Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial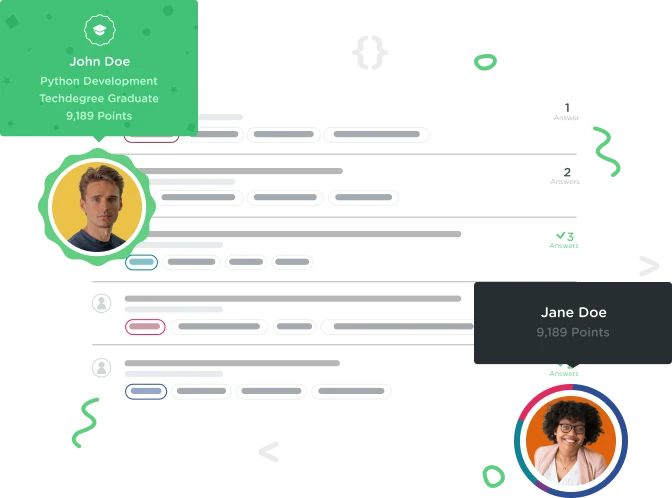

micahraaf
4,937 PointsHow can I call a function that returns multiple inputs with a function that takes multiple inputs without TypeError?
This may be a little advanced for this lesson. I took the input calls and combined them into a method called "get_input()" that returns multiple values like this:
def get_input():
total_due = float(input("What is the total: "))
amount_of_people = int(input("What is the amount of people: "))
return total_due,amount_of_people
I then called this function with split_check() that accepts two inputs, total and amount_of_people like this:
amount_due = split_check(get_input())
When I did this, I received a TypeError. The only way I have found around this is to capture the results of get_input() using this:
input_total, input_amount_of_people = get_input()
By then calling split_check(input_total,input_amount_of_people), the script runs correctly. Is there a way to call split_check(get_input()) without a TypeError? Would I have to return a tuple or another type from get_input() and accept this new type in split_check()?
3 Answers

Kevin Gates
15,053 PointsCan you update your question via the markdown cheatsheet? Specifically using the backticks and add the language you're using.
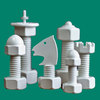
Steven Parker
230,274 PointsSince "split_check" requires two arguments, there's nothing you can do to "get_input" to make its output usable directly for input. But you could modify "split_check" to take a tuple, or create an intermediate conversion function:
def split_check_from_tuple(tup):
return split_check(tup[0], tup[1])
Then you should be able to: "amount_due = split_check_from_tuple(get_input())
".
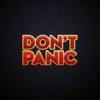
Hambone F
3,581 PointsActually, there is, see my other answer - in python you can send positional args as a tuple or list and unpack them with the *
operator, or even as a keyword dictionary and unpack that with the **
operator.
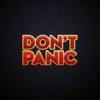
Hambone F
3,581 PointsActually - you're already returning a tuple:
return total_due,amount_of_people
And since you're already returning a tuple, all you need to do is unpack that tuple using the *
operator when you send it to split_check
:
amount_due = split_check(*get_input())