Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial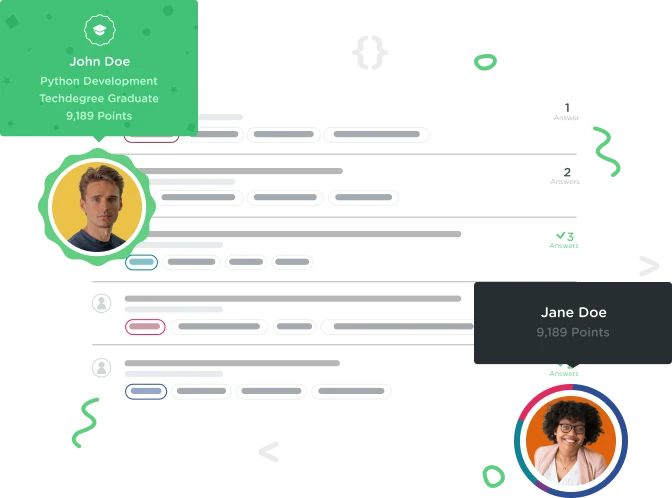

Dan Smith
2,942 PointsHow can i determine which part of the array i want to multiply and how do i send it to a new var?
const numbers = [1,2,3,4,5,6,7,8,9,10]; let times10 = [];
// times10 should be: [10,20,30,40,50,60,70,80,90,100] // Write your code below
//Your Code Goes Here: numbers.forEach(function(multiply) { multiply [i] = ""; return times10 = (multiply[i] *= 10); });
I CANNOT FIGURE HOW CAN I TELL THE JS THAT I WANT TO MULTIPLE THE CONST NUMBERS.
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times10 = [];
// times10 should be: [10,20,30,40,50,60,70,80,90,100]
// Write your code below
numbers.forEach(function(multiply) {
multiply [i] = "";
return times10 = (multiply[i] *= 10);
});
3 Answers
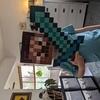
Gabbie Metheny
33,778 PointsWith .forEach()
, you don't need to worry about a counter variable, it's build into the method! So there's no need for an index of [i]
in your code. I'd also recommend changing your multiply
parameter to number
, simply because it will hopefully clarify what is happening in your code - you're iterating over each number in numbers
.
Right now, you're trying to return the number you've multiplied, but you want to actually add it to the array. You can use the .push()
method for this.
numbers.forEach(function(number) {
// multiply the number by 10
// and push it onto the times10 array
});
Let me know if you need further clarification!

Dan Smith
2,942 Pointsso it basically knows i want to use all the items inside the array? what if i have a chain of arrays?
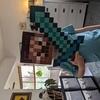
Gabbie Metheny
33,778 PointsYes, it's basically a shortcut if you want to do something to each item in an array.
const array = [1, 2, 3, 4];
for (let i=0; i<array.length; i+=1 ) {
console.log(array[i])
}
// output:
// 1
// 2
// 3
// 4
const array = [1, 2, 3, 4];
array.forEach(function(number) {
console.log(number);
});
// output:
// 1
// 2
// 3
// 4
The above two loops will produce the same output.
As far as a chain of arrays, can you give me an example of what you mean?

Thomas Nilsen
14,957 PointsYou could use the map-function for this:
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times10 = [];
// times10 should be: [10,20,30,40,50,60,70,80,90,100]
// Write your code below
times10 = numbers.map(n => n * 10)
console.log(times10);
Edit
Sorry - I didn't see this was a forEach challenge. I'll just leave the answer here if you're interested