Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial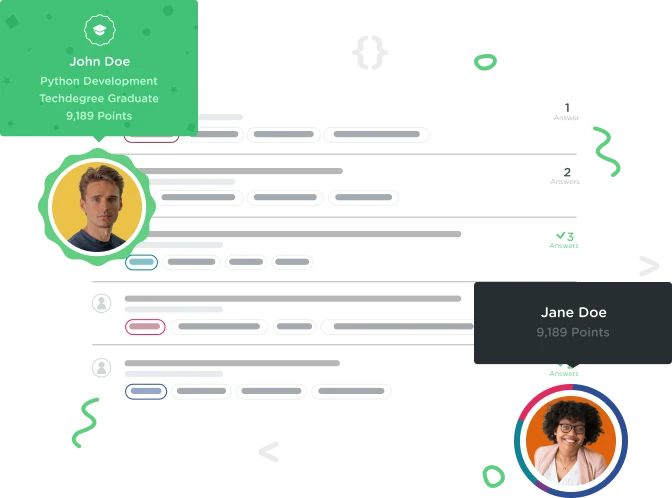

Jess Sanders
12,086 PointsHow can I deploy my Hangman Java game using IntelliJ IDEA?
I would like to share my Hangman game made in the Java course on my personal web site. Can someone point me towards a tutorial that can guide me through the process?
I'm looking for something like this, but for IntelliJ IDEA: http://www.oxfordmathcenter.com/drupal7/node/37
4 Answers

Seth Kroger
56,413 PointsSo, I tried putting that into applet form and succeeded. Since you already have a Swing GUI to make it into an applet you need to extend the class from JApplet instead of JFrame then change main() to init().
The real issue is that Oracle Java's security policy forces anyone viewing the applet through a browser to manually add an exception to allow it to run. Unless your willing to shell out $$$$ for a signing certificate.

Seth Kroger
56,413 PointsThe only parts really specific to an IDE is adding the acm.jar file to the project creating your .jar file and the instructions from the IDEA docs are here https://www.jetbrains.com/idea/help/configuring-module-dependencies-and-libraries.html#d1265574e216 and here https://www.jetbrains.com/idea/help/packaging-a-module-into-a-jar-file.html

