Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial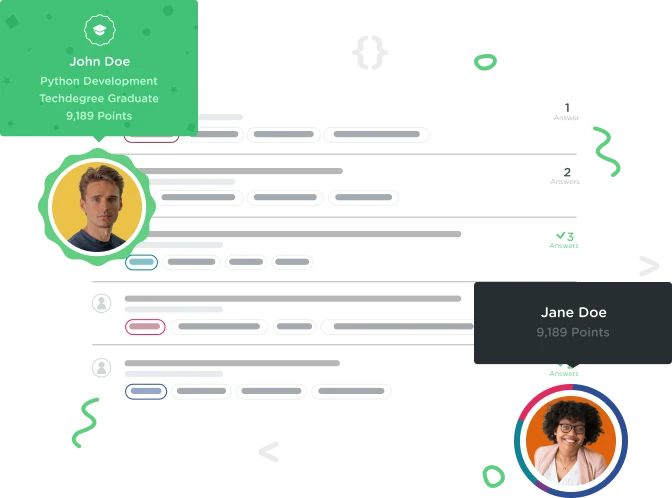

Vlatko .
2,526 PointsHow can I change text color for different Crystal Ball predictions?
Here's the code for my Crystal Ball's random predictions:
- (NSArray *) predictions {
if (_predictions == nil){
_predictions = @[@"It is certain.", @"It is decidedly so.", @"All signs say YES!", @"The stars are not aligned.", @"My reply is NO."];
}
return _predictions;
}
- (NSString *) randomPrediction {
int random = arc4random_uniform(self.predictions.count);
return [self.predictions objectAtIndex:random];
}
What I want to do is, create a new method that takes the index value returned above and throws it into an if else statement that will change the color based on the prediction chosen. For example, if either of the first two predictions are returned, index 0 or 1, the text color will be green, but if they aren't, it'll be red.
This is something I think it might look like, but honestly, I have no idea how to code this and it's not really something directly taught in the lessons:
- (NSString *) selectedColor {
if (randomPrediction = objectAtIndexes:0,1) {
return [UIColor greenColor];
} else {
return [UIColor redColor];
}
}
1 Answer

Stone Preston
42,016 Pointswhy not just make a randomColor method as well? just add a colors property to your class and a getter method for the property
@property (nonatomic) NSArray *colors;
- (NSArray *) colors {
if (_predictions == nil){
_predictions = @[[UIColor redColor], [UIColor greenColor], [UIColor blueColor]];
}
return _predictions;
}
then a method to randomize the colors
- (UIColor *) randomColor {
int random = arc4random_uniform(self.colors.count);
return [self.colors objectAtIndex:random];
}
you can do it the way you originally wanted as well. you would have to add a property to store the random prediction in the randomPrediction method, that way you can compare them in your selectedColors method
so add a property to store the prediction that was chosen randomly
@property (nonatomic) NSString *selectedPrediction;
and modify your randomPrediction method so that it stores the prediction in a property as well as returning it
- (NSString *) randomPrediction {
int random = arc4random_uniform(self.predictions.count);
self.selectedPrediction = [self.predictions objectAtIndex:random];
return self.selectedPrediction;
}
and then in your selectedColor method
- (UIColor *) selectedColor {
//if the selectedPrediction is the first or second one in the predictions array return green
if ([self.selectedPrediction isEqualToString:[self.predictions objectAtIndex:0]] || [self.selectedPrediction isEqualToString:[self.predictions objectAtIndex:0]]) {
return [UIColor greenColor];
} else {
return [UIColor redColor];
}
Vlatko .
2,526 PointsVlatko .
2,526 PointsGenius, it works!
I already had the randomColors down, but thought it would be neat to change colors to green/red for good/bad predictions. I can finally do that now with your code example. This will definitely come in handy for future projects. Thanks for the help.