Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial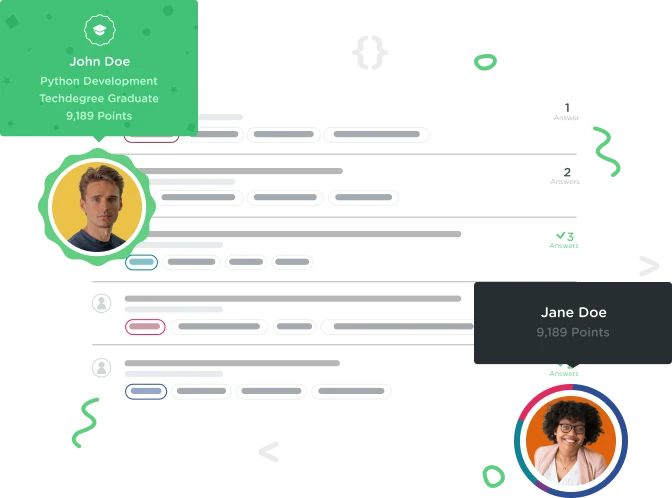

Steve Berrill
20,016 PointsHow can I also loop through the href for different social links in each list?
I made a variable $social_links as an array and then made a conditional in the foreach loop to echo the appropreite id of the the array in the href. I think this can be done allot cleaner though!
5 Answers
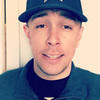
Vince Brown
16,249 PointsHey Steve, like Tom said its kind of hard to know what you're working with without the code but here is how I would personally think about possibly doing it. I too am a php beginner but figured it might help not only you but me as well lol.
Set array up so you can store more than just href and this way any other social media can be easily added later to the project
$social_links = array();
$social_links=['facebook"] = array(
"href" => "http://www.facebook.com",
"icon" => "path/to/image",
"name" => "facebook"
);
$social_links["twitter"] = array(
"href" => "http://www.twitter.com",
"icon" => "path/to/image",
"name" => "twitter"
);
and then you would want to construct a function to create the html to pass into the for each loop that way you keep your separation of concerns.
function get_social_media_links($social_link) {
$output = "";
$output = $output . '<li class =" ' . $social_link["name"] . ' "> ';
$output = $output . '<a href=" ' . $social_link["href"] . ' ">';
$output = $output . '<img src=" ' . $social_link["icon"] . ' "> ';
$output = $output . '</a>';
$output = $output . '</li>';
return $output;
}
and the foreach loop would look like this in your html
<ul class="social-media-links">
<?php
foreach($social_links as $social_link) {
echo get_social_media_links($social_link);
}
?>
</ul>
I didnt get a chance to test it but I think it should be good to go
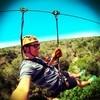
thomascawthorn
22,986 PointsI really like this. You can use even less code with this little number:
<?php
$output .= '</li>';
BUT. It's not very MVC. I am personally not a big fan of creating 'view' content in functions - it's not at all "wrong", but if you do it a lot, it can make for a messy and mixed controller/view. I see the related course to this post is PHP basics, so you may not be familiar with a MVC just yet.. If you work your way through any of the ruby on rails courses or laravel courses, these all use MVC frameworks. Essentially you split logic, decision making and visible output into 3 different sections called Model (logic), controller (decision maker) and view (visible output).
When you're creating a view like in this example, to me it seems much cleaner to write in html and open/close php tags where necessary, especially as there's a lot more html than php. I would do something like:
<?php
foreach($social_media_links as $social_media_link) {
include( inc/partials/social_media_icon.html.php );
}
?>
and the include file would be
<li class ="<?php $social_links["name"]; ?>">
<a href="<?php $social_links["href"]; ?>">
<img src="<?php $social_links["icon"]; ?>">
</a>
</li>
Thoughts?
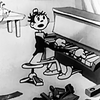
Andrew Rickards
18,877 PointsI know it's a bit late but here is my two cents. This is how I would have done it,
<?php
$social_links = array(
'facebook' => array( "href" => "http://www.facebook.com", "icon" => "facebook" ),
'twitter' => array( "href" => "http://www.twitter.com", "icon" => "twitter" ),
'google' => array( "href" => "http://plus.google.com", "icon" => "google" ),
'linkedinn' => array( "href" => "http://uk.linkedin.com", "icon" => "linkedinn" ),
);
?>
<ul class="social">
<?php foreach($social_links as $social_link) : ?>
<li>
<a href="<?php echo $social_link["href"]; ?>">
<span class="icon <?php echo $social_link["icon"]; ?>"></span>
</a>
</li>
<?php endforeach; ?>
</ul>

hl9
8,443 PointsThank you Andrew -- Your solution worked beautifully for my question here regarding attaching links to array items that are svg images (an svg sprite) instead of png's
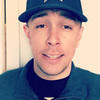
Vince Brown
16,249 PointsHaha glad it helped. I definitely learned some things as well, I am in the same boat as you right now with php as long as it works at first I can always refactor later. I feel like its one task to learn the concepts of a language and get comfortable with it and a whole other beast implementing it in a clean structured way.
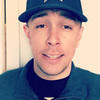
Vince Brown
16,249 PointsTom,
That code really makes me happy so clean and easy to sift through ! my code definitely is a rework of the php basics and I recently just did a site for a school project where I was templating a page but with no database experience I ended up using a huge array (like the one I wrote above) to store a whole bunch of information about different projects so I could later set them with a _GET variable and filter them in. I was able to keep my my main index file clean and just use the foreach loop to echo the function from my file that held the function in includes folder. But The whole $output strategy always seemed really messy and quite a tedious to write making sure all quotes dont interfere etc.. The first snippet you posted that you said "you could even lessen code with this number"
<?php
$output .= '</li>';
what is this exactly achieving , to me im processing that just has $ouput = the closing tag of an <li> is there any magic going on here lol?
sorry if I am kind of hijacking your question Steve!
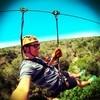
thomascawthorn
22,986 PointsNo worries!
It's a slightly nicer way to concatenate:
<?php
$output = $output . "Some string here";
// could be replaced by
$output .= "Some string here";
It's another way of saying $output equals itself plus "some string here".

Steve Berrill
20,016 PointsHi Tom / Vince,
thanks for the advice,
this is what I ended up with!
<?php
$social_links = array();
$social_links['facebook'] = array(
"href" => "http://www.facebook.com",
"icon" => "facebook",
);
$social_links['twitter'] = array(
"href" => "http://www.twitter.com",
"icon" => "twitter",
);
$social_links['google'] = array(
"href" => "http://plus.google.com",
"icon" => "google",
);
$social_links['linkedinn'] = array(
"href" => "http://uk.linkedin.com",
"icon" => "linkedinn",
);
function get_social_media_links($social_link) {
$output = "<li>";
$output = $output . '<a href="' . $social_link["href"] . '">';
$output = $output . '<span class="icon ' . $social_link["icon"] . '"> ';
$output = $output . '</span>';
$output = $output . '</a></li>';
return $output;
}
?>
<ul class="social">
<?php
foreach($social_links as $social_link) {
echo get_social_media_links($social_link);
} ?>
</ul>
I know its not very MVC as im just getting into this also, so maybe I will refactor another time lol
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsI think you might get more luck if you post your current code for people to play with ;)