Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial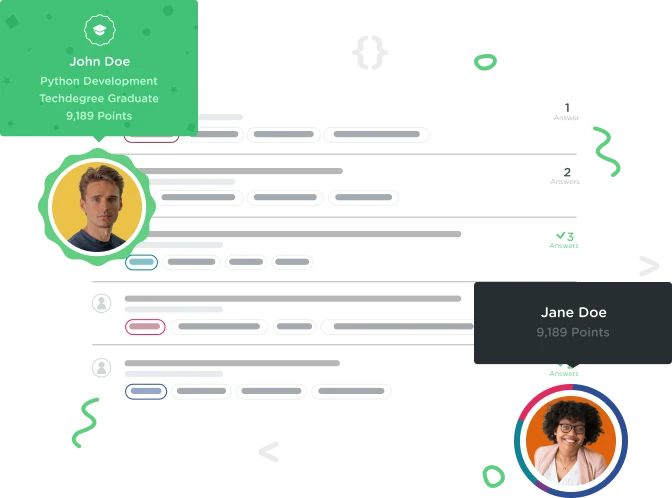
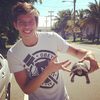
Dylan Hobbs
9,279 PointsHow can I change the url location when image is randomized to link to randomized image?
Hey Everyone,
Been playing with javascript and jquery lately and trying to figure this out but it has stumped me quite a bit.
I'm not sure if anyone here is able to help or figure it out but it's worth a shot!
Currently i've made a site that will randomize videos/images on click and on page load.
Once the page is loaded a video will randomize from the array and display.
If the user clicks the video it triggers it to randomize again.
What I am trying to figure out is how I can make the URL change as the new videos are randomized WITHOUT reloading the page.
So if a user randomizes to a funny video they like, they would be able to share the direct video with a friend just by copying the url link.
I have tried using something like history.js but find that the url's generated aren't consistent or linkable afterwards.
I am thinking I might have to use window.location.hash but can't figure it out.
Best answer and my undying respect for anyone who can help me solve this.
Here is a jsbin of the current project
http://jsbin.com/zemaje/1/edit?js,output
Note: I would like to figure this out in the situation if I had 1000's of images, just so I know how this would work.
Thanks in advance!
1 Answer

Geoff Parsons
11,679 PointsYou can't change the whole URL without reloading the page because it would make creating phishing sites too easy.
If the list of images is not going to change you could still use either the history API, history.js, or window.location.hash to achieve the same effect though. Simply put the index of the image or video into the hash:
$(document).ready(function() {
function loadImage(index){
if ( index < images.length ) {
var imageUrl = images[index];
$("#image img").prop('src', imageUrl);
window.location.hash = index;
} else {
loadRandomImage();
}
}
function loadRandomImage() {
var index = Math.floor(Math.random() * images.length)
loadImage(index)
}
if(window.location.hash) {
var hashIndex = parseInt(window.location.hash.replace(/[^\d]/,''))
loadImage(hashIndex)
} else {
loadRandomImage();
}
$('#image').on('click', loadRandomImage);
});
It's not the ideal solution but it will get the job done. This is similar to how a URL shortening site works though they will store the indexes (in most cases a Base64-encoded string instead of a direct array index) in a database along with the URL it represents.
Hopefully that's a better answer than my previous.
Dylan Hobbs
9,279 PointsDylan Hobbs
9,279 PointsHey Geoff,
Thanks for the response, but I have already figured out how to do this. The question I am trying to figure out is how I can change the URL LOCATION when an image is pulled from the array in the page.
.. I don't think you read the full post of my question or checked the jsfiddle that I provided, If you did read the full question I posted, I must not have written it clear enough so apologies in advance.
Thanks anyways
Geoff Parsons
11,679 PointsGeoff Parsons
11,679 PointsYou're right, sorry about that! Updated my answer above to hopefully be more along the lines of what you're looking for. Short-answer though is that you can't actually achieve what you're looking for; long answer is: you might be able to fake it.
Dylan Hobbs
9,279 PointsDylan Hobbs
9,279 PointsThanks geoff, no worries at all! I do have a few questions still. Will this allow users who visit the site to be able to link to the image that was randomized?
Wouldn't I be able to somehow use the array - for instance if it is the 23rd image in the array, then I can just call it by using the array number - [22] and somehow use location.hash to put it into the url so it would be something like 'mysite.com/image22' for instance?
I'm really not sure how to do this, i've tried reading documentation on it and I have checked with history.js as well as html5 pushstate but have found that it doesn't seem to be as reliable as it says and HTML5 pushstate doesn't have much for browser compatibility
I have seen sites achieve this without any server side, I really can't think of the name at the moment but there must be a way to append the array number to the end of the url of the image that is randomized. By faking it, will it still give the desired affect of linking to a specific image/video?
Would you by any chance be able to throw some code into the fiddle I've provided on how it could work?
Dylan Hobbs
9,279 PointsDylan Hobbs
9,279 PointsThanks Geoff, no worries at all! I do have some questions still. Would this method of 'faking' it still allow users who visit to link directly to an image that was randomized? For sharing purposes for instance?
Also, wouldn't I be able to use the image number in the array and append it to the url? For instance if it is the 23rd image in the array would I be able to pull it as [22] and somehow use locaton.hash to add it to the url?
I'm still learning javascript but I have seen other sites achieve this and i'm pretty sure they aren't using anything server sided. Not sure if it's using the 'faking' it method though.
I have been scratching my head over this for a while and have looked into the documentation for html5 pushstates as well as history.js but from what i've read they aren't very reliable and html5 pushstate doesn't have the browser compatibility on older versions..
I don't know, i'm pretty confused haha. Do you think you would be able to add into the jsbin I provided even just a little bit so I can see how I would achieve this?
Geoff Parsons
11,679 PointsGeoff Parsons
11,679 PointsCheck out the update to the answer above for some example code. Unfortunately it doesn't look like jsfiddle allows you to mess with the window location at all from within the script; at least not that i can see. So you'll need to try running these locally i think.
Dylan Hobbs
9,279 PointsDylan Hobbs
9,279 PointsOkay will do! Will the code you've provided above or this 'faking'it method allow for the sharing functionality that I am after?
Geoff Parsons
11,679 PointsGeoff Parsons
11,679 PointsIt should. It will append the array index to the url to look something like:
http://yoursite.example.com/images.html#123
Each time they click the image it will load a new one and update the URL hash. If a user copies the URL and pastes it the script will look for that and try to load that specific image:
The only issue would be if you change the order of images in your array then old URLs will be invalid as they may load different images/videos.
Dylan Hobbs
9,279 PointsDylan Hobbs
9,279 PointsHey Geoff! Sorry to bug you again. I've been playing around with the code you've provided and i've finally got it to load in as mysite.com/#2 or /#3 etc.. depending on which video is loaded in but does not allow linking to that link afterwards.
Just a few questions: Does the # symbol mean that it is generated within the HTML and is just temporary? Is there anyway to remove this, or am I not entering the code in properly?
Here is an updated jsbin. http://jsbin.com/vesebu/1/edit?html,js,output
Thanks again