Jess Sanders
12,086 PointsI'm sharing my hangman game here, because I haven't yet figured out how to put it on the web.
drawHangman.java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
/**
* Created by jess on 4/17/15.
*/
public class drawHangman extends JFrame {
public boolean head = false;
public boolean body = false;
public boolean leftArm = false;
public boolean rightArm = false;
public boolean hips = false;
public boolean leftLeg = false;
public boolean rightLeg = false;
public JPanel p1;
public JPanel p2;
public JButton btnA = new JButton("a");
public JButton btnB = new JButton("b");
public JButton btnC = new JButton("c");
public JButton btnD = new JButton("d");
public JButton btnE = new JButton("e");
public JButton btnF = new JButton("f");
public JButton btnG = new JButton("g");
public JButton btnH = new JButton("h");
public JButton btnI = new JButton("i");
public JButton btnJ = new JButton("j");
public JButton btnK = new JButton("k");
public JButton btnL = new JButton("l");
public JButton btnM = new JButton("m");
public JButton btnN = new JButton("n");
public JButton btnO = new JButton("o");
public JButton btnP = new JButton("p");
public JButton btnQ = new JButton("q");
public JButton btnR = new JButton("r");
public JButton btnS = new JButton("s");
public JButton btnT = new JButton("t");
public JButton btnU = new JButton("u");
public JButton btnV = new JButton("v");
public JButton btnW = new JButton("w");
public JButton btnX = new JButton("x");
public JButton btnY = new JButton("y");
public JButton btnZ = new JButton("z");
private Game mGame;
private DrawCanvas canvas;
private Label currentWord;
public drawHangman() {
Game game = new Game("beehive");
mGame = game;
canvas = new DrawCanvas();
canvas.setPreferredSize(new Dimension(1100, 500));
this.setContentPane(canvas);
setUpComponents();
this.pack();
this.setVisible(true);
}
public void setUpComponents() {
p1 = new JPanel();
p2 = new JPanel();
JPanel p3 = new JPanel();
p1.setLayout(new FlowLayout());
p2.setLayout(new FlowLayout());
p3.setLayout(new FlowLayout());
p1.setBackground(Color.RED);
p2.setBackground(Color.RED);
p3.setBackground(Color.WHITE);
p1.add(btnA);
p1.add(btnB);
p1.add(btnC);
p1.add(btnD);
p1.add(btnE);
p1.add(btnF);
p1.add(btnG);
p1.add(btnH);
p1.add(btnI);
p1.add(btnJ);
p1.add(btnK);
p1.add(btnL);
p1.add(btnM);
p2.add(btnN);
p2.add(btnO);
p2.add(btnP);
p2.add(btnQ);
p2.add(btnR);
p2.add(btnS);
p2.add(btnT);
p2.add(btnU);
p2.add(btnV);
p2.add(btnW);
p2.add(btnX);
p2.add(btnY);
p2.add(btnZ);
btnA.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnA);
checkLetter('a');
}
});
btnB.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnB);
checkLetter('b');
}
});
btnC.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnC);
checkLetter('c');
}
});
btnD.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnD);
checkLetter('d');
}
});
btnE.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnE);
checkLetter('e');
}
});
btnF.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnF);
checkLetter('f');
}
});
btnG.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnG);
checkLetter('g');
}
});
btnH.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnH);
checkLetter('h');
}
});
btnI.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnI);
checkLetter('i');
}
});
btnJ.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnJ);
checkLetter('j');
}
});
btnK.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnK);
checkLetter('k');
}
});
btnL.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnL);
checkLetter('l');
}
});
btnM.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p1.remove(btnM);
checkLetter('m');
}
});
btnN.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnN);
checkLetter('n');
}
});
btnO.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnO);
checkLetter('o');
}
});
btnP.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnP);
checkLetter('p');
}
});
btnQ.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnQ);
checkLetter('q');
}
});
btnR.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnR);
checkLetter('r');
}
});
btnS.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnS);
checkLetter('s');
}
});
btnT.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnT);
checkLetter('t');
}
});
btnU.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnU);
checkLetter('u');
}
});
btnV.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnV);
checkLetter('v');
}
});
btnW.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnW);
checkLetter('w');
}
});
btnX.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnX);
checkLetter('x');
}
});
btnY.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnY);
checkLetter('y');
}
});
btnZ.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
p2.remove(btnZ);
checkLetter('z');
}
});
getContentPane().setLayout(new FlowLayout());
getContentPane().setBackground(Color.white);
getContentPane().add(p1);
getContentPane().add(p2);
currentWord = new Label("- - - - - - - - - -");
p3.add(currentWord);
getContentPane().add(p3);
}
public void setUpActions() {
}
private void checkLetter (char ltr) {
if (mGame.validateGuess(ltr)) {
if (mGame.applyGuess(ltr)) {
currentWord.setText(mGame.getCurrentProgress());
} else {
int wrongGuesses = mGame.mMisses.length();
if (wrongGuesses == 1) {
head = true;
} else if (wrongGuesses == 2) {
body = true;
} else if (wrongGuesses == 3) {
leftArm = true;
} else if (wrongGuesses == 4) {
rightArm = true;
} else if (wrongGuesses == 5) {
hips = true;
} else if (wrongGuesses == 6) {
leftLeg = true;
} else if (wrongGuesses == 7) {
rightLeg = true;
}
}
}
canvas.repaint();
requestFocus(); //change the focus to JFrame
}
private class DrawCanvas extends JPanel {
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
setBackground(Color.WHITE);
g.fill3DRect(48, 48+50, 4, 300, true);
g.fill3DRect(48, 48+50, 48, 4, true);
g.fill3DRect(96, 48+50, 4, 48, true);
g.fill3DRect(24, 348+50, 48, 4, true);
if (head) {
g.fillOval(24 + 48, 48 + 48 + 50, 48, 48); //draw head
}
if (body) {
g.fill3DRect(96, 48 + 48 + 50 + 48, 4, 48, true); //draw body
}
if (leftArm) {
g.fill3DRect(96-12,48+48+50+48+18,12,4,true);
}
if (rightArm) {
g.fill3DRect(96,48+48+50+48+18,12+4,4,true);
}
if (hips) {
g.fill3DRect(96-10,48+48+50+48+48,12+10,4,true);
}
if (leftLeg){
g.fill3DRect(96-10,48+48+50+48+48,4,24,true);
}
if (rightLeg){
g.fill3DRect(96+6+4,48+48+50+48+48,4,24,true);
getContentPane().remove(p1);
getContentPane().remove(p2);
}
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new drawHangman();
}
});
}
}

Seth Kroger
56,413 PointsWould you mind if I had a few suggestions? That button code isn't particularly DRY. Usually if you have a lot of buttons or other widgets it's better to have one listener and have the listener find out which button was pressed. An event listener like this is usually passed the "who, what, when", or at least the "who".
private ActionListener mLetterListener = new ActionListener() {
public void actionPerformed(ActionEvent e) {
JButton buttonClicked = (JButton) e.getSource();
char letter = buttonClicked.getText().charAt(0);
buttonClicked.getParent().remove(buttonClicked);
checkLetter(letter);
}
};
Also since you have 26 individual buttons that are each 1. added to a panel, 2. given a listener, you can replace all that with a for loop.
public static final String ALPHABET = "abcdefghijklmnopqrstuvwxyz";
// ...
int length = ALPHABET.length();
for (int i = 0; i < length; i++) {
JButton button = new JButton(ALPHABET.substring(i,i+1));
button.addActionListener(mLetterListener);
if(i < length/2) { // add the first half of the alphabet to panel 1
p1.add(button);
} else { // and the second half to panel 2
p2.add(button);
}
}

Jess Sanders
12,086 PointsThis was going to be my next step. Thanks!

Jess Sanders
12,086 PointsGame.java
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
public String mHits;
public String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
public boolean validateGuess(char letter) {
boolean isANewGuess = false;
if (Character.isLetter(letter)) {
isANewGuess = true;
}
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0){
isANewGuess = false;
}
return isANewGuess;
}
public boolean applyGuess(char letter) {
boolean isAHit = mAnswer.indexOf(letter) >= 0;
if (isAHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isAHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
progress += " ";
}
return progress;
}
public String getRemainingTries() {
return String.valueOf(MAX_MISSES - mMisses.length());
}
}