Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial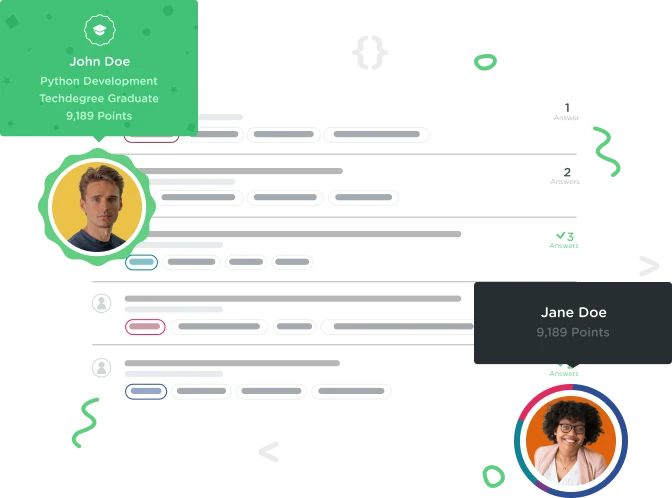

Sarvesh Tandon
93 PointsHow can I convert String into int and int into string in C++ and Java??
b = a + 0; I tried this but it did not work.
2 Answers
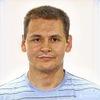
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsIn Java you should use valueOf
method for both conversions:
https://docs.oracle.com/javase/7/docs/api/java/lang/Integer.html#valueOf(java.lang.String)
https://docs.oracle.com/javase/7/docs/api/java/lang/String.html#valueOf(int)
So to convert int
to String
you write:
int number = 1;
String stringFromNumber = String.valueOf(number);
// shorthand will be
String stringFromNumber = number + "";
To convert int
to String
is harder, because you have to account for NumberFormatException
, see Docs links above:
String numberString = "1";
int intFromString;
try {
intFromString = Integer.valueOf(numberString);
} catch (NumberFormatException nfe) {
// do something
System.out.println(numberString + " is not a number");
}
For C++ I don't know, so you have to google yourself...
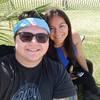
Henry Phillips
501 PointsFor C++, you would use a function called itoa and stoi. These stand for integer to http://www.cplusplus.com/reference/cstdlib/itoa/ http://www.cplusplus.com/reference/string/stoi/
To convert from an int to a string, you would type out:
int a = 10; char *intStr = itoa(a); string str = string(intStr);
To convert from string to an int you would use stoi: http://www.cplusplus.com/reference/string/stoi/
string str1 = "45"; int myint1 = stoi(str1);
Sorry for the late response, but hey, maybe it'll help someone else down the line.
Sarvesh Tandon
93 PointsSarvesh Tandon
93 PointsIn this 'b' is a int and 'a' is a string.