Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial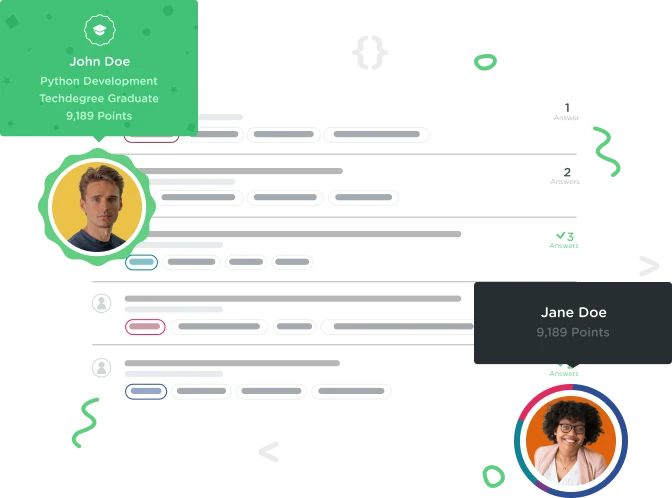

Nurbek Ismailov
2,068 Pointshow can i combine this code together to print one message in html
I was writing a quiz app, and i can't figure out how i can print sentence: "You got (4 or whatever #) questions right, and You Earned a (Gold or other) Medal" as one sentence using the code below. correctGuess is counter variable. thanks
function print (message) {
var printHTML= document.getElementById('outcome');
printHTML.innerHTML=message;
}
var results= 'You got ' + correctGuess + ' question(s) right';
print (results);
if (correctGuess >= 4) {
print("<p><strong> and You Earned a Gold Medal</strong></p>");
}
else if (correctGuess >= 3) {
print("<p><strong>and You Earned a Silver Medal</strong></p>");
}
else if (correctGuess >= 2) {
print("<p><strong>and You Earned a Bronze Medal</strong></p>");
}
else {
print('<p><strong>and You Earned No Medal</strong></p>');
}
3 Answers

Aaron Martone
3,290 Points/*
* Let's be DRY (Don't Repeat Yourself). "Code Smells" are repeating patterns
* in script that indicate that a form of automation should be able to
* remove repeat statements and consolidate into less code.
*/
// First thing, let's name our functions semantically. 'print' doesn't tell us
// much, but 'displayResults' is a bit easier to determine its function.
// Note we don't need to pass anything into the function, as the only variable
// it needs access to (correctGuess) is in its parent scope, so it has access
// to this anyways.
function displayResults() {
// This variable wll hold the message we build programmatically and display
// to the user.
var message = '';
// This is how all messages start...
message += '<p>You got ' + correctGuess + ' question';
// This says 'If correctGuess is not 1, we turn "question" to "questions"
// for the sake of proper grammar.'...
message += (correctGuess !== 1) ? 's' : '';
// Continue on building the message...
message += ' right <strong>and You Earned ';
// Here we determine the medal type using nested ternary operators. The
// ternary is (expression that equates to true/false) ? true result : false
// result. And notice as with abovee we are '+=' the 'message' variable.
// This keeps adding the strings together to make a whole message.
message += (correctGuess >= 4) ? 'A Gold'
: (correctGuess === 3) ? 'A Silver'
: (correctGuess === 2) ? 'A Bronze'
: 'No';
// Close off the remaining of the message.
message += ' Medal.</strong></p>';
// Since we know the target and are not using it anywhere else, there's no
// need to make a variable to hold a reference to it. We simply target it
// and send the message to its '.innerHTML' in one step.
document.getElementById('outcome').innerHTML = message;
}
// Change to any number.
var correctGuess = 5;
// Call the function to get the ball rolling. No arguments needed.
displayResults();```

Nurbek Ismailov
2,068 PointsAaron yours is definitely DRY, but a lot of new concept for me. i don't understand the logic of
message += (correctGuess !== 1) ? 's' : '';
message +=
(correctGuess >= 4) ? 'A Gold'
: (correctGuess === 3) ? 'A Silver'
: (correctGuess === 2) ? 'A Bronze'
: 'No';
what does : , ? " " mean
like in line message += (correctGuess !== 1) ? 's' : ' ';

