Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial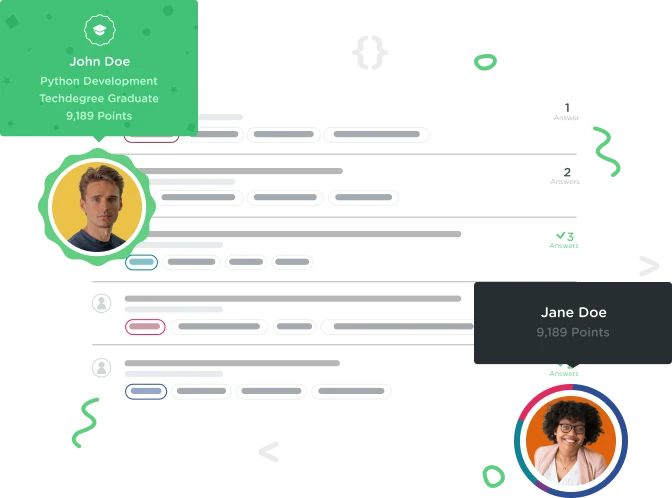

Amir Shahabnia
29,310 PointsHow can I add user's location to the request URL instead of hardcoding latitude and longitude?
I used locationManager function to get the user's latitude and longitude. I saved these values as Strings in a variable outside of the viewConroller's class. However when I try to use reach these variables' values in viewDidLoad(), the variables are empty. Below is my code:
import UIKit
import CoreLocation
var lat = ""
var long = ""
extension CurrentWeather {
var temperatureString: String {
return "\(Int(temperature))ΒΊ"
}
var humidityString: String {
let percentageValue = Int(humidity * 100)
return "\(percentageValue)%"
}
var precipitationProbabilityString: String {
let percentageValue = Int(precipitationProbability * 100)
return "\(percentageValue)%"
}
}
class ViewController: UIViewController, CLLocationManagerDelegate {
@IBOutlet weak var currentTemperatureLabel: UILabel!
@IBOutlet weak var currentHumidityLabel: UILabel!
@IBOutlet weak var currentPrecipitationLabel: UILabel!
@IBOutlet weak var currentWeatherIcon: UIImageView!
@IBOutlet weak var currentSummaryLabel: UILabel!
@IBOutlet weak var refreshButton: UIButton!
@IBOutlet weak var activityIndicator: UIActivityIndicatorView!
@IBOutlet weak var city: UILabel!
@IBOutlet weak var state: UILabel!
private let forecastAPI = "8a2bc5e53cc044aeefcfbabb13733561"
// loaction manager and lat and longitude vars
let locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
let icon = WeatherIcon.PartlyCloudyDay.image
let currentWeather = CurrentWeather(temperature: 56.0, humidity: 1.0, precipitationProbability: 1.0, summary: "Wet and rainy!", icon: icon)
display(currentWeather)
// instantiate loaction manager
locationManager.delegate = self;
locationManager.desiredAccuracy = kCLLocationAccuracyBest
locationManager.requestAlwaysAuthorization()
locationManager.startUpdatingLocation()
// creating baseURL and forecastURL
let baseURL = NSURL(string: "https://api.darksky.net/forecast/\(forecastAPI)/")
let forecastURL = NSURL(string: "\(lat),\(long)", relativeToURL: baseURL)
print(forecastURL?.description)
}
func locationManager(manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
let location: CLLocationCoordinate2D = locationManager.location!.coordinate
print("locations = \(location.latitude) \(location.longitude)")
lat = String(location.latitude)
long = String(location.longitude)
// get the street number, geocode
let geoCoder = CLGeocoder()
let locationAddress = CLLocation(latitude: location.latitude, longitude: location.longitude)
geoCoder.reverseGeocodeLocation(locationAddress) { (placemarks, error) in
// Place details
var placeMark: CLPlacemark!
placeMark = placemarks?[0]
// Address dictionary
//print(placeMark.addressDictionary)
// City
if let city = placeMark.addressDictionary!["City"] as? NSString {
self.city.text! = city as String
}
// Zip code
if let zip = placeMark.addressDictionary!["State"] as? NSString {
self.state.text! = zip as String
}
}
}
Amir Shahabnia
29,310 PointsAmir Shahabnia
29,310 PointsSo when I run:
let forecastURL = NSURL(string: "\(lat),\(long)", relativeToURL: baseURL)
it doesn't add user's latitude and longitude to the baseURL.