printing out the inputs in the function?
Stone Preston
42,016 PointsStone Preston
42,016 Pointsthe external parameter name allows you to understand what the parameter actually is when you call the function since you have to provide the parameter name when calling a function with external parameters.
if you did not provide external parameters, the function call would look like this:
isDivisible(15, 4)
what is 15? what is 4? which one is the dividend? see the reason external parameters are helpful?
isDivisible(dividend: 15, divisor: 4)
in the above call, its clear what each argument is.
now, if you wanted to add the println inside the function you would have access the the parameters:
František Bakyta
1,452 PointsFrantišek Bakyta
1,452 PointsThank you very much Stone, now I fully understand what you tried to explain me :-)