Aaron Martone
3,290 PointsFirst, there is string concatenation. It's the process of taking 1 string and adding on additional content. I use the +=
operator to do this, so a += b
is the same as saying a = a + b
.
var a = 'Hello';
a += ' World'; // now 'a' is equal to 'Hello World'.
The second part is what's known as a ternary operator
. The syntax is expression ? value1 : value2
. The way it works is that the expression
is evaluated. If it's true, value1
is used, otherwise if it's false, value2
is used.
var choice = 'grapes'
var fruit = (choice === 'bananas') ? 'You chose bananas!'
: (choice === 'apples') ? 'You chose apples!'
: (choice === 'grapes') ? 'You chose grapes!'
: 'Is that even fruit?';
How the above works from the top down is: JS determines if your choice (grapes) is equal to bananas. Since it isn't, the first value (You chose bananas) is not used, but it goes to the value after the : (which in this case is another ternary that checks if your choice (grapes) is equal to apples, which again, it is not, so it continues in this pattern to the next ternary, which is true, so the value 'You chose grapes' is stored into the var fruit.
This pattern is a concise way to do multiple if/else.

Cindy Lea
Courses Plus Student 6,497 PointsHeres a similar quiz & its code:
// 5 questions var answer1 = prompt("In Toy Story, what is the name of the super cool pizza place?"); // Pizza Planet var answer2 = prompt("Who is the dude in green in The Legend of Zelda?"); // Link var answer3 = prompt("What is GLaDOS' favourite vegetable?"); // Potatoes var answer4 = prompt("Who is Luke Skywalker's father?"); // Darth Vader var answer5 = prompt("Who says, 'YOU! SHALL NOT! PASS!'"); // Gandalf
var numberCorrect = 0;
// Question 1 if (answer1.toUpperCase() === "PIZZA PLANET") { numberCorrect += 1; } else{}
// Question 2 if (answer2.toUpperCase() === "LINK") { numberCorrect += 1; } else{}
// Question 3 if (answer3.toUpperCase() === "POTATOES") { numberCorrect += 1; } else{}
//Question 4 if (answer4.toUpperCase() === "DARTH VADER") { numberCorrect += 1; } else{}
//Question 5 if (answer5.toUpperCase() === "GANDALF") { numberCorrect += 1; } else{}
// Awards if ( numberCorrect === 5) { document.write("Congratulations! You got " + numberCorrect + " correct out of 5 and earned a Gold Medal!"); } else if ( numberCorrect === 3 || numberCorrect==4) { document.write("Congratulations! You got " + numberCorrect + " correct out of 5 and earned a Silver Medal!"); } else if ( numberCorrect === 1 || numberCorrect === 2) { document.write("Congratulations! You got " + numberCorrect + " correct out of 5 and earned a Bronze Medal!"); } else if ( numberCorrect === 0) { document.write("Sorry, but you shall not pass. :(");
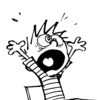
stevenstabile
9,763 PointsHi Nurbek,
You are calling the print function twice and overwriting the first call with the second.
You could do something like this: (I assume you've defined correctGuess somewhere but I'll add it here just for the example)
function print (message) {
var printHTML= document.getElementById('outcome');
printHTML.innerHTML=message;
}
var correctGuess = 5;
var results= '<p>You got ' + correctGuess + ' question(s) right';
if (correctGuess >= 4) {
results += "<strong> and You Earned a Gold Medal</strong></p>";
print(results);
}
else if (correctGuess >= 3) {
results += "<strong> and You Earned a Silver Medal</strong></p>";
print(results);
}
else if (correctGuess >= 2) {
results += "<strong> and You Earned a Bronze Medal</strong></p>";
print(results);
}
else {
results += "<strong> and You Earned No Medal</strong></p>";
print(results);
}
Aaron Martone
3,290 PointsAaron Martone
3,290 PointsI don't see 'correctGuess' defined anywhere, but I'll assume that's been calculated. an example would be: if (correctGuess >= 4) { print('<p><strong>You got ' + correctGuess + ' questions right, and you Earned a Gold Medal.</strong></p>');