Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial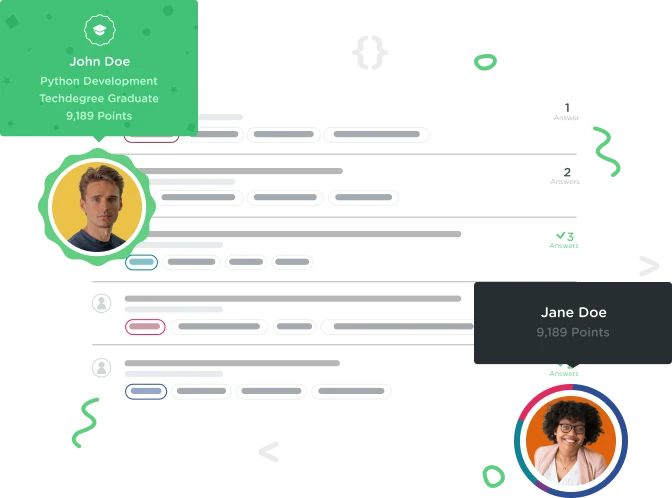
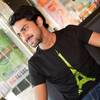
Aman Kumar
10,444 PointsHow can I access the static method "getMaker()"?
Sorry to ask question here about the last topic. Actually, i got it in the quiz
Right answer was static::getMaker()
But in one of my example when i tried the same syntax, it generated an error
Below is the code i tried
<?php
class Animal
{
public static $owner = 'Hayden';
public $name;
public $color;
public function __construct($name, $color)
{
$this->name = $name;
$this->color = $color;
}
public function getInfo()
{
return $this->name . $this->color;
}
public function getOwner()
{
return self::$owner;
}
public static function getOwnerName()
{
return self::$owner;
}
}
class Dog extends Animal
{
public $breed;
public function __construct($name, $color, $breed)
{
parent::__construct($name, $color);
$this->breed = $breed;
}
public function getInfo()
{
return 'Name: ' . $this->name . '<br/>Color: ' . $this->color . '<br/>Breed: ' . $this->breed;
}
}
?>
then i tried
echo static::getOwnerName();
and i got an error
Fatal error: Cannot access static:: when no class scope is active in /Applications/XAMPP/xamppfiles/htdocs/treehouse/oop.php on line 118
ignore the path specified
Can anyone please explain me about the the error in a bit detail. Thank You.
3 Answers

Chris McKirgan
5,666 PointsTo call a function statically, you simply need to call the static function using the format:
class::staticfunction();
Where 'class' is the name of your class ('Animal') and 'staticfunction' is your desired function ('Animal'). E.g.:
Animal::getOwnerName();
The key is understanding the syntax of what the format of the function definition is. Heres a breakdown using your function as an example
public static function getOwnerName()
Public
This is the scope of your function. This allows it to be access in other classes, or within your own. Options are public, private etc.
static
This allows your function to be called without instantiating your class as an object - you can just use Animal:: getOwnerName(); . Just note that this could mean you can call other functions, nor rely on the class's __constructor() method.
function
This tells PHP that the definition is a function.
getOwnerName
This is the name of your choice for the function.
()
These allow you to put in variable paramets to be accessed as scoped variables within your function. You could, for example do the following: Animal:: getOwnerName('harry');
// ^-- code
public static function getOwnerName($sName) {
echo $sName; // simply echos your parsed parameter.
}
// code --v
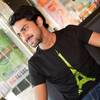
Aman Kumar
10,444 PointsI asked that, the correct answer to that question in the quiz was static::getInfo() but it is not working in my case. Why ?

Chris McKirgan
5,666 Points@amamkr if that is the case, the only possible reason for it is that there is another class called "static" of which you are calling the static function "getInfo". I have seen in quizes that they often fabricate new code to try and abstract the concepts from your own code.
It would be helpful to see the question and options in context - could you share them? I'm confused as you are at this point without it! :o)

Matthew Newman
8,741 PointsI think that answer to that question is either miss leading of just wrong. But below I compiled three ways to return the value of the $owner property which "Hayden".
Hope this helps.
I tested all three of these examples in workspaces and all work. ENJOY :)
echo Animal::$owner; //Calls the value of $owner property straight from the Animal class PROPERTY
echo Animal::getOwnerName(); //Calls the value of $owner property straight from the Animal class METHOD
$ownerObject = new Animal(); echo $ownerObject->getOwner(); //Calls the value of $owner property from an INSTANCE of the Animal class

Matthew Newman
8,741 PointsHere is a better formatted version:
<?php echo Animal::$owner . '<br>'; //Calls the value of $owner property straight from the Animal class PROPERTY
echo Animal::getOwnerName() . '<br>'; //Calls the value of $owner property straight from the Animal class METHOD
$ownerObject = new Animal(); echo $ownerObject->getOwner() . '<br>'; //Calls the value of $owner property from an INSTANCE of the Animal class ?>
Arbee Jacob Pagalilauan
7,483 PointsArbee Jacob Pagalilauan
7,483 PointsI was also confused on the same question. I tested both: "$this->getMaker();" and "static::getMaker();" and they seemed to work on the following code with no errors encountered on my end.
Code:
Output:
Please clarify. :)